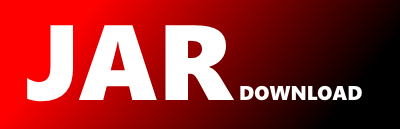
com.powsybl.dataframe.network.adders.SeriesUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pypowsybl Show documentation
Show all versions of pypowsybl Show documentation
A C interface to powsybl, for pypowsybl implementation
The newest version!
/**
* Copyright (c) 2021-2022, RTE (http://www.rte-france.com)
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
* SPDX-License-Identifier: MPL-2.0
*/
package com.powsybl.dataframe.network.adders;
import com.powsybl.commons.PowsyblException;
import com.powsybl.dataframe.update.DoubleSeries;
import com.powsybl.dataframe.update.IntSeries;
import com.powsybl.dataframe.update.StringSeries;
import com.powsybl.dataframe.update.UpdatingDataframe;
import java.util.function.Consumer;
import java.util.function.DoubleConsumer;
import java.util.function.IntConsumer;
/**
* @author Yichen TANG {@literal }
*/
public final class SeriesUtils {
private SeriesUtils() {
}
public static void applyIfPresent(IntSeries series, int index, IntConsumer consumer) {
if (series != null) {
consumer.accept(series.get(index));
}
}
@FunctionalInterface
public interface BooleanConsumer {
void accept(boolean value);
}
public static void applyBooleanIfPresent(IntSeries series, int index, BooleanConsumer consumer) {
if (series != null) {
consumer.accept(series.get(index) == 1);
}
}
public static void applyIfPresent(DoubleSeries series, int index, DoubleConsumer consumer) {
if (series != null) {
consumer.accept(series.get(index));
}
}
public static void applyIfPresent(StringSeries series, int index, Consumer consumer) {
if (series != null) {
consumer.accept(series.get(index));
}
}
public static > void applyIfPresent(StringSeries series, int index, Class enumClass, Consumer consumer) {
if (series != null) {
consumer.accept(Enum.valueOf(enumClass, series.get(index)));
}
}
public static StringSeries getRequiredStrings(UpdatingDataframe dataframe, String name) {
StringSeries series = dataframe.getStrings(name);
if (series == null) {
throwRequiredColumnException(name);
}
return series;
}
public static IntSeries getRequiredInts(UpdatingDataframe dataframe, String name) {
IntSeries series = dataframe.getInts(name);
if (series == null) {
throwRequiredColumnException(name);
}
return series;
}
public static DoubleSeries getRequiredDoubles(UpdatingDataframe dataframe, String name) {
DoubleSeries series = dataframe.getDoubles(name);
if (series == null) {
throwRequiredColumnException(name);
}
return series;
}
private static void throwRequiredColumnException(String name) {
throw new PowsyblException(String.format("Required column %s is missing.", name));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy