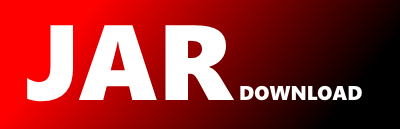
com.powsybl.metrix.mapping.EquipmentTimeSeriesMapperObserver Maven / Gradle / Ivy
/*
* Copyright (c) 2020, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* SPDX-License-Identifier: MPL-2.0
*/
package com.powsybl.metrix.mapping;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Range;
import com.powsybl.iidm.network.Identifiable;
import com.powsybl.iidm.network.Network;
import com.powsybl.timeseries.TimeSeriesIndex;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
/**
* @author Marianne Funfrock {@literal }
*/
public class EquipmentTimeSeriesMapperObserver extends DefaultEquipmentTimeSeriesMapperObserver {
public static final String EQUIPMENT = "equipment";
public static final String VARIABLE = "variable";
protected int currentVersion;
protected int currentPointInChunk;
protected int currentChunk;
protected final int chunkSize;
protected final Range pointRange;
protected final Set equipmentTsKeys;
protected final Set equipmentNotMappedTsKeys;
protected final Map constantValues = new LinkedHashMap<>();
protected final Map values = new LinkedHashMap<>();
private final MappingKeyNetworkValue mappingKeyNetworkValue;
public EquipmentTimeSeriesMapperObserver(Network network, TimeSeriesMappingConfig mappingConfig, int chunkSize, Range pointRange) {
Objects.requireNonNull(network);
Objects.requireNonNull(mappingConfig);
Objects.requireNonNull(pointRange);
this.chunkSize = chunkSize;
this.pointRange = pointRange;
this.mappingKeyNetworkValue = new MappingKeyNetworkValue(network);
TimeSeriesMappingConfigChecker configChecker = new TimeSeriesMappingConfigChecker(mappingConfig);
this.equipmentTsKeys = configChecker.getEquipmentTimeSeriesKeys();
this.equipmentNotMappedTsKeys = configChecker.getNotMappedEquipmentTimeSeriesKeys();
}
@Override
public void versionStart(int version) {
currentVersion = version;
currentChunk = -1;
}
@Override
public void timeSeriesMappingStart(int point, TimeSeriesIndex index) {
if (point != TimeSeriesMapper.CONSTANT_VARIANT_ID) {
if (point % chunkSize == 0) {
// start of chunk
equipmentTsKeys.forEach(key -> values.put(key, new double[Math.min(chunkSize, pointRange.upperEndpoint() - point + 1)]));
currentPointInChunk = 0;
currentChunk++;
} else {
currentPointInChunk++;
}
}
}
@Override
public void timeSeriesMappedToEquipment(int point, String timeSeriesName, Identifiable> identifiable, MappingVariable variable, double equipmentValue) {
MappingKey key = new MappingKey(variable, identifiable.getId());
if (point == TimeSeriesMapper.CONSTANT_VARIANT_ID && equipmentTsKeys.contains(key)) {
constantValues.put(key, new double[]{equipmentValue});
} else if (values.containsKey(key)) {
values.get(key)[currentPointInChunk] = equipmentValue;
}
}
@Override
public void timeSeriesMappingEnd(int point, TimeSeriesIndex index, double balance) {
if (point == TimeSeriesMapper.CONSTANT_VARIANT_ID) {
// end of constant variant
constantValues.forEach((key, doubles) -> addTimeSeries(idAndVariableToTsName(key), currentVersion, pointRange, doubles, computeTags(key), index));
equipmentTsKeys.removeAll(constantValues.keySet());
constantValues.clear();
// add constant time series for not mapped equipments
equipmentNotMappedTsKeys.forEach(key -> addTimeSeries(idAndVariableToTsName(key), currentVersion, pointRange, computeValue(key), computeTags(key), index));
} else {
if (currentPointInChunk == chunkSize - 1 || point == pointRange.upperEndpoint()) {
// end of chunk
Range chunkRange = Range.closed(currentChunk * chunkSize, Math.min((currentChunk + 1) * chunkSize - 1, pointRange.upperEndpoint()));
values.forEach((key, doubles) -> addTimeSeries(idAndVariableToTsName(key), currentVersion, chunkRange, doubles, computeTags(key), index));
values.clear();
}
}
}
@Override
public void versionEnd(int version) {
values.clear();
}
@Override
public void end() {
equipmentTsKeys.clear();
}
protected static String idAndVariableToTsName(MappingKey key) {
// MappingKey(variable, id), entry of mappingConfig equipmentToTimeSeriesMapping -> equipment timeSeriesName _
return key.getId() + "_" + key.getMappingVariable().getVariableName();
}
protected static Map computeTags(MappingKey key) {
return ImmutableMap.of(EQUIPMENT, key.getId(), VARIABLE, key.getMappingVariable().getVariableName());
}
protected double[] computeValue(MappingKey key) {
return new double[]{mappingKeyNetworkValue.getValue(key)};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy