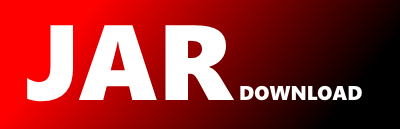
com.lyra.vads.ws.v5.CardRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of PayzenWebServicesSDK Show documentation
Show all versions of PayzenWebServicesSDK Show documentation
SDK to use Payzen SOAP Web Services
package com.lyra.vads.ws.v5;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for cardRequest complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="cardRequest">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="number" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="scheme" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="expiryMonth" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="expiryYear" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="cardSecurityCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="cardHolderBirthDay" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="paymentToken" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "cardRequest", propOrder = {
"number",
"scheme",
"expiryMonth",
"expiryYear",
"cardSecurityCode",
"cardHolderBirthDay",
"paymentToken"
})
public class CardRequest {
protected String number;
protected String scheme;
protected Integer expiryMonth;
protected Integer expiryYear;
protected String cardSecurityCode;
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar cardHolderBirthDay;
protected String paymentToken;
/**
* Gets the value of the number property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNumber() {
return number;
}
/**
* Sets the value of the number property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNumber(String value) {
this.number = value;
}
/**
* Gets the value of the scheme property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getScheme() {
return scheme;
}
/**
* Sets the value of the scheme property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setScheme(String value) {
this.scheme = value;
}
/**
* Gets the value of the expiryMonth property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getExpiryMonth() {
return expiryMonth;
}
/**
* Sets the value of the expiryMonth property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setExpiryMonth(Integer value) {
this.expiryMonth = value;
}
/**
* Gets the value of the expiryYear property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getExpiryYear() {
return expiryYear;
}
/**
* Sets the value of the expiryYear property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setExpiryYear(Integer value) {
this.expiryYear = value;
}
/**
* Gets the value of the cardSecurityCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardSecurityCode() {
return cardSecurityCode;
}
/**
* Sets the value of the cardSecurityCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCardSecurityCode(String value) {
this.cardSecurityCode = value;
}
/**
* Gets the value of the cardHolderBirthDay property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getCardHolderBirthDay() {
return cardHolderBirthDay;
}
/**
* Sets the value of the cardHolderBirthDay property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setCardHolderBirthDay(XMLGregorianCalendar value) {
this.cardHolderBirthDay = value;
}
/**
* Gets the value of the paymentToken property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPaymentToken() {
return paymentToken;
}
/**
* Sets the value of the paymentToken property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPaymentToken(String value) {
this.paymentToken = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy