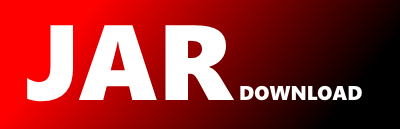
com.powsybl.openloadflow.network.LfTopoConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of powsybl-open-loadflow Show documentation
Show all versions of powsybl-open-loadflow Show documentation
An open source loadflow based on PowSyBl
The newest version!
/**
* Copyright (c) 2023, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* SPDX-License-Identifier: MPL-2.0
*/
package com.powsybl.openloadflow.network;
import com.powsybl.iidm.network.Switch;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
/**
* @author Geoffroy Jamgotchian {@literal }
*/
public class LfTopoConfig {
private final Set switchesToOpen;
private final Set switchesToClose;
private final Set busIdsToLose;
private final Set branchIdsWithPtcToRetain;
private final Set branchIdsWithRtcToRetain;
private final Set shuntIdsToOperate;
private final Set branchIdsOpenableSide1;
private final Set branchIdsOpenableSide2;
private final Set branchIdsToClose;
public LfTopoConfig() {
switchesToOpen = new HashSet<>();
switchesToClose = new HashSet<>();
busIdsToLose = new HashSet<>();
branchIdsWithPtcToRetain = new HashSet<>();
branchIdsWithRtcToRetain = new HashSet<>();
shuntIdsToOperate = new HashSet<>();
branchIdsOpenableSide1 = new HashSet<>();
branchIdsOpenableSide2 = new HashSet<>();
branchIdsToClose = new HashSet<>();
}
public LfTopoConfig(LfTopoConfig other) {
Objects.requireNonNull(other);
this.switchesToOpen = new HashSet<>(other.switchesToOpen);
this.switchesToClose = new HashSet<>(other.switchesToClose);
this.busIdsToLose = new HashSet<>(other.busIdsToLose);
this.branchIdsWithPtcToRetain = new HashSet<>(other.branchIdsWithPtcToRetain);
this.branchIdsWithRtcToRetain = new HashSet<>(other.branchIdsWithRtcToRetain);
this.shuntIdsToOperate = new HashSet<>(other.shuntIdsToOperate);
this.branchIdsOpenableSide1 = new HashSet<>(other.branchIdsOpenableSide1);
this.branchIdsOpenableSide2 = new HashSet<>(other.branchIdsOpenableSide2);
this.branchIdsToClose = new HashSet<>(other.branchIdsToClose);
}
public Set getSwitchesToOpen() {
return switchesToOpen;
}
public Set getSwitchesToClose() {
return switchesToClose;
}
public Set getBusIdsToLose() {
return busIdsToLose;
}
public void addBranchIdWithPtcToRetain(String branchId) {
branchIdsWithPtcToRetain.add(branchId);
}
public void addBranchIdWithRtcToRetain(String branchId) {
branchIdsWithRtcToRetain.add(branchId);
}
public void addShuntIdToOperate(String shuntId) {
shuntIdsToOperate.add(shuntId);
}
public boolean isBreaker() {
return !(switchesToOpen.isEmpty() && switchesToClose.isEmpty() && busIdsToLose.isEmpty());
}
public boolean isRetainedPtc(String branchId) {
return branchIdsWithPtcToRetain.contains(branchId);
}
public boolean isRetainedRtc(String branchId) {
return branchIdsWithRtcToRetain.contains(branchId);
}
public boolean isOperatedShunt(String shuntId) {
return shuntIdsToOperate.contains(shuntId);
}
public Set getBranchIdsOpenableSide1() {
return branchIdsOpenableSide1;
}
public Set getBranchIdsOpenableSide2() {
return branchIdsOpenableSide2;
}
public Set getBranchIdsToClose() {
return branchIdsToClose;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy