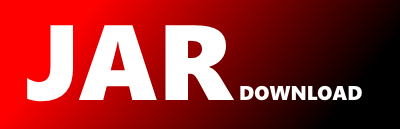
com.powsybl.computation.mpi.MpiJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of powsybl-computation-mpi Show documentation
Show all versions of powsybl-computation-mpi Show documentation
A computation implementation to run computations with MPI
The newest version!
/**
* Copyright (c) 2016, All partners of the iTesla project (http://www.itesla-project.eu/consortium)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* SPDX-License-Identifier: MPL-2.0
*/
package com.powsybl.computation.mpi;
import com.powsybl.computation.CommandExecution;
import com.powsybl.computation.ExecutionError;
import com.powsybl.computation.ExecutionListener;
import com.powsybl.computation.ExecutionReport;
import java.nio.file.Path;
import java.util.*;
import java.util.concurrent.CompletableFuture;
/**
*
* @author Geoffroy Jamgotchian {@literal }
*/
public class MpiJob {
private final int id;
private final CommandExecution execution;
private final ExecutionListener listener;
private final CompletableFuture future;
private final Path workingDir;
private final Map variables;
private int taskIndex = 0;
private final List runningTasks = new ArrayList<>();
private final List errors = new ArrayList<>();
private final Set usedRanks = new HashSet<>();
MpiJob(int id, CommandExecution execution, Path workingDir, Map variables, ExecutionListener listener, CompletableFuture future) {
this.id = id;
this.execution = execution;
this.workingDir = workingDir;
this.variables = variables;
this.listener = new ProfiledExecutionListener(listener);
this.future = future;
}
CommandExecution getExecution() {
return execution;
}
int getId() {
return id;
}
Path getWorkingDir() {
return workingDir;
}
Map getVariables() {
return variables;
}
Map getExecutionVariables() {
return CommandExecution.getExecutionVariables(variables, execution);
}
ExecutionListener getListener() {
return listener;
}
CompletableFuture getFuture() {
return future;
}
int getTaskIndex() {
return taskIndex;
}
void setTaskIndex(int taskIndex) {
this.taskIndex = taskIndex;
}
List getRunningTasks() {
return runningTasks;
}
List getErrors() {
return errors;
}
Set getUsedRanks() {
return usedRanks;
}
boolean isCompleted() {
return taskIndex >= execution.getExecutionCount() && runningTasks.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy