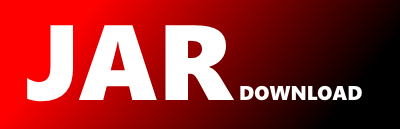
com.powsybl.dataframe.BaseDataframeMapperBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pypowsybl Show documentation
Show all versions of pypowsybl Show documentation
A C interface to powsybl, for pypowsybl implementation
The newest version!
/**
* Copyright (c) 2021, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* SPDX-License-Identifier: MPL-2.0
*/
package com.powsybl.dataframe;
import com.powsybl.commons.PowsyblException;
import com.powsybl.dataframe.update.UpdatingDataframe;
import org.apache.commons.lang3.function.TriFunction;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.function.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Base class for builders of mappers. It uses recursive generic pattern to return the actual
* builder class.
*
* @author Sylvain Leclerc {@literal }
*/
public class BaseDataframeMapperBuilder> {
@FunctionalInterface
public interface ItemGetter {
I getItem(T network, UpdatingDataframe updatingDataframe, int lineNumber);
}
@FunctionalInterface
public interface ItemGetterWithContext {
I getItem(T network, UpdatingDataframe updatingDataframe, int lineNumber, C context);
}
protected BiFunction> itemsProvider;
protected ItemGetterWithContext itemMultiIndexGetter;
protected final List> series = new ArrayList<>();
public B itemsProvider(Function> itemsProvider) {
this.itemsProvider = (t, c) -> itemsProvider.apply(t);
return (B) this;
}
public B itemsProvider(BiFunction> itemsProvider) {
this.itemsProvider = itemsProvider;
return (B) this;
}
public B itemsStreamProvider(Function> itemsProvider) {
return this.itemsProvider((object, context) -> itemsProvider.apply(object).collect(Collectors.toList()));
}
public B itemsStreamProvider(BiFunction> itemsProvider) {
return this.itemsProvider((object, context) -> itemsProvider.apply(object, context).collect(Collectors.toList()));
}
public B itemGetter(BiFunction itemGetter) {
return this.itemGetter((object, context, id) -> itemGetter.apply(object, id));
}
public B itemGetter(TriFunction itemGetter) {
this.itemMultiIndexGetter = (network, updatingDataframe, lineNumber, context) -> {
String id = updatingDataframe.getStringValue("id", lineNumber)
.orElseThrow(() -> new PowsyblException("id is missing"));
return itemGetter.apply(network, context, id);
};
return (B) this;
}
public B itemMultiIndexGetter(ItemGetterWithContext itemMultiIndexGetter) {
this.itemMultiIndexGetter = itemMultiIndexGetter;
return (B) this;
}
public B itemMultiIndexGetter(ItemGetter itemMultiIndexGetter) {
return itemMultiIndexGetter((network, updatingDataframe, lineNumber, context) -> itemMultiIndexGetter.getItem(network, updatingDataframe, lineNumber));
}
public B doubles(String name, ToDoubleFunction value, DoubleSeriesMapper.DoubleUpdater updater) {
return doubles(name, value, updater, true);
}
public B doubles(String name, ToDoubleBiFunction value, DoubleSeriesMapper.DoubleUpdater updater, boolean defaultAttribute) {
series.add(new DoubleSeriesMapper<>(name, value, updater, defaultAttribute));
return (B) this;
}
public B doubles(String name, ToDoubleBiFunction value, DoubleSeriesMapper.DoubleUpdater updater) {
series.add(new DoubleSeriesMapper<>(name, value, updater, true));
return (B) this;
}
public B doubles(String name, ToDoubleFunction value, DoubleSeriesMapper.DoubleUpdater updater, boolean defaultAttribute) {
return doubles(name, (u, pu) -> value.applyAsDouble(u), updater, defaultAttribute);
}
public B doubles(Map> nameValuesMap) {
nameValuesMap.forEach(this::doubles);
return (B) this;
}
public B doubles(String name, ToDoubleFunction value) {
return doubles(name, value, null, true);
}
public B doubles(String name, ToDoubleBiFunction value) {
return doubles(name, value, null, true);
}
public B doubles(String name, ToDoubleBiFunction value, boolean defaultAttribute) {
return doubles(name, value, null, defaultAttribute);
}
public B doubles(String name, ToDoubleFunction value, boolean defaultAttribute) {
return doubles(name, value, null, defaultAttribute);
}
public B ints(String name, ToIntFunction value, IntSeriesMapper.IntUpdater updater) {
return ints(name, value, updater, true);
}
public B ints(String name, ToIntFunction value, IntSeriesMapper.IntUpdater updater, boolean defaultAttribute) {
series.add(new IntSeriesMapper<>(name, value, updater, defaultAttribute));
return (B) this;
}
public B ints(String name, ToIntFunction value) {
return ints(name, value, null, true);
}
public B ints(String name, ToIntFunction value, boolean defaultAttribute) {
return ints(name, value, null, defaultAttribute);
}
public B intsIndex(String name, ToIntFunction value) {
series.add(new IntSeriesMapper<>(name, true, value));
return (B) this;
}
public B booleans(String name, Predicate value, BooleanSeriesMapper.BooleanUpdater updater) {
return booleans(name, value, updater, true);
}
public B booleans(String name, Predicate value, BooleanSeriesMapper.BooleanUpdater updater, boolean defaultAttribute) {
series.add(new BooleanSeriesMapper<>(name, value, updater, defaultAttribute));
return (B) this;
}
public B booleans(String name, Predicate value) {
return booleans(name, value, null, true);
}
public B booleans(String name, Predicate value, boolean defaultAttribute) {
return booleans(name, value, null, defaultAttribute);
}
public B strings(String name, Function value, BiConsumer updater) {
return strings(name, value, updater, true);
}
public B strings(String name, Function value, BiConsumer updater, boolean defaultAttribute) {
series.add(new StringSeriesMapper<>(name, value, updater, defaultAttribute));
return (B) this;
}
public B strings(String name, Function value) {
return strings(name, value, null, true);
}
public B strings(String name, Function value, boolean defaultAttribute) {
return strings(name, value, null, defaultAttribute);
}
public B stringsIndex(String name, Function value) {
series.add(new StringSeriesMapper<>(name, true, value));
return (B) this;
}
public > B enums(String name, Class enumClass, Function value, BiConsumer updater) {
return enums(name, enumClass, value, updater, true);
}
public > B enums(String name, Class enumClass, Function value, BiConsumer updater, boolean defaultAttribute) {
series.add(new EnumSeriesMapper<>(name, enumClass, value, updater, defaultAttribute));
return (B) this;
}
public > B enums(String name, Class enumClass, Function value) {
return enums(name, enumClass, value, null, true);
}
public > B enums(String name, Class enumClass, Function value, boolean defaultAttribute) {
return enums(name, enumClass, value, null, defaultAttribute);
}
public DataframeMapper build() {
return new AbstractDataframeMapper<>(series) {
@Override
protected List getItems(T object, C context) {
return itemsProvider.apply(object, context);
}
@Override
protected U getItem(T object, UpdatingDataframe dataframe, int index, C context) {
return itemMultiIndexGetter.getItem(object, dataframe, index, context);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy