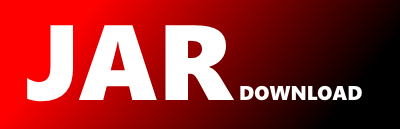
com.processpuzzle.fitnesse.connect.testbed.file.FileSystemStorageService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fitnesse-connect-testbed-backend Show documentation
Show all versions of fitnesse-connect-testbed-backend Show documentation
Test server for integration tests
package com.processpuzzle.fitnesse.connect.testbed.file;
import java.io.IOException;
import java.net.MalformedURLException;
import java.nio.file.FileAlreadyExistsException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.stream.Stream;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.stereotype.Service;
import org.springframework.util.FileSystemUtils;
import org.springframework.web.multipart.MultipartFile;
@Service
public class FileSystemStorageService implements StorageService {
private static final Logger logger = LoggerFactory.getLogger( FileSystemStorageService.class );
private final Path rootLocation;
// constructors
@Autowired public FileSystemStorageService( StorageProperties properties ) {
this.rootLocation = Paths.get( properties.getLocation() );
}
// public accessors and mutators
@Override public void deleteAll() {
FileSystemUtils.deleteRecursively( rootLocation.toFile() );
}
@Override public void init() {
try{
Files.createDirectory( rootLocation );
}catch( FileAlreadyExistsException e ){
logger.info( "File storage location: " + this.rootLocation + " already exists.");
}catch( IOException e ){
throw new StorageException( "Could not initialize storage", e );
}
}
@Override public Path load( String filename ) {
return rootLocation.resolve( filename );
}
@Override public Stream loadAll() {
try{
return Files.walk( this.rootLocation, 1 ).filter( path -> !path.equals( this.rootLocation ) ).map( path -> this.rootLocation.relativize( path ) );
}catch( IOException e ){
throw new StorageException( "Failed to read stored files", e );
}
}
@Override public Resource loadAsResource( String filename ) {
try{
Path file = load( filename );
Resource resource = new UrlResource( file.toUri() );
if( resource.exists() || resource.isReadable() ){
return resource;
}else{
throw new StorageFileNotFoundException( "Could not read file: " + filename );
}
}catch( MalformedURLException e ){
throw new StorageFileNotFoundException( "Could not read file: " + filename, e );
}
}
@Override public void store( MultipartFile file ) {
try{
if( file.isEmpty() ){
throw new StorageException( "Failed to store empty file " + file.getOriginalFilename() );
}
Files.copy( file.getInputStream(), this.rootLocation.resolve( file.getOriginalFilename() ) );
}catch( IOException e ){
throw new StorageException( "Failed to store file " + file.getOriginalFilename(), e );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy