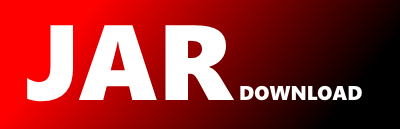
com.powsybl.flow_decomposition.NodalInjectionComputer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of powsybl-flow-decomposition Show documentation
Show all versions of powsybl-flow-decomposition Show documentation
Implementation of ACER methodology for flow decomposition
The newest version!
/*
* Copyright (c) 2022, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.powsybl.flow_decomposition;
import com.powsybl.iidm.network.Country;
import com.powsybl.iidm.network.Injection;
import com.powsybl.iidm.network.Network;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import static com.powsybl.flow_decomposition.DecomposedFlow.ALLOCATED_COLUMN_NAME;
import static com.powsybl.flow_decomposition.DecomposedFlow.NO_FLOW;
import static com.powsybl.flow_decomposition.DecomposedFlow.XNODE_COLUMN_NAME;
/**
* @author Hugo Schindler {@literal }
* @author Sebastien Murgey {@literal }
*/
class NodalInjectionComputer {
private static final double DEFAULT_GLSK_FACTOR = 0.0;
public static final double DEFAULT_NET_POSITION = 0.0;
private final NetworkMatrixIndexes networkMatrixIndexes;
NodalInjectionComputer(NetworkMatrixIndexes networkMatrixIndexes) {
this.networkMatrixIndexes = networkMatrixIndexes;
}
SparseMatrixWithIndexesTriplet run(Network network,
Map> glsks,
Map netPositions) {
ReferenceNodalInjectionComputer referenceNodalInjectionComputer = new ReferenceNodalInjectionComputer();
Map nodalInjectionDcReference = referenceNodalInjectionComputer.run(networkMatrixIndexes.getNodeList());
Map nodalInjectionForXNodeFlow = referenceNodalInjectionComputer.run(networkMatrixIndexes.getUnmergedXNodeList());
Map nodalInjectionsForAllocatedFlow = getNodalInjectionsForAllocatedFlows(glsks, netPositions);
SparseMatrixWithIndexesTriplet nodalInjectionMatrix = getEmptyNodalInjectionMatrix(glsks,
nodalInjectionsForAllocatedFlow.size() + nodalInjectionDcReference.size() + nodalInjectionForXNodeFlow.size());
fillNodalInjectionsWithAllocatedFlow(nodalInjectionsForAllocatedFlow, nodalInjectionMatrix);
fillNodalInjectionsWithXNodeFlow(nodalInjectionForXNodeFlow, nodalInjectionMatrix);
fillNodalInjectionsWithLoopFlow(network, nodalInjectionsForAllocatedFlow, nodalInjectionForXNodeFlow, nodalInjectionDcReference, nodalInjectionMatrix);
return nodalInjectionMatrix;
}
private Map getNodalInjectionsForAllocatedFlows(Map> glsks,
Map netPositions) {
return networkMatrixIndexes.getNodeList().stream()
.collect(Collectors.toMap(
Injection::getId,
injection -> getIndividualNodalInjectionForAllocatedFlows(injection, glsks, netPositions)
)
);
}
private double getIndividualNodalInjectionForAllocatedFlows(Injection> injection,
Map> glsks,
Map netPositions) {
Country injectionCountry = NetworkUtil.getInjectionCountry(injection);
return glsks.get(injectionCountry).getOrDefault(injection.getId(), DEFAULT_GLSK_FACTOR)
* netPositions.getOrDefault(injectionCountry, DEFAULT_NET_POSITION);
}
private SparseMatrixWithIndexesTriplet getEmptyNodalInjectionMatrix(Map> glsks, Integer size) {
List columns = glsks.keySet().stream()
.map(NetworkUtil::getLoopFlowIdFromCountry)
.collect(Collectors.toList());
columns.add(ALLOCATED_COLUMN_NAME);
columns.add(XNODE_COLUMN_NAME);
return new SparseMatrixWithIndexesTriplet(
networkMatrixIndexes.getNodeIndex(), NetworkUtil.getIndex(columns), size);
}
private void fillNodalInjectionsWithAllocatedFlow(Map nodalInjectionsForAllocatedFlow,
SparseMatrixWithIndexesTriplet nodalInjectionMatrix) {
nodalInjectionsForAllocatedFlow.forEach(
(injectionId, injectionValue) -> nodalInjectionMatrix.addItem(injectionId,
ALLOCATED_COLUMN_NAME, injectionValue)
);
}
private void fillNodalInjectionsWithXNodeFlow(Map xNodeInjection,
SparseMatrixWithIndexesTriplet nodalInjectionMatrix) {
xNodeInjection.forEach(
(injectionId, injectionValue) -> nodalInjectionMatrix.addItem(injectionId,
XNODE_COLUMN_NAME, injectionValue)
);
}
private void fillNodalInjectionsWithLoopFlow(Network network,
Map nodalInjectionsForAllocatedFlow,
Map nodalInjectionsForXNodeFlow,
Map nodalInjectionDcReference,
SparseMatrixWithIndexesTriplet nodalInjectionMatrix) {
networkMatrixIndexes.getNodeList().forEach(
node -> {
String nodeId = node.getId();
nodalInjectionMatrix.addItem(
nodeId,
NetworkUtil.getLoopFlowIdFromCountry(network, nodeId),
computeNodalInjectionForLoopFLow(
nodalInjectionDcReference.get(nodeId),
nodalInjectionsForAllocatedFlow.get(nodeId),
nodalInjectionsForXNodeFlow.getOrDefault(nodeId, NO_FLOW)
)
);
});
}
private double computeNodalInjectionForLoopFLow(double referenceDcNodalInjection,
double nodalInjectionForAllocatedFlow,
double nodalInjectionForXNodeFlow) {
return referenceDcNodalInjection - nodalInjectionForAllocatedFlow - nodalInjectionForXNodeFlow;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy