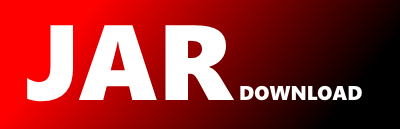
com.lyra.vads.ws.v5.ShippingDetailsRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of PayzenWebServicesSDK Show documentation
Show all versions of PayzenWebServicesSDK Show documentation
SDK to use Payzen SOAP Web Services
package com.lyra.vads.ws.v5;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for shippingDetailsRequest complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="shippingDetailsRequest">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="type" type="{http://v5.ws.vads.lyra.com/}custStatus" minOccurs="0"/>
* <element name="firstName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="lastName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="phoneNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="streetNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="address" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="address2" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="district" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="zipCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="city" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="state" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="country" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="deliveryCompanyName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="shippingSpeed" type="{http://v5.ws.vads.lyra.com/}deliverySpeed" minOccurs="0"/>
* <element name="shippingMethod" type="{http://v5.ws.vads.lyra.com/}deliveryType" minOccurs="0"/>
* <element name="legalName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="identityCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "shippingDetailsRequest", propOrder = {
"type",
"firstName",
"lastName",
"phoneNumber",
"streetNumber",
"address",
"address2",
"district",
"zipCode",
"city",
"state",
"country",
"deliveryCompanyName",
"shippingSpeed",
"shippingMethod",
"legalName",
"identityCode"
})
public class ShippingDetailsRequest {
protected CustStatus type;
protected String firstName;
protected String lastName;
protected String phoneNumber;
protected String streetNumber;
protected String address;
protected String address2;
protected String district;
protected String zipCode;
protected String city;
protected String state;
protected String country;
protected String deliveryCompanyName;
protected DeliverySpeed shippingSpeed;
protected DeliveryType shippingMethod;
protected String legalName;
protected String identityCode;
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link CustStatus }
*
*/
public CustStatus getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link CustStatus }
*
*/
public void setType(CustStatus value) {
this.type = value;
}
/**
* Gets the value of the firstName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFirstName() {
return firstName;
}
/**
* Sets the value of the firstName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFirstName(String value) {
this.firstName = value;
}
/**
* Gets the value of the lastName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLastName() {
return lastName;
}
/**
* Sets the value of the lastName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLastName(String value) {
this.lastName = value;
}
/**
* Gets the value of the phoneNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPhoneNumber() {
return phoneNumber;
}
/**
* Sets the value of the phoneNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPhoneNumber(String value) {
this.phoneNumber = value;
}
/**
* Gets the value of the streetNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreetNumber() {
return streetNumber;
}
/**
* Sets the value of the streetNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreetNumber(String value) {
this.streetNumber = value;
}
/**
* Gets the value of the address property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAddress() {
return address;
}
/**
* Sets the value of the address property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAddress(String value) {
this.address = value;
}
/**
* Gets the value of the address2 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAddress2() {
return address2;
}
/**
* Sets the value of the address2 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAddress2(String value) {
this.address2 = value;
}
/**
* Gets the value of the district property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDistrict() {
return district;
}
/**
* Sets the value of the district property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDistrict(String value) {
this.district = value;
}
/**
* Gets the value of the zipCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getZipCode() {
return zipCode;
}
/**
* Sets the value of the zipCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setZipCode(String value) {
this.zipCode = value;
}
/**
* Gets the value of the city property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCity() {
return city;
}
/**
* Sets the value of the city property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCity(String value) {
this.city = value;
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getState() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setState(String value) {
this.state = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
/**
* Gets the value of the deliveryCompanyName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeliveryCompanyName() {
return deliveryCompanyName;
}
/**
* Sets the value of the deliveryCompanyName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeliveryCompanyName(String value) {
this.deliveryCompanyName = value;
}
/**
* Gets the value of the shippingSpeed property.
*
* @return
* possible object is
* {@link DeliverySpeed }
*
*/
public DeliverySpeed getShippingSpeed() {
return shippingSpeed;
}
/**
* Sets the value of the shippingSpeed property.
*
* @param value
* allowed object is
* {@link DeliverySpeed }
*
*/
public void setShippingSpeed(DeliverySpeed value) {
this.shippingSpeed = value;
}
/**
* Gets the value of the shippingMethod property.
*
* @return
* possible object is
* {@link DeliveryType }
*
*/
public DeliveryType getShippingMethod() {
return shippingMethod;
}
/**
* Sets the value of the shippingMethod property.
*
* @param value
* allowed object is
* {@link DeliveryType }
*
*/
public void setShippingMethod(DeliveryType value) {
this.shippingMethod = value;
}
/**
* Gets the value of the legalName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLegalName() {
return legalName;
}
/**
* Sets the value of the legalName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLegalName(String value) {
this.legalName = value;
}
/**
* Gets the value of the identityCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIdentityCode() {
return identityCode;
}
/**
* Sets the value of the identityCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIdentityCode(String value) {
this.identityCode = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy