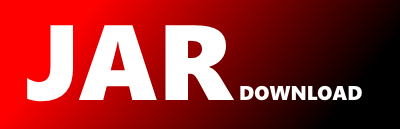
com.powsybl.computation.mpi.generated.Messages Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: messages.proto
package com.powsybl.computation.mpi.generated;
public final class Messages {
private Messages() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface CommonFileOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// required int32 chunk = 2;
/**
* required int32 chunk = 2;
*/
boolean hasChunk();
/**
* required int32 chunk = 2;
*/
int getChunk();
// required bool last = 3;
/**
* required bool last = 3;
*/
boolean hasLast();
/**
* required bool last = 3;
*/
boolean getLast();
// required bytes data = 4;
/**
* required bytes data = 4;
*/
boolean hasData();
/**
* required bytes data = 4;
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code messages.CommonFile}
*/
public static final class CommonFile extends
com.google.protobuf.GeneratedMessage
implements CommonFileOrBuilder {
// Use CommonFile.newBuilder() to construct.
private CommonFile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private CommonFile(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final CommonFile defaultInstance;
public static CommonFile getDefaultInstance() {
return defaultInstance;
}
public CommonFile getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommonFile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
chunk_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
last_ = input.readBool();
break;
}
case 34: {
bitField0_ |= 0x00000008;
data_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_CommonFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_CommonFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.CommonFile.class, Messages.CommonFile.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CommonFile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommonFile(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required int32 chunk = 2;
public static final int CHUNK_FIELD_NUMBER = 2;
private int chunk_;
/**
* required int32 chunk = 2;
*/
public boolean hasChunk() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 chunk = 2;
*/
public int getChunk() {
return chunk_;
}
// required bool last = 3;
public static final int LAST_FIELD_NUMBER = 3;
private boolean last_;
/**
* required bool last = 3;
*/
public boolean hasLast() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bool last = 3;
*/
public boolean getLast() {
return last_;
}
// required bytes data = 4;
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_;
/**
* required bytes data = 4;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
private void initFields() {
name_ = "";
chunk_ = 0;
last_ = false;
data_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasChunk()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLast()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasData()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, chunk_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(3, last_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, data_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, chunk_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, last_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.CommonFile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.CommonFile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.CommonFile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.CommonFile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.CommonFile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.CommonFile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.CommonFile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.CommonFile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.CommonFile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.CommonFile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.CommonFile prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.CommonFile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.CommonFileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_CommonFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_CommonFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.CommonFile.class, Messages.CommonFile.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.CommonFile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
chunk_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
last_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_CommonFile_descriptor;
}
public Messages.CommonFile getDefaultInstanceForType() {
return Messages.CommonFile.getDefaultInstance();
}
public Messages.CommonFile build() {
Messages.CommonFile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.CommonFile buildPartial() {
Messages.CommonFile result = new Messages.CommonFile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.chunk_ = chunk_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.last_ = last_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.data_ = data_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.CommonFile) {
return mergeFrom((Messages.CommonFile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.CommonFile other) {
if (other == Messages.CommonFile.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasChunk()) {
setChunk(other.getChunk());
}
if (other.hasLast()) {
setLast(other.getLast());
}
if (other.hasData()) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasChunk()) {
return false;
}
if (!hasLast()) {
return false;
}
if (!hasData()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.CommonFile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.CommonFile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// required int32 chunk = 2;
private int chunk_ ;
/**
* required int32 chunk = 2;
*/
public boolean hasChunk() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 chunk = 2;
*/
public int getChunk() {
return chunk_;
}
/**
* required int32 chunk = 2;
*/
public Builder setChunk(int value) {
bitField0_ |= 0x00000002;
chunk_ = value;
onChanged();
return this;
}
/**
* required int32 chunk = 2;
*/
public Builder clearChunk() {
bitField0_ = (bitField0_ & ~0x00000002);
chunk_ = 0;
onChanged();
return this;
}
// required bool last = 3;
private boolean last_ ;
/**
* required bool last = 3;
*/
public boolean hasLast() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bool last = 3;
*/
public boolean getLast() {
return last_;
}
/**
* required bool last = 3;
*/
public Builder setLast(boolean value) {
bitField0_ |= 0x00000004;
last_ = value;
onChanged();
return this;
}
/**
* required bool last = 3;
*/
public Builder clearLast() {
bitField0_ = (bitField0_ & ~0x00000004);
last_ = false;
onChanged();
return this;
}
// required bytes data = 4;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes data = 4;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* required bytes data = 4;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
data_ = value;
onChanged();
return this;
}
/**
* required bytes data = 4;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000008);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:messages.CommonFile)
}
static {
defaultInstance = new CommonFile(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.CommonFile)
}
public interface TaskOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 jobId = 1;
/**
* required int32 jobId = 1;
*/
boolean hasJobId();
/**
* required int32 jobId = 1;
*/
int getJobId();
// required int32 index = 2;
/**
* required int32 index = 2;
*/
boolean hasIndex();
/**
* required int32 index = 2;
*/
int getIndex();
// required .messages.Task.Environment env = 3;
/**
* required .messages.Task.Environment env = 3;
*/
boolean hasEnv();
/**
* required .messages.Task.Environment env = 3;
*/
Messages.Task.Environment getEnv();
/**
* required .messages.Task.Environment env = 3;
*/
Messages.Task.EnvironmentOrBuilder getEnvOrBuilder();
// required string cmdId = 4;
/**
* required string cmdId = 4;
*/
boolean hasCmdId();
/**
* required string cmdId = 4;
*/
java.lang.String getCmdId();
/**
* required string cmdId = 4;
*/
com.google.protobuf.ByteString
getCmdIdBytes();
// repeated .messages.Task.Command command = 5;
/**
* repeated .messages.Task.Command command = 5;
*/
java.util.List
getCommandList();
/**
* repeated .messages.Task.Command command = 5;
*/
Messages.Task.Command getCommand(int index);
/**
* repeated .messages.Task.Command command = 5;
*/
int getCommandCount();
/**
* repeated .messages.Task.Command command = 5;
*/
java.util.List extends Messages.Task.CommandOrBuilder>
getCommandOrBuilderList();
/**
* repeated .messages.Task.Command command = 5;
*/
Messages.Task.CommandOrBuilder getCommandOrBuilder(
int index);
// repeated .messages.Task.InputFile inputFile = 6;
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
java.util.List
getInputFileList();
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
Messages.Task.InputFile getInputFile(int index);
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
int getInputFileCount();
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
java.util.List extends Messages.Task.InputFileOrBuilder>
getInputFileOrBuilderList();
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
Messages.Task.InputFileOrBuilder getInputFileOrBuilder(
int index);
// repeated .messages.Task.OutputFile outputFile = 7;
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
java.util.List
getOutputFileList();
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
Messages.Task.OutputFile getOutputFile(int index);
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
int getOutputFileCount();
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
java.util.List extends Messages.Task.OutputFileOrBuilder>
getOutputFileOrBuilderList();
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
Messages.Task.OutputFileOrBuilder getOutputFileOrBuilder(
int index);
// required bool initJob = 8;
/**
* required bool initJob = 8;
*/
boolean hasInitJob();
/**
* required bool initJob = 8;
*/
boolean getInitJob();
// repeated int32 completedJobId = 9;
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
java.util.List getCompletedJobIdList();
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
int getCompletedJobIdCount();
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
int getCompletedJobId(int index);
}
/**
* Protobuf type {@code messages.Task}
*/
public static final class Task extends
com.google.protobuf.GeneratedMessage
implements TaskOrBuilder {
// Use Task.newBuilder() to construct.
private Task(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Task(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Task defaultInstance;
public static Task getDefaultInstance() {
return defaultInstance;
}
public Task getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Task(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
jobId_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
index_ = input.readInt32();
break;
}
case 26: {
Messages.Task.Environment.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = env_.toBuilder();
}
env_ = input.readMessage(Messages.Task.Environment.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(env_);
env_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
bitField0_ |= 0x00000008;
cmdId_ = input.readBytes();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
command_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
command_.add(input.readMessage(Messages.Task.Command.PARSER, extensionRegistry));
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
inputFile_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
inputFile_.add(input.readMessage(Messages.Task.InputFile.PARSER, extensionRegistry));
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
outputFile_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
outputFile_.add(input.readMessage(Messages.Task.OutputFile.PARSER, extensionRegistry));
break;
}
case 64: {
bitField0_ |= 0x00000010;
initJob_ = input.readBool();
break;
}
case 72: {
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
completedJobId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
completedJobId_.add(input.readInt32());
break;
}
case 74: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100) && input.getBytesUntilLimit() > 0) {
completedJobId_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
while (input.getBytesUntilLimit() > 0) {
completedJobId_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
command_ = java.util.Collections.unmodifiableList(command_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
inputFile_ = java.util.Collections.unmodifiableList(inputFile_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
outputFile_ = java.util.Collections.unmodifiableList(outputFile_);
}
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
completedJobId_ = java.util.Collections.unmodifiableList(completedJobId_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.class, Messages.Task.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Task parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Task(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public interface InputFileOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// required .messages.Task.InputFile.Scope scope = 2;
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
boolean hasScope();
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
Messages.Task.InputFile.Scope getScope();
// optional bytes data = 3;
/**
* optional bytes data = 3;
*/
boolean hasData();
/**
* optional bytes data = 3;
*/
com.google.protobuf.ByteString getData();
// required .messages.Task.InputFile.PreProcessor preProcessor = 4;
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
boolean hasPreProcessor();
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
Messages.Task.InputFile.PreProcessor getPreProcessor();
}
/**
* Protobuf type {@code messages.Task.InputFile}
*/
public static final class InputFile extends
com.google.protobuf.GeneratedMessage
implements InputFileOrBuilder {
// Use InputFile.newBuilder() to construct.
private InputFile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private InputFile(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final InputFile defaultInstance;
public static InputFile getDefaultInstance() {
return defaultInstance;
}
public InputFile getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InputFile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
Messages.Task.InputFile.Scope value = Messages.Task.InputFile.Scope.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
scope_ = value;
}
break;
}
case 26: {
bitField0_ |= 0x00000004;
data_ = input.readBytes();
break;
}
case 32: {
int rawValue = input.readEnum();
Messages.Task.InputFile.PreProcessor value = Messages.Task.InputFile.PreProcessor.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
bitField0_ |= 0x00000008;
preProcessor_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_InputFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_InputFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.InputFile.class, Messages.Task.InputFile.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public InputFile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InputFile(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code messages.Task.InputFile.PreProcessor}
*/
public enum PreProcessor
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NONE = 1;
*/
NONE(0, 1),
/**
* ARCHIVE_UNZIP = 2;
*/
ARCHIVE_UNZIP(1, 2),
/**
* FILE_GUNZIP = 3;
*/
FILE_GUNZIP(2, 3),
;
/**
* NONE = 1;
*/
public static final int NONE_VALUE = 1;
/**
* ARCHIVE_UNZIP = 2;
*/
public static final int ARCHIVE_UNZIP_VALUE = 2;
/**
* FILE_GUNZIP = 3;
*/
public static final int FILE_GUNZIP_VALUE = 3;
public final int getNumber() { return value; }
public static PreProcessor valueOf(int value) {
switch (value) {
case 1: return NONE;
case 2: return ARCHIVE_UNZIP;
case 3: return FILE_GUNZIP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PreProcessor findValueByNumber(int number) {
return PreProcessor.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return Messages.Task.InputFile.getDescriptor().getEnumTypes().get(0);
}
private static final PreProcessor[] VALUES = values();
public static PreProcessor valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private PreProcessor(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:messages.Task.InputFile.PreProcessor)
}
/**
* Protobuf enum {@code messages.Task.InputFile.Scope}
*/
public enum Scope
implements com.google.protobuf.ProtocolMessageEnum {
/**
* RUN = 1;
*/
RUN(0, 1),
/**
* JOB = 2;
*/
JOB(1, 2),
/**
* TASK = 3;
*/
TASK(2, 3),
;
/**
* RUN = 1;
*/
public static final int RUN_VALUE = 1;
/**
* JOB = 2;
*/
public static final int JOB_VALUE = 2;
/**
* TASK = 3;
*/
public static final int TASK_VALUE = 3;
public final int getNumber() { return value; }
public static Scope valueOf(int value) {
switch (value) {
case 1: return RUN;
case 2: return JOB;
case 3: return TASK;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Scope findValueByNumber(int number) {
return Scope.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return Messages.Task.InputFile.getDescriptor().getEnumTypes().get(1);
}
private static final Scope[] VALUES = values();
public static Scope valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Scope(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:messages.Task.InputFile.Scope)
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .messages.Task.InputFile.Scope scope = 2;
public static final int SCOPE_FIELD_NUMBER = 2;
private Messages.Task.InputFile.Scope scope_;
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
public boolean hasScope() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
public Messages.Task.InputFile.Scope getScope() {
return scope_;
}
// optional bytes data = 3;
public static final int DATA_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString data_;
/**
* optional bytes data = 3;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes data = 3;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
// required .messages.Task.InputFile.PreProcessor preProcessor = 4;
public static final int PREPROCESSOR_FIELD_NUMBER = 4;
private Messages.Task.InputFile.PreProcessor preProcessor_;
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
public boolean hasPreProcessor() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
public Messages.Task.InputFile.PreProcessor getPreProcessor() {
return preProcessor_;
}
private void initFields() {
name_ = "";
scope_ = Messages.Task.InputFile.Scope.RUN;
data_ = com.google.protobuf.ByteString.EMPTY;
preProcessor_ = Messages.Task.InputFile.PreProcessor.NONE;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasScope()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPreProcessor()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, scope_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, data_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeEnum(4, preProcessor_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, scope_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, data_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, preProcessor_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.Task.InputFile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.InputFile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.InputFile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.InputFile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.InputFile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.InputFile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.Task.InputFile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.Task.InputFile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.Task.InputFile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.InputFile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.Task.InputFile prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.Task.InputFile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.Task.InputFileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_InputFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_InputFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.InputFile.class, Messages.Task.InputFile.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.Task.InputFile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
scope_ = Messages.Task.InputFile.Scope.RUN;
bitField0_ = (bitField0_ & ~0x00000002);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
preProcessor_ = Messages.Task.InputFile.PreProcessor.NONE;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_Task_InputFile_descriptor;
}
public Messages.Task.InputFile getDefaultInstanceForType() {
return Messages.Task.InputFile.getDefaultInstance();
}
public Messages.Task.InputFile build() {
Messages.Task.InputFile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.Task.InputFile buildPartial() {
Messages.Task.InputFile result = new Messages.Task.InputFile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.scope_ = scope_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.data_ = data_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.preProcessor_ = preProcessor_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.Task.InputFile) {
return mergeFrom((Messages.Task.InputFile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.Task.InputFile other) {
if (other == Messages.Task.InputFile.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasScope()) {
setScope(other.getScope());
}
if (other.hasData()) {
setData(other.getData());
}
if (other.hasPreProcessor()) {
setPreProcessor(other.getPreProcessor());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasScope()) {
return false;
}
if (!hasPreProcessor()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.Task.InputFile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.Task.InputFile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// required .messages.Task.InputFile.Scope scope = 2;
private Messages.Task.InputFile.Scope scope_ = Messages.Task.InputFile.Scope.RUN;
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
public boolean hasScope() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
public Messages.Task.InputFile.Scope getScope() {
return scope_;
}
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
public Builder setScope(Messages.Task.InputFile.Scope value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
scope_ = value;
onChanged();
return this;
}
/**
* required .messages.Task.InputFile.Scope scope = 2;
*/
public Builder clearScope() {
bitField0_ = (bitField0_ & ~0x00000002);
scope_ = Messages.Task.InputFile.Scope.RUN;
onChanged();
return this;
}
// optional bytes data = 3;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes data = 3;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes data = 3;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* optional bytes data = 3;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
data_ = value;
onChanged();
return this;
}
/**
* optional bytes data = 3;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000004);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// required .messages.Task.InputFile.PreProcessor preProcessor = 4;
private Messages.Task.InputFile.PreProcessor preProcessor_ = Messages.Task.InputFile.PreProcessor.NONE;
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
public boolean hasPreProcessor() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
public Messages.Task.InputFile.PreProcessor getPreProcessor() {
return preProcessor_;
}
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
public Builder setPreProcessor(Messages.Task.InputFile.PreProcessor value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
preProcessor_ = value;
onChanged();
return this;
}
/**
* required .messages.Task.InputFile.PreProcessor preProcessor = 4;
*/
public Builder clearPreProcessor() {
bitField0_ = (bitField0_ & ~0x00000008);
preProcessor_ = Messages.Task.InputFile.PreProcessor.NONE;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:messages.Task.InputFile)
}
static {
defaultInstance = new InputFile(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.Task.InputFile)
}
public interface OutputFileOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
boolean hasPostProcessor();
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
Messages.Task.OutputFile.PostProcessor getPostProcessor();
}
/**
* Protobuf type {@code messages.Task.OutputFile}
*/
public static final class OutputFile extends
com.google.protobuf.GeneratedMessage
implements OutputFileOrBuilder {
// Use OutputFile.newBuilder() to construct.
private OutputFile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private OutputFile(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final OutputFile defaultInstance;
public static OutputFile getDefaultInstance() {
return defaultInstance;
}
public OutputFile getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OutputFile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
Messages.Task.OutputFile.PostProcessor value = Messages.Task.OutputFile.PostProcessor.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
postProcessor_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_OutputFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_OutputFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.OutputFile.class, Messages.Task.OutputFile.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public OutputFile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OutputFile(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code messages.Task.OutputFile.PostProcessor}
*/
public enum PostProcessor
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NONE = 1;
*/
NONE(0, 1),
/**
* FILE_GZIP = 2;
*/
FILE_GZIP(1, 2),
;
/**
* NONE = 1;
*/
public static final int NONE_VALUE = 1;
/**
* FILE_GZIP = 2;
*/
public static final int FILE_GZIP_VALUE = 2;
public final int getNumber() { return value; }
public static PostProcessor valueOf(int value) {
switch (value) {
case 1: return NONE;
case 2: return FILE_GZIP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PostProcessor findValueByNumber(int number) {
return PostProcessor.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return Messages.Task.OutputFile.getDescriptor().getEnumTypes().get(0);
}
private static final PostProcessor[] VALUES = values();
public static PostProcessor valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private PostProcessor(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:messages.Task.OutputFile.PostProcessor)
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
public static final int POSTPROCESSOR_FIELD_NUMBER = 2;
private Messages.Task.OutputFile.PostProcessor postProcessor_;
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
public boolean hasPostProcessor() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
public Messages.Task.OutputFile.PostProcessor getPostProcessor() {
return postProcessor_;
}
private void initFields() {
name_ = "";
postProcessor_ = Messages.Task.OutputFile.PostProcessor.NONE;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPostProcessor()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, postProcessor_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, postProcessor_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.Task.OutputFile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.OutputFile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.OutputFile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.OutputFile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.OutputFile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.OutputFile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.Task.OutputFile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.Task.OutputFile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.Task.OutputFile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.OutputFile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.Task.OutputFile prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.Task.OutputFile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.Task.OutputFileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_OutputFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_OutputFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.OutputFile.class, Messages.Task.OutputFile.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.Task.OutputFile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
postProcessor_ = Messages.Task.OutputFile.PostProcessor.NONE;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_Task_OutputFile_descriptor;
}
public Messages.Task.OutputFile getDefaultInstanceForType() {
return Messages.Task.OutputFile.getDefaultInstance();
}
public Messages.Task.OutputFile build() {
Messages.Task.OutputFile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.Task.OutputFile buildPartial() {
Messages.Task.OutputFile result = new Messages.Task.OutputFile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.postProcessor_ = postProcessor_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.Task.OutputFile) {
return mergeFrom((Messages.Task.OutputFile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.Task.OutputFile other) {
if (other == Messages.Task.OutputFile.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasPostProcessor()) {
setPostProcessor(other.getPostProcessor());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasPostProcessor()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.Task.OutputFile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.Task.OutputFile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
private Messages.Task.OutputFile.PostProcessor postProcessor_ = Messages.Task.OutputFile.PostProcessor.NONE;
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
public boolean hasPostProcessor() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
public Messages.Task.OutputFile.PostProcessor getPostProcessor() {
return postProcessor_;
}
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
public Builder setPostProcessor(Messages.Task.OutputFile.PostProcessor value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
postProcessor_ = value;
onChanged();
return this;
}
/**
* required .messages.Task.OutputFile.PostProcessor postProcessor = 2;
*/
public Builder clearPostProcessor() {
bitField0_ = (bitField0_ & ~0x00000002);
postProcessor_ = Messages.Task.OutputFile.PostProcessor.NONE;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:messages.Task.OutputFile)
}
static {
defaultInstance = new OutputFile(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.Task.OutputFile)
}
public interface VariableOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// required string value = 2;
/**
* required string value = 2;
*/
boolean hasValue();
/**
* required string value = 2;
*/
java.lang.String getValue();
/**
* required string value = 2;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code messages.Task.Variable}
*/
public static final class Variable extends
com.google.protobuf.GeneratedMessage
implements VariableOrBuilder {
// Use Variable.newBuilder() to construct.
private Variable(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Variable(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Variable defaultInstance;
public static Variable getDefaultInstance() {
return defaultInstance;
}
public Variable getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Variable(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_Variable_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_Variable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.Variable.class, Messages.Task.Variable.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Variable parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Variable(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private java.lang.Object value_;
/**
* required string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
name_ = "";
value_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.Task.Variable parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.Variable parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.Variable parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.Variable parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.Variable parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.Variable parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.Task.Variable parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.Task.Variable parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.Task.Variable parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.Variable parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.Task.Variable prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.Task.Variable}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.Task.VariableOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_Variable_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_Variable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.Variable.class, Messages.Task.Variable.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.Task.Variable.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_Task_Variable_descriptor;
}
public Messages.Task.Variable getDefaultInstanceForType() {
return Messages.Task.Variable.getDefaultInstance();
}
public Messages.Task.Variable build() {
Messages.Task.Variable result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.Task.Variable buildPartial() {
Messages.Task.Variable result = new Messages.Task.Variable(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.Task.Variable) {
return mergeFrom((Messages.Task.Variable)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.Task.Variable other) {
if (other == Messages.Task.Variable.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasValue()) {
bitField0_ |= 0x00000002;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.Task.Variable parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.Task.Variable) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// required string value = 2;
private java.lang.Object value_ = "";
/**
* required string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 2;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
/**
* required string value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* required string value = 2;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:messages.Task.Variable)
}
static {
defaultInstance = new Variable(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.Task.Variable)
}
public interface EnvironmentOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// repeated .messages.Task.Variable variable = 1;
/**
* repeated .messages.Task.Variable variable = 1;
*/
java.util.List
getVariableList();
/**
* repeated .messages.Task.Variable variable = 1;
*/
Messages.Task.Variable getVariable(int index);
/**
* repeated .messages.Task.Variable variable = 1;
*/
int getVariableCount();
/**
* repeated .messages.Task.Variable variable = 1;
*/
java.util.List extends Messages.Task.VariableOrBuilder>
getVariableOrBuilderList();
/**
* repeated .messages.Task.Variable variable = 1;
*/
Messages.Task.VariableOrBuilder getVariableOrBuilder(
int index);
}
/**
* Protobuf type {@code messages.Task.Environment}
*/
public static final class Environment extends
com.google.protobuf.GeneratedMessage
implements EnvironmentOrBuilder {
// Use Environment.newBuilder() to construct.
private Environment(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Environment(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Environment defaultInstance;
public static Environment getDefaultInstance() {
return defaultInstance;
}
public Environment getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Environment(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
variable_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
variable_.add(input.readMessage(Messages.Task.Variable.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
variable_ = java.util.Collections.unmodifiableList(variable_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_Environment_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_Environment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.Environment.class, Messages.Task.Environment.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Environment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Environment(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
// repeated .messages.Task.Variable variable = 1;
public static final int VARIABLE_FIELD_NUMBER = 1;
private java.util.List variable_;
/**
* repeated .messages.Task.Variable variable = 1;
*/
public java.util.List getVariableList() {
return variable_;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public java.util.List extends Messages.Task.VariableOrBuilder>
getVariableOrBuilderList() {
return variable_;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public int getVariableCount() {
return variable_.size();
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Messages.Task.Variable getVariable(int index) {
return variable_.get(index);
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Messages.Task.VariableOrBuilder getVariableOrBuilder(
int index) {
return variable_.get(index);
}
private void initFields() {
variable_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
for (int i = 0; i < getVariableCount(); i++) {
if (!getVariable(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < variable_.size(); i++) {
output.writeMessage(1, variable_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < variable_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, variable_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.Task.Environment parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.Environment parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.Environment parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.Environment parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.Environment parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.Environment parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.Task.Environment parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.Task.Environment parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.Task.Environment parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.Environment parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.Task.Environment prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.Task.Environment}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.Task.EnvironmentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_Environment_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_Environment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.Environment.class, Messages.Task.Environment.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.Task.Environment.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getVariableFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (variableBuilder_ == null) {
variable_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
variableBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_Task_Environment_descriptor;
}
public Messages.Task.Environment getDefaultInstanceForType() {
return Messages.Task.Environment.getDefaultInstance();
}
public Messages.Task.Environment build() {
Messages.Task.Environment result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.Task.Environment buildPartial() {
Messages.Task.Environment result = new Messages.Task.Environment(this);
int from_bitField0_ = bitField0_;
if (variableBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
variable_ = java.util.Collections.unmodifiableList(variable_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.variable_ = variable_;
} else {
result.variable_ = variableBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.Task.Environment) {
return mergeFrom((Messages.Task.Environment)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.Task.Environment other) {
if (other == Messages.Task.Environment.getDefaultInstance()) return this;
if (variableBuilder_ == null) {
if (!other.variable_.isEmpty()) {
if (variable_.isEmpty()) {
variable_ = other.variable_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureVariableIsMutable();
variable_.addAll(other.variable_);
}
onChanged();
}
} else {
if (!other.variable_.isEmpty()) {
if (variableBuilder_.isEmpty()) {
variableBuilder_.dispose();
variableBuilder_ = null;
variable_ = other.variable_;
bitField0_ = (bitField0_ & ~0x00000001);
variableBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getVariableFieldBuilder() : null;
} else {
variableBuilder_.addAllMessages(other.variable_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getVariableCount(); i++) {
if (!getVariable(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.Task.Environment parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.Task.Environment) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// repeated .messages.Task.Variable variable = 1;
private java.util.List variable_ =
java.util.Collections.emptyList();
private void ensureVariableIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
variable_ = new java.util.ArrayList(variable_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.Variable, Messages.Task.Variable.Builder, Messages.Task.VariableOrBuilder> variableBuilder_;
/**
* repeated .messages.Task.Variable variable = 1;
*/
public java.util.List getVariableList() {
if (variableBuilder_ == null) {
return java.util.Collections.unmodifiableList(variable_);
} else {
return variableBuilder_.getMessageList();
}
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public int getVariableCount() {
if (variableBuilder_ == null) {
return variable_.size();
} else {
return variableBuilder_.getCount();
}
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Messages.Task.Variable getVariable(int index) {
if (variableBuilder_ == null) {
return variable_.get(index);
} else {
return variableBuilder_.getMessage(index);
}
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder setVariable(
int index, Messages.Task.Variable value) {
if (variableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVariableIsMutable();
variable_.set(index, value);
onChanged();
} else {
variableBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder setVariable(
int index, Messages.Task.Variable.Builder builderForValue) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.set(index, builderForValue.build());
onChanged();
} else {
variableBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder addVariable(Messages.Task.Variable value) {
if (variableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVariableIsMutable();
variable_.add(value);
onChanged();
} else {
variableBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder addVariable(
int index, Messages.Task.Variable value) {
if (variableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVariableIsMutable();
variable_.add(index, value);
onChanged();
} else {
variableBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder addVariable(
Messages.Task.Variable.Builder builderForValue) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.add(builderForValue.build());
onChanged();
} else {
variableBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder addVariable(
int index, Messages.Task.Variable.Builder builderForValue) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.add(index, builderForValue.build());
onChanged();
} else {
variableBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder addAllVariable(
java.lang.Iterable extends Messages.Task.Variable> values) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
super.addAll(values, variable_);
onChanged();
} else {
variableBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder clearVariable() {
if (variableBuilder_ == null) {
variable_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
variableBuilder_.clear();
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Builder removeVariable(int index) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.remove(index);
onChanged();
} else {
variableBuilder_.remove(index);
}
return this;
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Messages.Task.Variable.Builder getVariableBuilder(
int index) {
return getVariableFieldBuilder().getBuilder(index);
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Messages.Task.VariableOrBuilder getVariableOrBuilder(
int index) {
if (variableBuilder_ == null) {
return variable_.get(index); } else {
return variableBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public java.util.List extends Messages.Task.VariableOrBuilder>
getVariableOrBuilderList() {
if (variableBuilder_ != null) {
return variableBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(variable_);
}
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Messages.Task.Variable.Builder addVariableBuilder() {
return getVariableFieldBuilder().addBuilder(
Messages.Task.Variable.getDefaultInstance());
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public Messages.Task.Variable.Builder addVariableBuilder(
int index) {
return getVariableFieldBuilder().addBuilder(
index, Messages.Task.Variable.getDefaultInstance());
}
/**
* repeated .messages.Task.Variable variable = 1;
*/
public java.util.List
getVariableBuilderList() {
return getVariableFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.Variable, Messages.Task.Variable.Builder, Messages.Task.VariableOrBuilder>
getVariableFieldBuilder() {
if (variableBuilder_ == null) {
variableBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.Variable, Messages.Task.Variable.Builder, Messages.Task.VariableOrBuilder>(
variable_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
variable_ = null;
}
return variableBuilder_;
}
// @@protoc_insertion_point(builder_scope:messages.Task.Environment)
}
static {
defaultInstance = new Environment(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.Task.Environment)
}
public interface CommandOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string program = 1;
/**
* required string program = 1;
*/
boolean hasProgram();
/**
* required string program = 1;
*/
java.lang.String getProgram();
/**
* required string program = 1;
*/
com.google.protobuf.ByteString
getProgramBytes();
// repeated string argument = 2;
/**
* repeated string argument = 2;
*/
java.util.List
getArgumentList();
/**
* repeated string argument = 2;
*/
int getArgumentCount();
/**
* repeated string argument = 2;
*/
java.lang.String getArgument(int index);
/**
* repeated string argument = 2;
*/
com.google.protobuf.ByteString
getArgumentBytes(int index);
// optional int32 timeout = 3;
/**
* optional int32 timeout = 3;
*/
boolean hasTimeout();
/**
* optional int32 timeout = 3;
*/
int getTimeout();
}
/**
* Protobuf type {@code messages.Task.Command}
*/
public static final class Command extends
com.google.protobuf.GeneratedMessage
implements CommandOrBuilder {
// Use Command.newBuilder() to construct.
private Command(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private Command(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final Command defaultInstance;
public static Command getDefaultInstance() {
return defaultInstance;
}
public Command getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Command(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
program_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
argument_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
argument_.add(input.readBytes());
break;
}
case 24: {
bitField0_ |= 0x00000002;
timeout_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
argument_ = new com.google.protobuf.UnmodifiableLazyStringList(argument_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_Command_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_Command_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.Command.class, Messages.Task.Command.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public Command parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Command(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string program = 1;
public static final int PROGRAM_FIELD_NUMBER = 1;
private java.lang.Object program_;
/**
* required string program = 1;
*/
public boolean hasProgram() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string program = 1;
*/
public java.lang.String getProgram() {
java.lang.Object ref = program_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
program_ = s;
}
return s;
}
}
/**
* required string program = 1;
*/
public com.google.protobuf.ByteString
getProgramBytes() {
java.lang.Object ref = program_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
program_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated string argument = 2;
public static final int ARGUMENT_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList argument_;
/**
* repeated string argument = 2;
*/
public java.util.List
getArgumentList() {
return argument_;
}
/**
* repeated string argument = 2;
*/
public int getArgumentCount() {
return argument_.size();
}
/**
* repeated string argument = 2;
*/
public java.lang.String getArgument(int index) {
return argument_.get(index);
}
/**
* repeated string argument = 2;
*/
public com.google.protobuf.ByteString
getArgumentBytes(int index) {
return argument_.getByteString(index);
}
// optional int32 timeout = 3;
public static final int TIMEOUT_FIELD_NUMBER = 3;
private int timeout_;
/**
* optional int32 timeout = 3;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 timeout = 3;
*/
public int getTimeout() {
return timeout_;
}
private void initFields() {
program_ = "";
argument_ = com.google.protobuf.LazyStringArrayList.EMPTY;
timeout_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasProgram()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getProgramBytes());
}
for (int i = 0; i < argument_.size(); i++) {
output.writeBytes(2, argument_.getByteString(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(3, timeout_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getProgramBytes());
}
{
int dataSize = 0;
for (int i = 0; i < argument_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(argument_.getByteString(i));
}
size += dataSize;
size += 1 * getArgumentList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, timeout_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.Task.Command parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.Command parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.Command parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task.Command parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task.Command parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.Command parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.Task.Command parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.Task.Command parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.Task.Command parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task.Command parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.Task.Command prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.Task.Command}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.Task.CommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_Command_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_Command_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.Command.class, Messages.Task.Command.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.Task.Command.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
program_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
argument_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
timeout_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_Task_Command_descriptor;
}
public Messages.Task.Command getDefaultInstanceForType() {
return Messages.Task.Command.getDefaultInstance();
}
public Messages.Task.Command build() {
Messages.Task.Command result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.Task.Command buildPartial() {
Messages.Task.Command result = new Messages.Task.Command(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.program_ = program_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
argument_ = new com.google.protobuf.UnmodifiableLazyStringList(
argument_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.argument_ = argument_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.timeout_ = timeout_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.Task.Command) {
return mergeFrom((Messages.Task.Command)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.Task.Command other) {
if (other == Messages.Task.Command.getDefaultInstance()) return this;
if (other.hasProgram()) {
bitField0_ |= 0x00000001;
program_ = other.program_;
onChanged();
}
if (!other.argument_.isEmpty()) {
if (argument_.isEmpty()) {
argument_ = other.argument_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureArgumentIsMutable();
argument_.addAll(other.argument_);
}
onChanged();
}
if (other.hasTimeout()) {
setTimeout(other.getTimeout());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasProgram()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.Task.Command parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.Task.Command) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string program = 1;
private java.lang.Object program_ = "";
/**
* required string program = 1;
*/
public boolean hasProgram() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string program = 1;
*/
public java.lang.String getProgram() {
java.lang.Object ref = program_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
program_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string program = 1;
*/
public com.google.protobuf.ByteString
getProgramBytes() {
java.lang.Object ref = program_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
program_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string program = 1;
*/
public Builder setProgram(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
program_ = value;
onChanged();
return this;
}
/**
* required string program = 1;
*/
public Builder clearProgram() {
bitField0_ = (bitField0_ & ~0x00000001);
program_ = getDefaultInstance().getProgram();
onChanged();
return this;
}
/**
* required string program = 1;
*/
public Builder setProgramBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
program_ = value;
onChanged();
return this;
}
// repeated string argument = 2;
private com.google.protobuf.LazyStringList argument_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureArgumentIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
argument_ = new com.google.protobuf.LazyStringArrayList(argument_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string argument = 2;
*/
public java.util.List
getArgumentList() {
return java.util.Collections.unmodifiableList(argument_);
}
/**
* repeated string argument = 2;
*/
public int getArgumentCount() {
return argument_.size();
}
/**
* repeated string argument = 2;
*/
public java.lang.String getArgument(int index) {
return argument_.get(index);
}
/**
* repeated string argument = 2;
*/
public com.google.protobuf.ByteString
getArgumentBytes(int index) {
return argument_.getByteString(index);
}
/**
* repeated string argument = 2;
*/
public Builder setArgument(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentIsMutable();
argument_.set(index, value);
onChanged();
return this;
}
/**
* repeated string argument = 2;
*/
public Builder addArgument(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentIsMutable();
argument_.add(value);
onChanged();
return this;
}
/**
* repeated string argument = 2;
*/
public Builder addAllArgument(
java.lang.Iterable values) {
ensureArgumentIsMutable();
super.addAll(values, argument_);
onChanged();
return this;
}
/**
* repeated string argument = 2;
*/
public Builder clearArgument() {
argument_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string argument = 2;
*/
public Builder addArgumentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentIsMutable();
argument_.add(value);
onChanged();
return this;
}
// optional int32 timeout = 3;
private int timeout_ ;
/**
* optional int32 timeout = 3;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 timeout = 3;
*/
public int getTimeout() {
return timeout_;
}
/**
* optional int32 timeout = 3;
*/
public Builder setTimeout(int value) {
bitField0_ |= 0x00000004;
timeout_ = value;
onChanged();
return this;
}
/**
* optional int32 timeout = 3;
*/
public Builder clearTimeout() {
bitField0_ = (bitField0_ & ~0x00000004);
timeout_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:messages.Task.Command)
}
static {
defaultInstance = new Command(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.Task.Command)
}
private int bitField0_;
// required int32 jobId = 1;
public static final int JOBID_FIELD_NUMBER = 1;
private int jobId_;
/**
* required int32 jobId = 1;
*/
public boolean hasJobId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 jobId = 1;
*/
public int getJobId() {
return jobId_;
}
// required int32 index = 2;
public static final int INDEX_FIELD_NUMBER = 2;
private int index_;
/**
* required int32 index = 2;
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 index = 2;
*/
public int getIndex() {
return index_;
}
// required .messages.Task.Environment env = 3;
public static final int ENV_FIELD_NUMBER = 3;
private Messages.Task.Environment env_;
/**
* required .messages.Task.Environment env = 3;
*/
public boolean hasEnv() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required .messages.Task.Environment env = 3;
*/
public Messages.Task.Environment getEnv() {
return env_;
}
/**
* required .messages.Task.Environment env = 3;
*/
public Messages.Task.EnvironmentOrBuilder getEnvOrBuilder() {
return env_;
}
// required string cmdId = 4;
public static final int CMDID_FIELD_NUMBER = 4;
private java.lang.Object cmdId_;
/**
* required string cmdId = 4;
*/
public boolean hasCmdId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required string cmdId = 4;
*/
public java.lang.String getCmdId() {
java.lang.Object ref = cmdId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cmdId_ = s;
}
return s;
}
}
/**
* required string cmdId = 4;
*/
public com.google.protobuf.ByteString
getCmdIdBytes() {
java.lang.Object ref = cmdId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cmdId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated .messages.Task.Command command = 5;
public static final int COMMAND_FIELD_NUMBER = 5;
private java.util.List command_;
/**
* repeated .messages.Task.Command command = 5;
*/
public java.util.List getCommandList() {
return command_;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public java.util.List extends Messages.Task.CommandOrBuilder>
getCommandOrBuilderList() {
return command_;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public int getCommandCount() {
return command_.size();
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Messages.Task.Command getCommand(int index) {
return command_.get(index);
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Messages.Task.CommandOrBuilder getCommandOrBuilder(
int index) {
return command_.get(index);
}
// repeated .messages.Task.InputFile inputFile = 6;
public static final int INPUTFILE_FIELD_NUMBER = 6;
private java.util.List inputFile_;
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public java.util.List getInputFileList() {
return inputFile_;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public java.util.List extends Messages.Task.InputFileOrBuilder>
getInputFileOrBuilderList() {
return inputFile_;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public int getInputFileCount() {
return inputFile_.size();
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Messages.Task.InputFile getInputFile(int index) {
return inputFile_.get(index);
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Messages.Task.InputFileOrBuilder getInputFileOrBuilder(
int index) {
return inputFile_.get(index);
}
// repeated .messages.Task.OutputFile outputFile = 7;
public static final int OUTPUTFILE_FIELD_NUMBER = 7;
private java.util.List outputFile_;
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public java.util.List getOutputFileList() {
return outputFile_;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public java.util.List extends Messages.Task.OutputFileOrBuilder>
getOutputFileOrBuilderList() {
return outputFile_;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public int getOutputFileCount() {
return outputFile_.size();
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Messages.Task.OutputFile getOutputFile(int index) {
return outputFile_.get(index);
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Messages.Task.OutputFileOrBuilder getOutputFileOrBuilder(
int index) {
return outputFile_.get(index);
}
// required bool initJob = 8;
public static final int INITJOB_FIELD_NUMBER = 8;
private boolean initJob_;
/**
* required bool initJob = 8;
*/
public boolean hasInitJob() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required bool initJob = 8;
*/
public boolean getInitJob() {
return initJob_;
}
// repeated int32 completedJobId = 9;
public static final int COMPLETEDJOBID_FIELD_NUMBER = 9;
private java.util.List completedJobId_;
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public java.util.List
getCompletedJobIdList() {
return completedJobId_;
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public int getCompletedJobIdCount() {
return completedJobId_.size();
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public int getCompletedJobId(int index) {
return completedJobId_.get(index);
}
private void initFields() {
jobId_ = 0;
index_ = 0;
env_ = Messages.Task.Environment.getDefaultInstance();
cmdId_ = "";
command_ = java.util.Collections.emptyList();
inputFile_ = java.util.Collections.emptyList();
outputFile_ = java.util.Collections.emptyList();
initJob_ = false;
completedJobId_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasJobId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasIndex()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasEnv()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCmdId()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasInitJob()) {
memoizedIsInitialized = 0;
return false;
}
if (!getEnv().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getCommandCount(); i++) {
if (!getCommand(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getInputFileCount(); i++) {
if (!getInputFile(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getOutputFileCount(); i++) {
if (!getOutputFile(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, jobId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, index_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, env_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getCmdIdBytes());
}
for (int i = 0; i < command_.size(); i++) {
output.writeMessage(5, command_.get(i));
}
for (int i = 0; i < inputFile_.size(); i++) {
output.writeMessage(6, inputFile_.get(i));
}
for (int i = 0; i < outputFile_.size(); i++) {
output.writeMessage(7, outputFile_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(8, initJob_);
}
for (int i = 0; i < completedJobId_.size(); i++) {
output.writeInt32(9, completedJobId_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, jobId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, index_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, env_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getCmdIdBytes());
}
for (int i = 0; i < command_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, command_.get(i));
}
for (int i = 0; i < inputFile_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, inputFile_.get(i));
}
for (int i = 0; i < outputFile_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, outputFile_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, initJob_);
}
{
int dataSize = 0;
for (int i = 0; i < completedJobId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(completedJobId_.get(i));
}
size += dataSize;
size += 1 * getCompletedJobIdList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.Task parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.Task parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.Task parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.Task parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.Task parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.Task parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.Task parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.Task prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.Task}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.TaskOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_Task_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_Task_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.Task.class, Messages.Task.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.Task.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getEnvFieldBuilder();
getCommandFieldBuilder();
getInputFileFieldBuilder();
getOutputFileFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
jobId_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
index_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
if (envBuilder_ == null) {
env_ = Messages.Task.Environment.getDefaultInstance();
} else {
envBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
cmdId_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
if (commandBuilder_ == null) {
command_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
commandBuilder_.clear();
}
if (inputFileBuilder_ == null) {
inputFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
inputFileBuilder_.clear();
}
if (outputFileBuilder_ == null) {
outputFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
outputFileBuilder_.clear();
}
initJob_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
completedJobId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_Task_descriptor;
}
public Messages.Task getDefaultInstanceForType() {
return Messages.Task.getDefaultInstance();
}
public Messages.Task build() {
Messages.Task result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.Task buildPartial() {
Messages.Task result = new Messages.Task(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.jobId_ = jobId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.index_ = index_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (envBuilder_ == null) {
result.env_ = env_;
} else {
result.env_ = envBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.cmdId_ = cmdId_;
if (commandBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
command_ = java.util.Collections.unmodifiableList(command_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.command_ = command_;
} else {
result.command_ = commandBuilder_.build();
}
if (inputFileBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
inputFile_ = java.util.Collections.unmodifiableList(inputFile_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.inputFile_ = inputFile_;
} else {
result.inputFile_ = inputFileBuilder_.build();
}
if (outputFileBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
outputFile_ = java.util.Collections.unmodifiableList(outputFile_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.outputFile_ = outputFile_;
} else {
result.outputFile_ = outputFileBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000010;
}
result.initJob_ = initJob_;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
completedJobId_ = java.util.Collections.unmodifiableList(completedJobId_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.completedJobId_ = completedJobId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.Task) {
return mergeFrom((Messages.Task)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.Task other) {
if (other == Messages.Task.getDefaultInstance()) return this;
if (other.hasJobId()) {
setJobId(other.getJobId());
}
if (other.hasIndex()) {
setIndex(other.getIndex());
}
if (other.hasEnv()) {
mergeEnv(other.getEnv());
}
if (other.hasCmdId()) {
bitField0_ |= 0x00000008;
cmdId_ = other.cmdId_;
onChanged();
}
if (commandBuilder_ == null) {
if (!other.command_.isEmpty()) {
if (command_.isEmpty()) {
command_ = other.command_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureCommandIsMutable();
command_.addAll(other.command_);
}
onChanged();
}
} else {
if (!other.command_.isEmpty()) {
if (commandBuilder_.isEmpty()) {
commandBuilder_.dispose();
commandBuilder_ = null;
command_ = other.command_;
bitField0_ = (bitField0_ & ~0x00000010);
commandBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getCommandFieldBuilder() : null;
} else {
commandBuilder_.addAllMessages(other.command_);
}
}
}
if (inputFileBuilder_ == null) {
if (!other.inputFile_.isEmpty()) {
if (inputFile_.isEmpty()) {
inputFile_ = other.inputFile_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureInputFileIsMutable();
inputFile_.addAll(other.inputFile_);
}
onChanged();
}
} else {
if (!other.inputFile_.isEmpty()) {
if (inputFileBuilder_.isEmpty()) {
inputFileBuilder_.dispose();
inputFileBuilder_ = null;
inputFile_ = other.inputFile_;
bitField0_ = (bitField0_ & ~0x00000020);
inputFileBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getInputFileFieldBuilder() : null;
} else {
inputFileBuilder_.addAllMessages(other.inputFile_);
}
}
}
if (outputFileBuilder_ == null) {
if (!other.outputFile_.isEmpty()) {
if (outputFile_.isEmpty()) {
outputFile_ = other.outputFile_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureOutputFileIsMutable();
outputFile_.addAll(other.outputFile_);
}
onChanged();
}
} else {
if (!other.outputFile_.isEmpty()) {
if (outputFileBuilder_.isEmpty()) {
outputFileBuilder_.dispose();
outputFileBuilder_ = null;
outputFile_ = other.outputFile_;
bitField0_ = (bitField0_ & ~0x00000040);
outputFileBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getOutputFileFieldBuilder() : null;
} else {
outputFileBuilder_.addAllMessages(other.outputFile_);
}
}
}
if (other.hasInitJob()) {
setInitJob(other.getInitJob());
}
if (!other.completedJobId_.isEmpty()) {
if (completedJobId_.isEmpty()) {
completedJobId_ = other.completedJobId_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureCompletedJobIdIsMutable();
completedJobId_.addAll(other.completedJobId_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasJobId()) {
return false;
}
if (!hasIndex()) {
return false;
}
if (!hasEnv()) {
return false;
}
if (!hasCmdId()) {
return false;
}
if (!hasInitJob()) {
return false;
}
if (!getEnv().isInitialized()) {
return false;
}
for (int i = 0; i < getCommandCount(); i++) {
if (!getCommand(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getInputFileCount(); i++) {
if (!getInputFile(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getOutputFileCount(); i++) {
if (!getOutputFile(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.Task parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.Task) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 jobId = 1;
private int jobId_ ;
/**
* required int32 jobId = 1;
*/
public boolean hasJobId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 jobId = 1;
*/
public int getJobId() {
return jobId_;
}
/**
* required int32 jobId = 1;
*/
public Builder setJobId(int value) {
bitField0_ |= 0x00000001;
jobId_ = value;
onChanged();
return this;
}
/**
* required int32 jobId = 1;
*/
public Builder clearJobId() {
bitField0_ = (bitField0_ & ~0x00000001);
jobId_ = 0;
onChanged();
return this;
}
// required int32 index = 2;
private int index_ ;
/**
* required int32 index = 2;
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 index = 2;
*/
public int getIndex() {
return index_;
}
/**
* required int32 index = 2;
*/
public Builder setIndex(int value) {
bitField0_ |= 0x00000002;
index_ = value;
onChanged();
return this;
}
/**
* required int32 index = 2;
*/
public Builder clearIndex() {
bitField0_ = (bitField0_ & ~0x00000002);
index_ = 0;
onChanged();
return this;
}
// required .messages.Task.Environment env = 3;
private Messages.Task.Environment env_ = Messages.Task.Environment.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
Messages.Task.Environment, Messages.Task.Environment.Builder, Messages.Task.EnvironmentOrBuilder> envBuilder_;
/**
* required .messages.Task.Environment env = 3;
*/
public boolean hasEnv() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required .messages.Task.Environment env = 3;
*/
public Messages.Task.Environment getEnv() {
if (envBuilder_ == null) {
return env_;
} else {
return envBuilder_.getMessage();
}
}
/**
* required .messages.Task.Environment env = 3;
*/
public Builder setEnv(Messages.Task.Environment value) {
if (envBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
env_ = value;
onChanged();
} else {
envBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* required .messages.Task.Environment env = 3;
*/
public Builder setEnv(
Messages.Task.Environment.Builder builderForValue) {
if (envBuilder_ == null) {
env_ = builderForValue.build();
onChanged();
} else {
envBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* required .messages.Task.Environment env = 3;
*/
public Builder mergeEnv(Messages.Task.Environment value) {
if (envBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
env_ != Messages.Task.Environment.getDefaultInstance()) {
env_ =
Messages.Task.Environment.newBuilder(env_).mergeFrom(value).buildPartial();
} else {
env_ = value;
}
onChanged();
} else {
envBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* required .messages.Task.Environment env = 3;
*/
public Builder clearEnv() {
if (envBuilder_ == null) {
env_ = Messages.Task.Environment.getDefaultInstance();
onChanged();
} else {
envBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* required .messages.Task.Environment env = 3;
*/
public Messages.Task.Environment.Builder getEnvBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getEnvFieldBuilder().getBuilder();
}
/**
* required .messages.Task.Environment env = 3;
*/
public Messages.Task.EnvironmentOrBuilder getEnvOrBuilder() {
if (envBuilder_ != null) {
return envBuilder_.getMessageOrBuilder();
} else {
return env_;
}
}
/**
* required .messages.Task.Environment env = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
Messages.Task.Environment, Messages.Task.Environment.Builder, Messages.Task.EnvironmentOrBuilder>
getEnvFieldBuilder() {
if (envBuilder_ == null) {
envBuilder_ = new com.google.protobuf.SingleFieldBuilder<
Messages.Task.Environment, Messages.Task.Environment.Builder, Messages.Task.EnvironmentOrBuilder>(
env_,
getParentForChildren(),
isClean());
env_ = null;
}
return envBuilder_;
}
// required string cmdId = 4;
private java.lang.Object cmdId_ = "";
/**
* required string cmdId = 4;
*/
public boolean hasCmdId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required string cmdId = 4;
*/
public java.lang.String getCmdId() {
java.lang.Object ref = cmdId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
cmdId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string cmdId = 4;
*/
public com.google.protobuf.ByteString
getCmdIdBytes() {
java.lang.Object ref = cmdId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cmdId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string cmdId = 4;
*/
public Builder setCmdId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
cmdId_ = value;
onChanged();
return this;
}
/**
* required string cmdId = 4;
*/
public Builder clearCmdId() {
bitField0_ = (bitField0_ & ~0x00000008);
cmdId_ = getDefaultInstance().getCmdId();
onChanged();
return this;
}
/**
* required string cmdId = 4;
*/
public Builder setCmdIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
cmdId_ = value;
onChanged();
return this;
}
// repeated .messages.Task.Command command = 5;
private java.util.List command_ =
java.util.Collections.emptyList();
private void ensureCommandIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
command_ = new java.util.ArrayList(command_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.Command, Messages.Task.Command.Builder, Messages.Task.CommandOrBuilder> commandBuilder_;
/**
* repeated .messages.Task.Command command = 5;
*/
public java.util.List getCommandList() {
if (commandBuilder_ == null) {
return java.util.Collections.unmodifiableList(command_);
} else {
return commandBuilder_.getMessageList();
}
}
/**
* repeated .messages.Task.Command command = 5;
*/
public int getCommandCount() {
if (commandBuilder_ == null) {
return command_.size();
} else {
return commandBuilder_.getCount();
}
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Messages.Task.Command getCommand(int index) {
if (commandBuilder_ == null) {
return command_.get(index);
} else {
return commandBuilder_.getMessage(index);
}
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder setCommand(
int index, Messages.Task.Command value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommandIsMutable();
command_.set(index, value);
onChanged();
} else {
commandBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder setCommand(
int index, Messages.Task.Command.Builder builderForValue) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.set(index, builderForValue.build());
onChanged();
} else {
commandBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder addCommand(Messages.Task.Command value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommandIsMutable();
command_.add(value);
onChanged();
} else {
commandBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder addCommand(
int index, Messages.Task.Command value) {
if (commandBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommandIsMutable();
command_.add(index, value);
onChanged();
} else {
commandBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder addCommand(
Messages.Task.Command.Builder builderForValue) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.add(builderForValue.build());
onChanged();
} else {
commandBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder addCommand(
int index, Messages.Task.Command.Builder builderForValue) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.add(index, builderForValue.build());
onChanged();
} else {
commandBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder addAllCommand(
java.lang.Iterable extends Messages.Task.Command> values) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
super.addAll(values, command_);
onChanged();
} else {
commandBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder clearCommand() {
if (commandBuilder_ == null) {
command_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
commandBuilder_.clear();
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Builder removeCommand(int index) {
if (commandBuilder_ == null) {
ensureCommandIsMutable();
command_.remove(index);
onChanged();
} else {
commandBuilder_.remove(index);
}
return this;
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Messages.Task.Command.Builder getCommandBuilder(
int index) {
return getCommandFieldBuilder().getBuilder(index);
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Messages.Task.CommandOrBuilder getCommandOrBuilder(
int index) {
if (commandBuilder_ == null) {
return command_.get(index); } else {
return commandBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .messages.Task.Command command = 5;
*/
public java.util.List extends Messages.Task.CommandOrBuilder>
getCommandOrBuilderList() {
if (commandBuilder_ != null) {
return commandBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(command_);
}
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Messages.Task.Command.Builder addCommandBuilder() {
return getCommandFieldBuilder().addBuilder(
Messages.Task.Command.getDefaultInstance());
}
/**
* repeated .messages.Task.Command command = 5;
*/
public Messages.Task.Command.Builder addCommandBuilder(
int index) {
return getCommandFieldBuilder().addBuilder(
index, Messages.Task.Command.getDefaultInstance());
}
/**
* repeated .messages.Task.Command command = 5;
*/
public java.util.List
getCommandBuilderList() {
return getCommandFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.Command, Messages.Task.Command.Builder, Messages.Task.CommandOrBuilder>
getCommandFieldBuilder() {
if (commandBuilder_ == null) {
commandBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.Command, Messages.Task.Command.Builder, Messages.Task.CommandOrBuilder>(
command_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
command_ = null;
}
return commandBuilder_;
}
// repeated .messages.Task.InputFile inputFile = 6;
private java.util.List inputFile_ =
java.util.Collections.emptyList();
private void ensureInputFileIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
inputFile_ = new java.util.ArrayList(inputFile_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.InputFile, Messages.Task.InputFile.Builder, Messages.Task.InputFileOrBuilder> inputFileBuilder_;
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public java.util.List getInputFileList() {
if (inputFileBuilder_ == null) {
return java.util.Collections.unmodifiableList(inputFile_);
} else {
return inputFileBuilder_.getMessageList();
}
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public int getInputFileCount() {
if (inputFileBuilder_ == null) {
return inputFile_.size();
} else {
return inputFileBuilder_.getCount();
}
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Messages.Task.InputFile getInputFile(int index) {
if (inputFileBuilder_ == null) {
return inputFile_.get(index);
} else {
return inputFileBuilder_.getMessage(index);
}
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder setInputFile(
int index, Messages.Task.InputFile value) {
if (inputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputFileIsMutable();
inputFile_.set(index, value);
onChanged();
} else {
inputFileBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder setInputFile(
int index, Messages.Task.InputFile.Builder builderForValue) {
if (inputFileBuilder_ == null) {
ensureInputFileIsMutable();
inputFile_.set(index, builderForValue.build());
onChanged();
} else {
inputFileBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder addInputFile(Messages.Task.InputFile value) {
if (inputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputFileIsMutable();
inputFile_.add(value);
onChanged();
} else {
inputFileBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder addInputFile(
int index, Messages.Task.InputFile value) {
if (inputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInputFileIsMutable();
inputFile_.add(index, value);
onChanged();
} else {
inputFileBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder addInputFile(
Messages.Task.InputFile.Builder builderForValue) {
if (inputFileBuilder_ == null) {
ensureInputFileIsMutable();
inputFile_.add(builderForValue.build());
onChanged();
} else {
inputFileBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder addInputFile(
int index, Messages.Task.InputFile.Builder builderForValue) {
if (inputFileBuilder_ == null) {
ensureInputFileIsMutable();
inputFile_.add(index, builderForValue.build());
onChanged();
} else {
inputFileBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder addAllInputFile(
java.lang.Iterable extends Messages.Task.InputFile> values) {
if (inputFileBuilder_ == null) {
ensureInputFileIsMutable();
super.addAll(values, inputFile_);
onChanged();
} else {
inputFileBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder clearInputFile() {
if (inputFileBuilder_ == null) {
inputFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
inputFileBuilder_.clear();
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Builder removeInputFile(int index) {
if (inputFileBuilder_ == null) {
ensureInputFileIsMutable();
inputFile_.remove(index);
onChanged();
} else {
inputFileBuilder_.remove(index);
}
return this;
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Messages.Task.InputFile.Builder getInputFileBuilder(
int index) {
return getInputFileFieldBuilder().getBuilder(index);
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Messages.Task.InputFileOrBuilder getInputFileOrBuilder(
int index) {
if (inputFileBuilder_ == null) {
return inputFile_.get(index); } else {
return inputFileBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public java.util.List extends Messages.Task.InputFileOrBuilder>
getInputFileOrBuilderList() {
if (inputFileBuilder_ != null) {
return inputFileBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(inputFile_);
}
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Messages.Task.InputFile.Builder addInputFileBuilder() {
return getInputFileFieldBuilder().addBuilder(
Messages.Task.InputFile.getDefaultInstance());
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public Messages.Task.InputFile.Builder addInputFileBuilder(
int index) {
return getInputFileFieldBuilder().addBuilder(
index, Messages.Task.InputFile.getDefaultInstance());
}
/**
* repeated .messages.Task.InputFile inputFile = 6;
*/
public java.util.List
getInputFileBuilderList() {
return getInputFileFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.InputFile, Messages.Task.InputFile.Builder, Messages.Task.InputFileOrBuilder>
getInputFileFieldBuilder() {
if (inputFileBuilder_ == null) {
inputFileBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.InputFile, Messages.Task.InputFile.Builder, Messages.Task.InputFileOrBuilder>(
inputFile_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
inputFile_ = null;
}
return inputFileBuilder_;
}
// repeated .messages.Task.OutputFile outputFile = 7;
private java.util.List outputFile_ =
java.util.Collections.emptyList();
private void ensureOutputFileIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
outputFile_ = new java.util.ArrayList(outputFile_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.OutputFile, Messages.Task.OutputFile.Builder, Messages.Task.OutputFileOrBuilder> outputFileBuilder_;
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public java.util.List getOutputFileList() {
if (outputFileBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputFile_);
} else {
return outputFileBuilder_.getMessageList();
}
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public int getOutputFileCount() {
if (outputFileBuilder_ == null) {
return outputFile_.size();
} else {
return outputFileBuilder_.getCount();
}
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Messages.Task.OutputFile getOutputFile(int index) {
if (outputFileBuilder_ == null) {
return outputFile_.get(index);
} else {
return outputFileBuilder_.getMessage(index);
}
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder setOutputFile(
int index, Messages.Task.OutputFile value) {
if (outputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputFileIsMutable();
outputFile_.set(index, value);
onChanged();
} else {
outputFileBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder setOutputFile(
int index, Messages.Task.OutputFile.Builder builderForValue) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.set(index, builderForValue.build());
onChanged();
} else {
outputFileBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder addOutputFile(Messages.Task.OutputFile value) {
if (outputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputFileIsMutable();
outputFile_.add(value);
onChanged();
} else {
outputFileBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder addOutputFile(
int index, Messages.Task.OutputFile value) {
if (outputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputFileIsMutable();
outputFile_.add(index, value);
onChanged();
} else {
outputFileBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder addOutputFile(
Messages.Task.OutputFile.Builder builderForValue) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.add(builderForValue.build());
onChanged();
} else {
outputFileBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder addOutputFile(
int index, Messages.Task.OutputFile.Builder builderForValue) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.add(index, builderForValue.build());
onChanged();
} else {
outputFileBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder addAllOutputFile(
java.lang.Iterable extends Messages.Task.OutputFile> values) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
super.addAll(values, outputFile_);
onChanged();
} else {
outputFileBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder clearOutputFile() {
if (outputFileBuilder_ == null) {
outputFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
outputFileBuilder_.clear();
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Builder removeOutputFile(int index) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.remove(index);
onChanged();
} else {
outputFileBuilder_.remove(index);
}
return this;
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Messages.Task.OutputFile.Builder getOutputFileBuilder(
int index) {
return getOutputFileFieldBuilder().getBuilder(index);
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Messages.Task.OutputFileOrBuilder getOutputFileOrBuilder(
int index) {
if (outputFileBuilder_ == null) {
return outputFile_.get(index); } else {
return outputFileBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public java.util.List extends Messages.Task.OutputFileOrBuilder>
getOutputFileOrBuilderList() {
if (outputFileBuilder_ != null) {
return outputFileBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputFile_);
}
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Messages.Task.OutputFile.Builder addOutputFileBuilder() {
return getOutputFileFieldBuilder().addBuilder(
Messages.Task.OutputFile.getDefaultInstance());
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public Messages.Task.OutputFile.Builder addOutputFileBuilder(
int index) {
return getOutputFileFieldBuilder().addBuilder(
index, Messages.Task.OutputFile.getDefaultInstance());
}
/**
* repeated .messages.Task.OutputFile outputFile = 7;
*/
public java.util.List
getOutputFileBuilderList() {
return getOutputFileFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.OutputFile, Messages.Task.OutputFile.Builder, Messages.Task.OutputFileOrBuilder>
getOutputFileFieldBuilder() {
if (outputFileBuilder_ == null) {
outputFileBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
Messages.Task.OutputFile, Messages.Task.OutputFile.Builder, Messages.Task.OutputFileOrBuilder>(
outputFile_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
outputFile_ = null;
}
return outputFileBuilder_;
}
// required bool initJob = 8;
private boolean initJob_ ;
/**
* required bool initJob = 8;
*/
public boolean hasInitJob() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* required bool initJob = 8;
*/
public boolean getInitJob() {
return initJob_;
}
/**
* required bool initJob = 8;
*/
public Builder setInitJob(boolean value) {
bitField0_ |= 0x00000080;
initJob_ = value;
onChanged();
return this;
}
/**
* required bool initJob = 8;
*/
public Builder clearInitJob() {
bitField0_ = (bitField0_ & ~0x00000080);
initJob_ = false;
onChanged();
return this;
}
// repeated int32 completedJobId = 9;
private java.util.List completedJobId_ = java.util.Collections.emptyList();
private void ensureCompletedJobIdIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
completedJobId_ = new java.util.ArrayList(completedJobId_);
bitField0_ |= 0x00000100;
}
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public java.util.List
getCompletedJobIdList() {
return java.util.Collections.unmodifiableList(completedJobId_);
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public int getCompletedJobIdCount() {
return completedJobId_.size();
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public int getCompletedJobId(int index) {
return completedJobId_.get(index);
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public Builder setCompletedJobId(
int index, int value) {
ensureCompletedJobIdIsMutable();
completedJobId_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public Builder addCompletedJobId(int value) {
ensureCompletedJobIdIsMutable();
completedJobId_.add(value);
onChanged();
return this;
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public Builder addAllCompletedJobId(
java.lang.Iterable extends java.lang.Integer> values) {
ensureCompletedJobIdIsMutable();
super.addAll(values, completedJobId_);
onChanged();
return this;
}
/**
* repeated int32 completedJobId = 9;
*
*
* for cleanup
*
*/
public Builder clearCompletedJobId() {
completedJobId_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:messages.Task)
}
static {
defaultInstance = new Task(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.Task)
}
public interface TaskResultOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required int32 exitCode = 1;
/**
* required int32 exitCode = 1;
*/
boolean hasExitCode();
/**
* required int32 exitCode = 1;
*/
int getExitCode();
// required int64 taskDuration = 2;
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
boolean hasTaskDuration();
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
long getTaskDuration();
// repeated int64 commandDuration = 3;
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
java.util.List getCommandDurationList();
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
int getCommandDurationCount();
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
long getCommandDuration(int index);
// required int64 workingDataSize = 4;
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
boolean hasWorkingDataSize();
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
long getWorkingDataSize();
// repeated .messages.TaskResult.OutputFile outputFile = 5;
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
java.util.List
getOutputFileList();
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
Messages.TaskResult.OutputFile getOutputFile(int index);
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
int getOutputFileCount();
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
java.util.List extends Messages.TaskResult.OutputFileOrBuilder>
getOutputFileOrBuilderList();
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
Messages.TaskResult.OutputFileOrBuilder getOutputFileOrBuilder(
int index);
}
/**
* Protobuf type {@code messages.TaskResult}
*/
public static final class TaskResult extends
com.google.protobuf.GeneratedMessage
implements TaskResultOrBuilder {
// Use TaskResult.newBuilder() to construct.
private TaskResult(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TaskResult(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TaskResult defaultInstance;
public static TaskResult getDefaultInstance() {
return defaultInstance;
}
public TaskResult getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TaskResult(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
exitCode_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
taskDuration_ = input.readInt64();
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
commandDuration_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
commandDuration_.add(input.readInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
commandDuration_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
commandDuration_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
case 32: {
bitField0_ |= 0x00000004;
workingDataSize_ = input.readInt64();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
outputFile_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
outputFile_.add(input.readMessage(Messages.TaskResult.OutputFile.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
commandDuration_ = java.util.Collections.unmodifiableList(commandDuration_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
outputFile_ = java.util.Collections.unmodifiableList(outputFile_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_TaskResult_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_TaskResult_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.TaskResult.class, Messages.TaskResult.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TaskResult parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TaskResult(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public interface OutputFileOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// required string name = 1;
/**
* required string name = 1;
*/
boolean hasName();
/**
* required string name = 1;
*/
java.lang.String getName();
/**
* required string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// optional bytes data = 2;
/**
* optional bytes data = 2;
*/
boolean hasData();
/**
* optional bytes data = 2;
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code messages.TaskResult.OutputFile}
*/
public static final class OutputFile extends
com.google.protobuf.GeneratedMessage
implements OutputFileOrBuilder {
// Use OutputFile.newBuilder() to construct.
private OutputFile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private OutputFile(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final OutputFile defaultInstance;
public static OutputFile getDefaultInstance() {
return defaultInstance;
}
public OutputFile getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OutputFile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
data_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_TaskResult_OutputFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_TaskResult_OutputFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.TaskResult.OutputFile.class, Messages.TaskResult.OutputFile.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public OutputFile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OutputFile(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional bytes data = 2;
public static final int DATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString data_;
/**
* optional bytes data = 2;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes data = 2;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
private void initFields() {
name_ = "";
data_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, data_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.TaskResult.OutputFile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.TaskResult.OutputFile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.TaskResult.OutputFile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.TaskResult.OutputFile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.TaskResult.OutputFile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.TaskResult.OutputFile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.TaskResult.OutputFile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.TaskResult.OutputFile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.TaskResult.OutputFile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.TaskResult.OutputFile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.TaskResult.OutputFile prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.TaskResult.OutputFile}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.TaskResult.OutputFileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_TaskResult_OutputFile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_TaskResult_OutputFile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.TaskResult.OutputFile.class, Messages.TaskResult.OutputFile.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.TaskResult.OutputFile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
data_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_TaskResult_OutputFile_descriptor;
}
public Messages.TaskResult.OutputFile getDefaultInstanceForType() {
return Messages.TaskResult.OutputFile.getDefaultInstance();
}
public Messages.TaskResult.OutputFile build() {
Messages.TaskResult.OutputFile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.TaskResult.OutputFile buildPartial() {
Messages.TaskResult.OutputFile result = new Messages.TaskResult.OutputFile(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.data_ = data_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.TaskResult.OutputFile) {
return mergeFrom((Messages.TaskResult.OutputFile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.TaskResult.OutputFile other) {
if (other == Messages.TaskResult.OutputFile.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasData()) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.TaskResult.OutputFile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.TaskResult.OutputFile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string name = 1;
private java.lang.Object name_ = "";
/**
* required string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// optional bytes data = 2;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes data = 2;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes data = 2;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* optional bytes data = 2;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
data_ = value;
onChanged();
return this;
}
/**
* optional bytes data = 2;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:messages.TaskResult.OutputFile)
}
static {
defaultInstance = new OutputFile(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.TaskResult.OutputFile)
}
private int bitField0_;
// required int32 exitCode = 1;
public static final int EXITCODE_FIELD_NUMBER = 1;
private int exitCode_;
/**
* required int32 exitCode = 1;
*/
public boolean hasExitCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 exitCode = 1;
*/
public int getExitCode() {
return exitCode_;
}
// required int64 taskDuration = 2;
public static final int TASKDURATION_FIELD_NUMBER = 2;
private long taskDuration_;
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
public boolean hasTaskDuration() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
public long getTaskDuration() {
return taskDuration_;
}
// repeated int64 commandDuration = 3;
public static final int COMMANDDURATION_FIELD_NUMBER = 3;
private java.util.List commandDuration_;
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public java.util.List
getCommandDurationList() {
return commandDuration_;
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public int getCommandDurationCount() {
return commandDuration_.size();
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public long getCommandDuration(int index) {
return commandDuration_.get(index);
}
// required int64 workingDataSize = 4;
public static final int WORKINGDATASIZE_FIELD_NUMBER = 4;
private long workingDataSize_;
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
public boolean hasWorkingDataSize() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
public long getWorkingDataSize() {
return workingDataSize_;
}
// repeated .messages.TaskResult.OutputFile outputFile = 5;
public static final int OUTPUTFILE_FIELD_NUMBER = 5;
private java.util.List outputFile_;
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public java.util.List getOutputFileList() {
return outputFile_;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public java.util.List extends Messages.TaskResult.OutputFileOrBuilder>
getOutputFileOrBuilderList() {
return outputFile_;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public int getOutputFileCount() {
return outputFile_.size();
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Messages.TaskResult.OutputFile getOutputFile(int index) {
return outputFile_.get(index);
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Messages.TaskResult.OutputFileOrBuilder getOutputFileOrBuilder(
int index) {
return outputFile_.get(index);
}
private void initFields() {
exitCode_ = 0;
taskDuration_ = 0L;
commandDuration_ = java.util.Collections.emptyList();
workingDataSize_ = 0L;
outputFile_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasExitCode()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasTaskDuration()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasWorkingDataSize()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getOutputFileCount(); i++) {
if (!getOutputFile(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, exitCode_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, taskDuration_);
}
for (int i = 0; i < commandDuration_.size(); i++) {
output.writeInt64(3, commandDuration_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(4, workingDataSize_);
}
for (int i = 0; i < outputFile_.size(); i++) {
output.writeMessage(5, outputFile_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, exitCode_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, taskDuration_);
}
{
int dataSize = 0;
for (int i = 0; i < commandDuration_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(commandDuration_.get(i));
}
size += dataSize;
size += 1 * getCommandDurationList().size();
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, workingDataSize_);
}
for (int i = 0; i < outputFile_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, outputFile_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static Messages.TaskResult parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.TaskResult parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.TaskResult parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Messages.TaskResult parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Messages.TaskResult parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.TaskResult parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Messages.TaskResult parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static Messages.TaskResult parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static Messages.TaskResult parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static Messages.TaskResult parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(Messages.TaskResult prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code messages.TaskResult}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements Messages.TaskResultOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Messages.internal_static_messages_TaskResult_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return Messages.internal_static_messages_TaskResult_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Messages.TaskResult.class, Messages.TaskResult.Builder.class);
}
// Construct using com.powsybl.computation.mpi.generated.Messages.TaskResult.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getOutputFileFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
exitCode_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
taskDuration_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
commandDuration_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
workingDataSize_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
if (outputFileBuilder_ == null) {
outputFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
outputFileBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Messages.internal_static_messages_TaskResult_descriptor;
}
public Messages.TaskResult getDefaultInstanceForType() {
return Messages.TaskResult.getDefaultInstance();
}
public Messages.TaskResult build() {
Messages.TaskResult result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public Messages.TaskResult buildPartial() {
Messages.TaskResult result = new Messages.TaskResult(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.exitCode_ = exitCode_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.taskDuration_ = taskDuration_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
commandDuration_ = java.util.Collections.unmodifiableList(commandDuration_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.commandDuration_ = commandDuration_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.workingDataSize_ = workingDataSize_;
if (outputFileBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
outputFile_ = java.util.Collections.unmodifiableList(outputFile_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.outputFile_ = outputFile_;
} else {
result.outputFile_ = outputFileBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Messages.TaskResult) {
return mergeFrom((Messages.TaskResult)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Messages.TaskResult other) {
if (other == Messages.TaskResult.getDefaultInstance()) return this;
if (other.hasExitCode()) {
setExitCode(other.getExitCode());
}
if (other.hasTaskDuration()) {
setTaskDuration(other.getTaskDuration());
}
if (!other.commandDuration_.isEmpty()) {
if (commandDuration_.isEmpty()) {
commandDuration_ = other.commandDuration_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureCommandDurationIsMutable();
commandDuration_.addAll(other.commandDuration_);
}
onChanged();
}
if (other.hasWorkingDataSize()) {
setWorkingDataSize(other.getWorkingDataSize());
}
if (outputFileBuilder_ == null) {
if (!other.outputFile_.isEmpty()) {
if (outputFile_.isEmpty()) {
outputFile_ = other.outputFile_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureOutputFileIsMutable();
outputFile_.addAll(other.outputFile_);
}
onChanged();
}
} else {
if (!other.outputFile_.isEmpty()) {
if (outputFileBuilder_.isEmpty()) {
outputFileBuilder_.dispose();
outputFileBuilder_ = null;
outputFile_ = other.outputFile_;
bitField0_ = (bitField0_ & ~0x00000010);
outputFileBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getOutputFileFieldBuilder() : null;
} else {
outputFileBuilder_.addAllMessages(other.outputFile_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasExitCode()) {
return false;
}
if (!hasTaskDuration()) {
return false;
}
if (!hasWorkingDataSize()) {
return false;
}
for (int i = 0; i < getOutputFileCount(); i++) {
if (!getOutputFile(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Messages.TaskResult parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Messages.TaskResult) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required int32 exitCode = 1;
private int exitCode_ ;
/**
* required int32 exitCode = 1;
*/
public boolean hasExitCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 exitCode = 1;
*/
public int getExitCode() {
return exitCode_;
}
/**
* required int32 exitCode = 1;
*/
public Builder setExitCode(int value) {
bitField0_ |= 0x00000001;
exitCode_ = value;
onChanged();
return this;
}
/**
* required int32 exitCode = 1;
*/
public Builder clearExitCode() {
bitField0_ = (bitField0_ & ~0x00000001);
exitCode_ = 0;
onChanged();
return this;
}
// required int64 taskDuration = 2;
private long taskDuration_ ;
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
public boolean hasTaskDuration() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
public long getTaskDuration() {
return taskDuration_;
}
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
public Builder setTaskDuration(long value) {
bitField0_ |= 0x00000002;
taskDuration_ = value;
onChanged();
return this;
}
/**
* required int64 taskDuration = 2;
*
*
* in ms
*
*/
public Builder clearTaskDuration() {
bitField0_ = (bitField0_ & ~0x00000002);
taskDuration_ = 0L;
onChanged();
return this;
}
// repeated int64 commandDuration = 3;
private java.util.List commandDuration_ = java.util.Collections.emptyList();
private void ensureCommandDurationIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
commandDuration_ = new java.util.ArrayList(commandDuration_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public java.util.List
getCommandDurationList() {
return java.util.Collections.unmodifiableList(commandDuration_);
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public int getCommandDurationCount() {
return commandDuration_.size();
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public long getCommandDuration(int index) {
return commandDuration_.get(index);
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public Builder setCommandDuration(
int index, long value) {
ensureCommandDurationIsMutable();
commandDuration_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public Builder addCommandDuration(long value) {
ensureCommandDurationIsMutable();
commandDuration_.add(value);
onChanged();
return this;
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public Builder addAllCommandDuration(
java.lang.Iterable extends java.lang.Long> values) {
ensureCommandDurationIsMutable();
super.addAll(values, commandDuration_);
onChanged();
return this;
}
/**
* repeated int64 commandDuration = 3;
*
*
* in ms
*
*/
public Builder clearCommandDuration() {
commandDuration_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
// required int64 workingDataSize = 4;
private long workingDataSize_ ;
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
public boolean hasWorkingDataSize() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
public long getWorkingDataSize() {
return workingDataSize_;
}
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
public Builder setWorkingDataSize(long value) {
bitField0_ |= 0x00000008;
workingDataSize_ = value;
onChanged();
return this;
}
/**
* required int64 workingDataSize = 4;
*
*
* in bytes
*
*/
public Builder clearWorkingDataSize() {
bitField0_ = (bitField0_ & ~0x00000008);
workingDataSize_ = 0L;
onChanged();
return this;
}
// repeated .messages.TaskResult.OutputFile outputFile = 5;
private java.util.List outputFile_ =
java.util.Collections.emptyList();
private void ensureOutputFileIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
outputFile_ = new java.util.ArrayList(outputFile_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.TaskResult.OutputFile, Messages.TaskResult.OutputFile.Builder, Messages.TaskResult.OutputFileOrBuilder> outputFileBuilder_;
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public java.util.List getOutputFileList() {
if (outputFileBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputFile_);
} else {
return outputFileBuilder_.getMessageList();
}
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public int getOutputFileCount() {
if (outputFileBuilder_ == null) {
return outputFile_.size();
} else {
return outputFileBuilder_.getCount();
}
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Messages.TaskResult.OutputFile getOutputFile(int index) {
if (outputFileBuilder_ == null) {
return outputFile_.get(index);
} else {
return outputFileBuilder_.getMessage(index);
}
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder setOutputFile(
int index, Messages.TaskResult.OutputFile value) {
if (outputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputFileIsMutable();
outputFile_.set(index, value);
onChanged();
} else {
outputFileBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder setOutputFile(
int index, Messages.TaskResult.OutputFile.Builder builderForValue) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.set(index, builderForValue.build());
onChanged();
} else {
outputFileBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder addOutputFile(Messages.TaskResult.OutputFile value) {
if (outputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputFileIsMutable();
outputFile_.add(value);
onChanged();
} else {
outputFileBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder addOutputFile(
int index, Messages.TaskResult.OutputFile value) {
if (outputFileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputFileIsMutable();
outputFile_.add(index, value);
onChanged();
} else {
outputFileBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder addOutputFile(
Messages.TaskResult.OutputFile.Builder builderForValue) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.add(builderForValue.build());
onChanged();
} else {
outputFileBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder addOutputFile(
int index, Messages.TaskResult.OutputFile.Builder builderForValue) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.add(index, builderForValue.build());
onChanged();
} else {
outputFileBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder addAllOutputFile(
java.lang.Iterable extends Messages.TaskResult.OutputFile> values) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
super.addAll(values, outputFile_);
onChanged();
} else {
outputFileBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder clearOutputFile() {
if (outputFileBuilder_ == null) {
outputFile_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
outputFileBuilder_.clear();
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Builder removeOutputFile(int index) {
if (outputFileBuilder_ == null) {
ensureOutputFileIsMutable();
outputFile_.remove(index);
onChanged();
} else {
outputFileBuilder_.remove(index);
}
return this;
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Messages.TaskResult.OutputFile.Builder getOutputFileBuilder(
int index) {
return getOutputFileFieldBuilder().getBuilder(index);
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Messages.TaskResult.OutputFileOrBuilder getOutputFileOrBuilder(
int index) {
if (outputFileBuilder_ == null) {
return outputFile_.get(index); } else {
return outputFileBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public java.util.List extends Messages.TaskResult.OutputFileOrBuilder>
getOutputFileOrBuilderList() {
if (outputFileBuilder_ != null) {
return outputFileBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputFile_);
}
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Messages.TaskResult.OutputFile.Builder addOutputFileBuilder() {
return getOutputFileFieldBuilder().addBuilder(
Messages.TaskResult.OutputFile.getDefaultInstance());
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public Messages.TaskResult.OutputFile.Builder addOutputFileBuilder(
int index) {
return getOutputFileFieldBuilder().addBuilder(
index, Messages.TaskResult.OutputFile.getDefaultInstance());
}
/**
* repeated .messages.TaskResult.OutputFile outputFile = 5;
*/
public java.util.List
getOutputFileBuilderList() {
return getOutputFileFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
Messages.TaskResult.OutputFile, Messages.TaskResult.OutputFile.Builder, Messages.TaskResult.OutputFileOrBuilder>
getOutputFileFieldBuilder() {
if (outputFileBuilder_ == null) {
outputFileBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
Messages.TaskResult.OutputFile, Messages.TaskResult.OutputFile.Builder, Messages.TaskResult.OutputFileOrBuilder>(
outputFile_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
outputFile_ = null;
}
return outputFileBuilder_;
}
// @@protoc_insertion_point(builder_scope:messages.TaskResult)
}
static {
defaultInstance = new TaskResult(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:messages.TaskResult)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_CommonFile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_CommonFile_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_Task_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_Task_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_Task_InputFile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_Task_InputFile_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_Task_OutputFile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_Task_OutputFile_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_Task_Variable_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_Task_Variable_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_Task_Environment_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_Task_Environment_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_Task_Command_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_Task_Command_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_TaskResult_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_TaskResult_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_messages_TaskResult_OutputFile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_messages_TaskResult_OutputFile_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\016messages.proto\022\010messages\"E\n\nCommonFile" +
"\022\014\n\004name\030\001 \002(\t\022\r\n\005chunk\030\002 \002(\005\022\014\n\004last\030\003 " +
"\002(\010\022\014\n\004data\030\004 \002(\014\"\254\006\n\004Task\022\r\n\005jobId\030\001 \002(" +
"\005\022\r\n\005index\030\002 \002(\005\022\'\n\003env\030\003 \002(\0132\032.messages" +
".Task.Environment\022\r\n\005cmdId\030\004 \002(\t\022\'\n\007comm" +
"and\030\005 \003(\0132\026.messages.Task.Command\022+\n\tinp" +
"utFile\030\006 \003(\0132\030.messages.Task.InputFile\022-" +
"\n\noutputFile\030\007 \003(\0132\031.messages.Task.Outpu" +
"tFile\022\017\n\007initJob\030\010 \002(\010\022\026\n\016completedJobId" +
"\030\t \003(\005\032\366\001\n\tInputFile\022\014\n\004name\030\001 \002(\t\022-\n\005sc",
"ope\030\002 \002(\0162\036.messages.Task.InputFile.Scop" +
"e\022\014\n\004data\030\003 \001(\014\022;\n\014preProcessor\030\004 \002(\0162%." +
"messages.Task.InputFile.PreProcessor\"<\n\014" +
"PreProcessor\022\010\n\004NONE\020\001\022\021\n\rARCHIVE_UNZIP\020" +
"\002\022\017\n\013FILE_GUNZIP\020\003\"#\n\005Scope\022\007\n\003RUN\020\001\022\007\n\003" +
"JOB\020\002\022\010\n\004TASK\020\003\032\204\001\n\nOutputFile\022\014\n\004name\030\001" +
" \002(\t\022>\n\rpostProcessor\030\002 \002(\0162\'.messages.T" +
"ask.OutputFile.PostProcessor\"(\n\rPostProc" +
"essor\022\010\n\004NONE\020\001\022\r\n\tFILE_GZIP\020\002\032\'\n\010Variab" +
"le\022\014\n\004name\030\001 \002(\t\022\r\n\005value\030\002 \002(\t\0328\n\013Envir",
"onment\022)\n\010variable\030\001 \003(\0132\027.messages.Task" +
".Variable\032=\n\007Command\022\017\n\007program\030\001 \002(\t\022\020\n" +
"\010argument\030\002 \003(\t\022\017\n\007timeout\030\003 \001(\005\"\305\001\n\nTas" +
"kResult\022\020\n\010exitCode\030\001 \002(\005\022\024\n\014taskDuratio" +
"n\030\002 \002(\003\022\027\n\017commandDuration\030\003 \003(\003\022\027\n\017work" +
"ingDataSize\030\004 \002(\003\0223\n\noutputFile\030\005 \003(\0132\037." +
"messages.TaskResult.OutputFile\032(\n\nOutput" +
"File\022\014\n\004name\030\001 \002(\t\022\014\n\004data\030\002 \001(\014B6\n*eu.i" +
"tesla_project.computation.mpi.messagesB\010" +
"Messages"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_messages_CommonFile_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_messages_CommonFile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_CommonFile_descriptor,
new java.lang.String[] { "Name", "Chunk", "Last", "Data", });
internal_static_messages_Task_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_messages_Task_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_Task_descriptor,
new java.lang.String[] { "JobId", "Index", "Env", "CmdId", "Command", "InputFile", "OutputFile", "InitJob", "CompletedJobId", });
internal_static_messages_Task_InputFile_descriptor =
internal_static_messages_Task_descriptor.getNestedTypes().get(0);
internal_static_messages_Task_InputFile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_Task_InputFile_descriptor,
new java.lang.String[] { "Name", "Scope", "Data", "PreProcessor", });
internal_static_messages_Task_OutputFile_descriptor =
internal_static_messages_Task_descriptor.getNestedTypes().get(1);
internal_static_messages_Task_OutputFile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_Task_OutputFile_descriptor,
new java.lang.String[] { "Name", "PostProcessor", });
internal_static_messages_Task_Variable_descriptor =
internal_static_messages_Task_descriptor.getNestedTypes().get(2);
internal_static_messages_Task_Variable_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_Task_Variable_descriptor,
new java.lang.String[] { "Name", "Value", });
internal_static_messages_Task_Environment_descriptor =
internal_static_messages_Task_descriptor.getNestedTypes().get(3);
internal_static_messages_Task_Environment_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_Task_Environment_descriptor,
new java.lang.String[] { "Variable", });
internal_static_messages_Task_Command_descriptor =
internal_static_messages_Task_descriptor.getNestedTypes().get(4);
internal_static_messages_Task_Command_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_Task_Command_descriptor,
new java.lang.String[] { "Program", "Argument", "Timeout", });
internal_static_messages_TaskResult_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_messages_TaskResult_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_TaskResult_descriptor,
new java.lang.String[] { "ExitCode", "TaskDuration", "CommandDuration", "WorkingDataSize", "OutputFile", });
internal_static_messages_TaskResult_OutputFile_descriptor =
internal_static_messages_TaskResult_descriptor.getNestedTypes().get(0);
internal_static_messages_TaskResult_OutputFile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_messages_TaskResult_OutputFile_descriptor,
new java.lang.String[] { "Name", "Data", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy