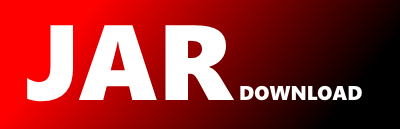
com.lyra.vads.ws.v5.CaptureResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of PayzenWebServicesSDK Show documentation
Show all versions of PayzenWebServicesSDK Show documentation
SDK to use Payzen SOAP Web Services
package com.lyra.vads.ws.v5;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for captureResponse complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="captureResponse">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="date" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="number" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="reconciliationStatus" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="refundAmount" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="refundCurrency" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="chargeback" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "captureResponse", propOrder = {
"date",
"number",
"reconciliationStatus",
"refundAmount",
"refundCurrency",
"chargeback"
})
public class CaptureResponse {
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar date;
protected Integer number;
protected Integer reconciliationStatus;
protected Long refundAmount;
protected Integer refundCurrency;
protected Boolean chargeback;
/**
* Gets the value of the date property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDate() {
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setDate(XMLGregorianCalendar value) {
this.date = value;
}
/**
* Gets the value of the number property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getNumber() {
return number;
}
/**
* Sets the value of the number property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setNumber(Integer value) {
this.number = value;
}
/**
* Gets the value of the reconciliationStatus property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getReconciliationStatus() {
return reconciliationStatus;
}
/**
* Sets the value of the reconciliationStatus property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setReconciliationStatus(Integer value) {
this.reconciliationStatus = value;
}
/**
* Gets the value of the refundAmount property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getRefundAmount() {
return refundAmount;
}
/**
* Sets the value of the refundAmount property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setRefundAmount(Long value) {
this.refundAmount = value;
}
/**
* Gets the value of the refundCurrency property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getRefundCurrency() {
return refundCurrency;
}
/**
* Sets the value of the refundCurrency property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setRefundCurrency(Integer value) {
this.refundCurrency = value;
}
/**
* Gets the value of the chargeback property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isChargeback() {
return chargeback;
}
/**
* Sets the value of the chargeback property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setChargeback(Boolean value) {
this.chargeback = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy