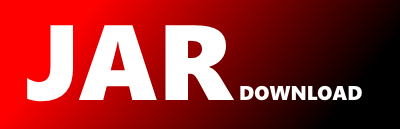
com.powsybl.flow_decomposition.SparseMatrixWithIndexesCSC Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of powsybl-flow-decomposition Show documentation
Show all versions of powsybl-flow-decomposition Show documentation
Implementation of ACER methodology for flow decomposition
The newest version!
/*
* Copyright (c) 2022, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.powsybl.flow_decomposition;
import org.ejml.data.DMatrixSparse;
import org.ejml.data.DMatrixSparseCSC;
import org.ejml.sparse.csc.CommonOps_DSCC;
import java.util.Iterator;
import java.util.Map;
import java.util.TreeMap;
import java.util.stream.Collectors;
/**
* @author Sebastien Murgey {@literal }
* @author Hugo Schindler {@literal }
*/
class SparseMatrixWithIndexesCSC extends AbstractSparseMatrixWithIndexes {
private final DMatrixSparseCSC cscMatrix;
SparseMatrixWithIndexesCSC(Map rowIndex, Map colIndex, DMatrixSparseCSC cscMatrix) {
super(rowIndex, colIndex);
this.cscMatrix = cscMatrix;
}
SparseMatrixWithIndexesCSC(Map rowIndex, Map colIndex) {
this(rowIndex, colIndex, new DMatrixSparseCSC(rowIndex.size(), colIndex.size()));
}
private Map inverseIndex(Map index) {
return index.entrySet()
.stream()
.collect(Collectors.toMap(Map.Entry::getValue, Map.Entry::getKey));
}
Map> toMap() {
Map colIndexInverse = inverseIndex(colIndex);
Map rowIndexInverse = inverseIndex(rowIndex);
Map> result = new TreeMap<>();
for (Iterator iterator = cscMatrix.createCoordinateIterator(); iterator.hasNext(); ) {
DMatrixSparse.CoordinateRealValue cell = iterator.next();
result.computeIfAbsent(rowIndexInverse.get(cell.row), v -> new TreeMap<>())
.put(colIndexInverse.get(cell.col), cell.value);
}
return result;
}
static SparseMatrixWithIndexesCSC mult(SparseMatrixWithIndexesCSC matrix1, SparseMatrixWithIndexesCSC matrix2) {
SparseMatrixWithIndexesCSC multiplicationResult = new SparseMatrixWithIndexesCSC(matrix1.rowIndex, matrix2.colIndex);
CommonOps_DSCC.mult(matrix1.cscMatrix, matrix2.cscMatrix, multiplicationResult.cscMatrix);
return multiplicationResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy