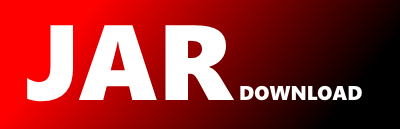
com.powsybl.powerfactory.model.PowerFactoryDataLoader Maven / Gradle / Ivy
/**
* Copyright (c) 2021, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package com.powsybl.powerfactory.model;
import java.io.IOException;
import java.io.InputStream;
import java.io.UncheckedIOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.ServiceLoader;
import java.util.function.Supplier;
import java.util.stream.Collectors;
/**
* @author Geoffroy Jamgotchian
*/
public interface PowerFactoryDataLoader {
@SuppressWarnings("unchecked")
static List> find(Class dataClass) {
Objects.requireNonNull(dataClass);
return ServiceLoader.load(PowerFactoryDataLoader.class).stream()
.map(ServiceLoader.Provider::get)
.filter(loader -> loader.getDataClass() == dataClass)
.map(loader -> (PowerFactoryDataLoader) loader)
.collect(Collectors.toList());
}
static Optional load(Path file, Class dataClass) {
Objects.requireNonNull(file);
return load(file.getFileName().toString(), () -> {
try {
return Files.newInputStream(file);
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}, dataClass);
}
static Optional load(String fileName, Supplier inputStreamSupplier,
Class dataClass) {
return load(fileName, inputStreamSupplier, dataClass, find(dataClass));
}
static Optional load(String fileName, Supplier inputStreamSupplier,
Class dataClass, List> dataLoaders) {
Objects.requireNonNull(fileName);
Objects.requireNonNull(inputStreamSupplier);
Objects.requireNonNull(dataLoaders);
for (PowerFactoryDataLoader dataLoader : dataLoaders) {
if (dataLoader.getDataClass() == dataClass && fileName.endsWith(dataLoader.getExtension())) {
try (var testIs = inputStreamSupplier.get()) {
if (dataLoader.test(testIs)) {
try (var loadIs = inputStreamSupplier.get()) {
return Optional.of(dataLoader.doLoad(fileName, loadIs));
}
}
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
}
return Optional.empty();
}
Class getDataClass();
String getExtension();
boolean test(InputStream is);
T doLoad(String fileName, InputStream is);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy