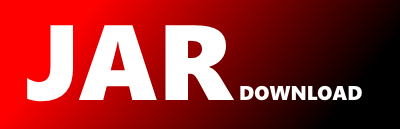
com.lyra.vads.ws.v5.AuthorizationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of PayzenWebServicesSDK Show documentation
Show all versions of PayzenWebServicesSDK Show documentation
SDK to use Payzen SOAP Web Services
package com.lyra.vads.ws.v5;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for authorizationResponse complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="authorizationResponse">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="mode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="amount" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="currency" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="date" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="number" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="result" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "authorizationResponse", propOrder = {
"mode",
"amount",
"currency",
"date",
"number",
"result"
})
public class AuthorizationResponse {
protected String mode;
protected Long amount;
protected Integer currency;
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar date;
protected String number;
protected Integer result;
/**
* Gets the value of the mode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMode() {
return mode;
}
/**
* Sets the value of the mode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMode(String value) {
this.mode = value;
}
/**
* Gets the value of the amount property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getAmount() {
return amount;
}
/**
* Sets the value of the amount property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setAmount(Long value) {
this.amount = value;
}
/**
* Gets the value of the currency property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getCurrency() {
return currency;
}
/**
* Sets the value of the currency property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setCurrency(Integer value) {
this.currency = value;
}
/**
* Gets the value of the date property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDate() {
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setDate(XMLGregorianCalendar value) {
this.date = value;
}
/**
* Gets the value of the number property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNumber() {
return number;
}
/**
* Sets the value of the number property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNumber(String value) {
this.number = value;
}
/**
* Gets the value of the result property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getResult() {
return result;
}
/**
* Sets the value of the result property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setResult(Integer value) {
this.result = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy