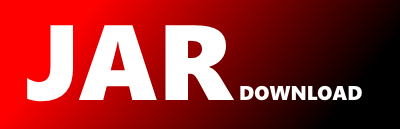
com.ppiech.auto.value.jackson.JacksonAuto Maven / Gradle / Ivy
package com.ppiech.auto.value.jackson;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.*;
public class JacksonAuto {
public static class Builder {
List factories = new ArrayList<>();
public Builder registerJsonMapperFactory(JsonMapperFactory factory) {
factories.add(factory);
return this;
}
public JacksonAuto build() {
factories.add(new DefaultJsonMapperFactory());
return new JacksonAuto(factories);
}
}
private final Map mappers = Collections.synchronizedMap(new HashMap());
private final List factories;
private JacksonAuto(List factories) {
this.factories = factories;
}
/**
* @return Returns a JsonMapper for a given class that has been annotated with @JsonObject.
*
* @param cls The class for which the JsonMapper should be fetched.
* @param Type of mapper for given object class.
* @throws NoSuchMapperException thrown if no matching mapper is found.
*/
public JsonMapper mapperFor(Class cls) throws NoSuchMapperException {
JsonMapper mapper = null;
if (mappers.containsKey(cls)) {
mapper = mappers.get(cls);
} else {
for (JsonMapperFactory factory : factories) {
mapper = factory.mapperFor(this, cls);
if (mapper != null) {
break;
}
}
mappers.put(cls, mapper);
}
if (mapper == null) {
throw new NoSuchMapperException(cls);
}
return mapper;
}
/**
* Parse an object from an InputStream.
*
* @param is The InputStream, most likely from your networking library.
* @param jsonObjectClass The @JsonObject class to parse the InputStream into
* @param Type of object based on given class.
* @return deserialized object
* @throws IOException thrown if there's an error deserializing object
*/
public E parse(InputStream is, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).parse(is);
}
/**
* Parse an object from a String. Note: parsing from an InputStream should be preferred over parsing from a String if possible.
*
* @param jsonString The JSON string being parsed.
* @param jsonObjectClass The @JsonObject class to parse the InputStream into
* @param Type of object based on given class.
* @return deserialized object
* @throws IOException thrown if there's an error deserializing object
*/
public E parse(String jsonString, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).parse(jsonString);
}
/**
* Parse a list of objects from an InputStream.
*
* @param is The inputStream, most likely from your networking library.
* @param jsonObjectClass The @JsonObject class to parse the InputStream into
* @param Type of object based on given class.
* @return deserialized object list
* @throws IOException thrown if there's an error deserializing object
*/
public List parseList(InputStream is, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).parseList(is);
}
/**
* Parse a list of objects from a String. Note: parsing from an InputStream should be preferred over parsing from a String if possible.
*
* @param jsonString The JSON string being parsed.
* @param jsonObjectClass The @JsonObject class to parse the InputStream into
* @param Type of object based on given class.
* @return deserialized object list
* @throws IOException thrown if there's an error deserializing object
*/
public List parseList(String jsonString, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).parseList(jsonString);
}
/**
* Parse a map of objects from an InputStream.
*
* @param is The inputStream, most likely from your networking library.
* @param jsonObjectClass The @JsonObject class to parse the InputStream into
* @param Type of object based on given class.
* @return deserialized object map
* @throws IOException thrown if there's an error deserializing object
*/
public Map parseMap(InputStream is, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).parseMap(is);
}
/**
* Parse a map of objects from a String. Note: parsing from an InputStream should be preferred over parsing from a String if possible.
*
* @param jsonString The JSON string being parsed.
* @param jsonObjectClass The @JsonObject class to parse the InputStream into
* @param Type of object based on given class.
* @return deserialized object map
* @throws IOException thrown if there's an error deserializing object
*/
public Map parseMap(String jsonString, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).parseMap(jsonString);
}
/**
* Serialize an object to a JSON String.
*
* @param object The object to serialize.
* @param Type of object based on given object.
* @throws IOException thrown if there's an error serializing object
* @return JSON string
*/
@SuppressWarnings("unchecked")
public String serialize(E object) throws IOException {
return mapperFor((Class)object.getClass()).serialize(object);
}
/**
* Serialize an object to an OutputStream.
*
* @param object The object to serialize.
* @param os The OutputStream being written to.
* @param Type of object based on given object.
* @throws IOException thrown if there's an error serializing object
*/
@SuppressWarnings("unchecked")
public void serialize(E object, OutputStream os) throws IOException {
mapperFor((Class)object.getClass()).serialize(object, os);
}
/**
* Serialize a list of objects to a JSON String.
*
* @param list The list of objects to serialize.
* @param jsonObjectClass The @JsonObject class of the list elements
* @param Type of object based on given list type.
* @throws IOException thrown if there's an error serializing object
* @return JSON string
*/
public String serialize(List list, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).serialize(list);
}
/**
* Serialize a list of objects to an OutputStream.
*
* @param list The list of objects to serialize.
* @param os The OutputStream to which the list should be serialized
* @param jsonObjectClass The @JsonObject class of the list elements
* @param Type of object based on given list type.
* @throws IOException thrown if there's an error serializing object
*/
public void serialize(List list, OutputStream os, Class jsonObjectClass) throws IOException {
mapperFor(jsonObjectClass).serialize(list, os);
}
/**
* Serialize a map of objects to a JSON String.
*
* @param map The map of objects to serialize.
* @param jsonObjectClass The @JsonObject class of the list elements
* @param Type of object based on given type.
* @throws IOException thrown if there's an error serializing object
* @return JSON string
*/
public String serialize(Map map, Class jsonObjectClass) throws IOException {
return mapperFor(jsonObjectClass).serialize(map);
}
/**
* Serialize a map of objects to an OutputStream.
*
* @param map The map of objects to serialize.
* @param os The OutputStream to which the list should be serialized
* @param jsonObjectClass The @JsonObject class of the list elements
* @param Type of object based on given type.
* @throws IOException thrown if there's an error serializing object
*/
public void serialize(Map map, OutputStream os, Class jsonObjectClass) throws IOException {
mapperFor(jsonObjectClass).serialize(map, os);
}
private static class DefaultJsonMapperFactory implements JsonMapperFactory {
@Override
public JsonMapper mapperFor(JacksonAuto jacksonAuto, Class cls) {
try {
return LoganSquare.mapperFor(cls);
} catch (NoSuchMapperException e) {
return null;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy