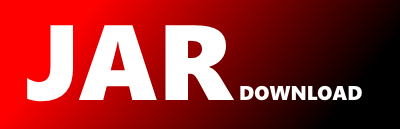
com.lyra.vads.ws.v5.TransactionItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of PayzenWebServicesSDK Show documentation
Show all versions of PayzenWebServicesSDK Show documentation
SDK to use Payzen SOAP Web Services
package com.lyra.vads.ws.v5;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for transactionItem complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="transactionItem">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="transactionUuid" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="transactionStatusLabel" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="amount" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="currency" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="expectedCaptureDate" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "transactionItem", propOrder = {
"transactionUuid",
"transactionStatusLabel",
"amount",
"currency",
"expectedCaptureDate"
})
public class TransactionItem {
protected String transactionUuid;
protected String transactionStatusLabel;
protected Long amount;
protected Integer currency;
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar expectedCaptureDate;
/**
* Gets the value of the transactionUuid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionUuid() {
return transactionUuid;
}
/**
* Sets the value of the transactionUuid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionUuid(String value) {
this.transactionUuid = value;
}
/**
* Gets the value of the transactionStatusLabel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionStatusLabel() {
return transactionStatusLabel;
}
/**
* Sets the value of the transactionStatusLabel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionStatusLabel(String value) {
this.transactionStatusLabel = value;
}
/**
* Gets the value of the amount property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getAmount() {
return amount;
}
/**
* Sets the value of the amount property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setAmount(Long value) {
this.amount = value;
}
/**
* Gets the value of the currency property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getCurrency() {
return currency;
}
/**
* Sets the value of the currency property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setCurrency(Integer value) {
this.currency = value;
}
/**
* Gets the value of the expectedCaptureDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getExpectedCaptureDate() {
return expectedCaptureDate;
}
/**
* Sets the value of the expectedCaptureDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setExpectedCaptureDate(XMLGregorianCalendar value) {
this.expectedCaptureDate = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy