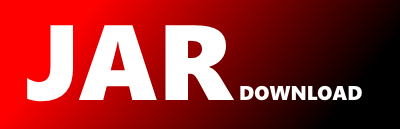
com.lyra.vads.ws.v5.CartItemInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of PayzenWebServicesSDK Show documentation
Show all versions of PayzenWebServicesSDK Show documentation
SDK to use Payzen SOAP Web Services
package com.lyra.vads.ws.v5;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for cartItemInfo complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="cartItemInfo">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="productLabel" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="productType" type="{http://v5.ws.vads.lyra.com/}productType" minOccurs="0"/>
* <element name="productRef" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="productQty" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="productAmount" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="productVat" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="productExtId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "cartItemInfo", propOrder = {
"productLabel",
"productType",
"productRef",
"productQty",
"productAmount",
"productVat",
"productExtId"
})
public class CartItemInfo {
protected String productLabel;
protected ProductType productType;
protected String productRef;
protected Integer productQty;
protected String productAmount;
protected String productVat;
protected String productExtId;
/**
* Gets the value of the productLabel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductLabel() {
return productLabel;
}
/**
* Sets the value of the productLabel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductLabel(String value) {
this.productLabel = value;
}
/**
* Gets the value of the productType property.
*
* @return
* possible object is
* {@link ProductType }
*
*/
public ProductType getProductType() {
return productType;
}
/**
* Sets the value of the productType property.
*
* @param value
* allowed object is
* {@link ProductType }
*
*/
public void setProductType(ProductType value) {
this.productType = value;
}
/**
* Gets the value of the productRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductRef() {
return productRef;
}
/**
* Sets the value of the productRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductRef(String value) {
this.productRef = value;
}
/**
* Gets the value of the productQty property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getProductQty() {
return productQty;
}
/**
* Sets the value of the productQty property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setProductQty(Integer value) {
this.productQty = value;
}
/**
* Gets the value of the productAmount property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductAmount() {
return productAmount;
}
/**
* Sets the value of the productAmount property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductAmount(String value) {
this.productAmount = value;
}
/**
* Gets the value of the productVat property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductVat() {
return productVat;
}
/**
* Sets the value of the productVat property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductVat(String value) {
this.productVat = value;
}
/**
* Gets the value of the productExtId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductExtId() {
return productExtId;
}
/**
* Sets the value of the productExtId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductExtId(String value) {
this.productExtId = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy