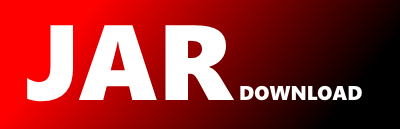
com.powsybl.openloadflow.network.ElementState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of powsybl-open-loadflow Show documentation
Show all versions of powsybl-open-loadflow Show documentation
An open source loadflow based on PowSyBl
The newest version!
/**
* Copyright (c) 2021, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* SPDX-License-Identifier: MPL-2.0
*/
package com.powsybl.openloadflow.network;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* @author Geoffroy Jamgotchian {@literal }
*/
public class ElementState {
protected final T element;
protected final boolean disabled;
public ElementState(T element) {
this.element = Objects.requireNonNull(element);
disabled = element.isDisabled();
}
public void restore() {
element.setDisabled(disabled);
}
public static > List save(Collection elements, Function save) {
Objects.requireNonNull(elements);
Objects.requireNonNull(save);
return elements.stream().map(save).collect(Collectors.toList());
}
public static > void restore(Collection states) {
Objects.requireNonNull(states);
states.forEach(ElementState::restore);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy