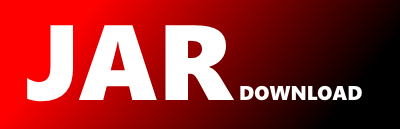
com.lyra.vads.ws.v5.CardResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of PayzenWebServicesSDK Show documentation
Show all versions of PayzenWebServicesSDK Show documentation
SDK to use Payzen SOAP Web Services
package com.lyra.vads.ws.v5;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for cardResponse complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="cardResponse">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="number" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="scheme" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="brand" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="country" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="productCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="bankCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="expiryMonth" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="expiryYear" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "cardResponse", propOrder = {
"number",
"scheme",
"brand",
"country",
"productCode",
"bankCode",
"expiryMonth",
"expiryYear"
})
public class CardResponse {
protected String number;
protected String scheme;
protected String brand;
protected String country;
protected String productCode;
protected String bankCode;
protected Integer expiryMonth;
protected Integer expiryYear;
/**
* Gets the value of the number property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNumber() {
return number;
}
/**
* Sets the value of the number property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNumber(String value) {
this.number = value;
}
/**
* Gets the value of the scheme property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getScheme() {
return scheme;
}
/**
* Sets the value of the scheme property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setScheme(String value) {
this.scheme = value;
}
/**
* Gets the value of the brand property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBrand() {
return brand;
}
/**
* Sets the value of the brand property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBrand(String value) {
this.brand = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
/**
* Gets the value of the productCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductCode() {
return productCode;
}
/**
* Sets the value of the productCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductCode(String value) {
this.productCode = value;
}
/**
* Gets the value of the bankCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBankCode() {
return bankCode;
}
/**
* Sets the value of the bankCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBankCode(String value) {
this.bankCode = value;
}
/**
* Gets the value of the expiryMonth property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getExpiryMonth() {
return expiryMonth;
}
/**
* Sets the value of the expiryMonth property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setExpiryMonth(Integer value) {
this.expiryMonth = value;
}
/**
* Gets the value of the expiryYear property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getExpiryYear() {
return expiryYear;
}
/**
* Sets the value of the expiryYear property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setExpiryYear(Integer value) {
this.expiryYear = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy