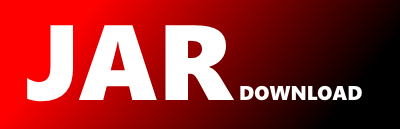
com.prowidesoftware.swift.model.mx.dic.Baseline4 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Specifies the commercial details of the underlying transaction.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Baseline4", propOrder = {
"submitrBaselnId",
"svcCd",
"purchsOrdrRef",
"buyr",
"sellr",
"buyrBk",
"sellrBk",
"buyrSdSubmitgBk",
"sellrSdSubmitgBk",
"bllTo",
"shipTo",
"consgn",
"goods",
"pmtTerms",
"sttlmTerms",
"pmtOblgtn",
"latstMtchDt",
"comrclDataSetReqrd",
"trnsprtDataSetReqrd",
"insrncDataSetReqrd",
"certDataSetReqrd",
"othrCertDataSetReqrd",
"inttToPayXpctd"
})
public class Baseline4 {
@XmlElement(name = "SubmitrBaselnId", required = true)
protected DocumentIdentification1 submitrBaselnId;
@XmlElement(name = "SvcCd", required = true)
@XmlSchemaType(name = "string")
protected TradeFinanceService2Code svcCd;
@XmlElement(name = "PurchsOrdrRef", required = true)
protected DocumentIdentification7 purchsOrdrRef;
@XmlElement(name = "Buyr", required = true)
protected PartyIdentification26 buyr;
@XmlElement(name = "Sellr", required = true)
protected PartyIdentification26 sellr;
@XmlElement(name = "BuyrBk", required = true)
protected BICIdentification1 buyrBk;
@XmlElement(name = "SellrBk", required = true)
protected BICIdentification1 sellrBk;
@XmlElement(name = "BuyrSdSubmitgBk")
protected List buyrSdSubmitgBk;
@XmlElement(name = "SellrSdSubmitgBk")
protected List sellrSdSubmitgBk;
@XmlElement(name = "BllTo")
protected PartyIdentification26 bllTo;
@XmlElement(name = "ShipTo")
protected PartyIdentification26 shipTo;
@XmlElement(name = "Consgn")
protected PartyIdentification26 consgn;
@XmlElement(name = "Goods", required = true)
protected LineItem11 goods;
@XmlElement(name = "PmtTerms", required = true)
protected List pmtTerms;
@XmlElement(name = "SttlmTerms")
protected SettlementTerms3 sttlmTerms;
@XmlElement(name = "PmtOblgtn")
protected List pmtOblgtn;
@XmlElement(name = "LatstMtchDt")
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar latstMtchDt;
@XmlElement(name = "ComrclDataSetReqrd", required = true)
protected RequiredSubmission2 comrclDataSetReqrd;
@XmlElement(name = "TrnsprtDataSetReqrd")
protected RequiredSubmission2 trnsprtDataSetReqrd;
@XmlElement(name = "InsrncDataSetReqrd")
protected RequiredSubmission3 insrncDataSetReqrd;
@XmlElement(name = "CertDataSetReqrd")
protected List certDataSetReqrd;
@XmlElement(name = "OthrCertDataSetReqrd")
protected List othrCertDataSetReqrd;
@XmlElement(name = "InttToPayXpctd")
protected boolean inttToPayXpctd;
/**
* Gets the value of the submitrBaselnId property.
*
* @return
* possible object is
* {@link DocumentIdentification1 }
*
*/
public DocumentIdentification1 getSubmitrBaselnId() {
return submitrBaselnId;
}
/**
* Sets the value of the submitrBaselnId property.
*
* @param value
* allowed object is
* {@link DocumentIdentification1 }
*
*/
public Baseline4 setSubmitrBaselnId(DocumentIdentification1 value) {
this.submitrBaselnId = value;
return this;
}
/**
* Gets the value of the svcCd property.
*
* @return
* possible object is
* {@link TradeFinanceService2Code }
*
*/
public TradeFinanceService2Code getSvcCd() {
return svcCd;
}
/**
* Sets the value of the svcCd property.
*
* @param value
* allowed object is
* {@link TradeFinanceService2Code }
*
*/
public Baseline4 setSvcCd(TradeFinanceService2Code value) {
this.svcCd = value;
return this;
}
/**
* Gets the value of the purchsOrdrRef property.
*
* @return
* possible object is
* {@link DocumentIdentification7 }
*
*/
public DocumentIdentification7 getPurchsOrdrRef() {
return purchsOrdrRef;
}
/**
* Sets the value of the purchsOrdrRef property.
*
* @param value
* allowed object is
* {@link DocumentIdentification7 }
*
*/
public Baseline4 setPurchsOrdrRef(DocumentIdentification7 value) {
this.purchsOrdrRef = value;
return this;
}
/**
* Gets the value of the buyr property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getBuyr() {
return buyr;
}
/**
* Sets the value of the buyr property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public Baseline4 setBuyr(PartyIdentification26 value) {
this.buyr = value;
return this;
}
/**
* Gets the value of the sellr property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getSellr() {
return sellr;
}
/**
* Sets the value of the sellr property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public Baseline4 setSellr(PartyIdentification26 value) {
this.sellr = value;
return this;
}
/**
* Gets the value of the buyrBk property.
*
* @return
* possible object is
* {@link BICIdentification1 }
*
*/
public BICIdentification1 getBuyrBk() {
return buyrBk;
}
/**
* Sets the value of the buyrBk property.
*
* @param value
* allowed object is
* {@link BICIdentification1 }
*
*/
public Baseline4 setBuyrBk(BICIdentification1 value) {
this.buyrBk = value;
return this;
}
/**
* Gets the value of the sellrBk property.
*
* @return
* possible object is
* {@link BICIdentification1 }
*
*/
public BICIdentification1 getSellrBk() {
return sellrBk;
}
/**
* Sets the value of the sellrBk property.
*
* @param value
* allowed object is
* {@link BICIdentification1 }
*
*/
public Baseline4 setSellrBk(BICIdentification1 value) {
this.sellrBk = value;
return this;
}
/**
* Gets the value of the buyrSdSubmitgBk property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the buyrSdSubmitgBk property.
*
*
* For example, to add a new item, do as follows:
*
* getBuyrSdSubmitgBk().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BICIdentification1 }
*
*
*/
public List getBuyrSdSubmitgBk() {
if (buyrSdSubmitgBk == null) {
buyrSdSubmitgBk = new ArrayList();
}
return this.buyrSdSubmitgBk;
}
/**
* Gets the value of the sellrSdSubmitgBk property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the sellrSdSubmitgBk property.
*
*
* For example, to add a new item, do as follows:
*
* getSellrSdSubmitgBk().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BICIdentification1 }
*
*
*/
public List getSellrSdSubmitgBk() {
if (sellrSdSubmitgBk == null) {
sellrSdSubmitgBk = new ArrayList();
}
return this.sellrSdSubmitgBk;
}
/**
* Gets the value of the bllTo property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getBllTo() {
return bllTo;
}
/**
* Sets the value of the bllTo property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public Baseline4 setBllTo(PartyIdentification26 value) {
this.bllTo = value;
return this;
}
/**
* Gets the value of the shipTo property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getShipTo() {
return shipTo;
}
/**
* Sets the value of the shipTo property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public Baseline4 setShipTo(PartyIdentification26 value) {
this.shipTo = value;
return this;
}
/**
* Gets the value of the consgn property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getConsgn() {
return consgn;
}
/**
* Sets the value of the consgn property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public Baseline4 setConsgn(PartyIdentification26 value) {
this.consgn = value;
return this;
}
/**
* Gets the value of the goods property.
*
* @return
* possible object is
* {@link LineItem11 }
*
*/
public LineItem11 getGoods() {
return goods;
}
/**
* Sets the value of the goods property.
*
* @param value
* allowed object is
* {@link LineItem11 }
*
*/
public Baseline4 setGoods(LineItem11 value) {
this.goods = value;
return this;
}
/**
* Gets the value of the pmtTerms property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the pmtTerms property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtTerms().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentTerms5 }
*
*
*/
public List getPmtTerms() {
if (pmtTerms == null) {
pmtTerms = new ArrayList();
}
return this.pmtTerms;
}
/**
* Gets the value of the sttlmTerms property.
*
* @return
* possible object is
* {@link SettlementTerms3 }
*
*/
public SettlementTerms3 getSttlmTerms() {
return sttlmTerms;
}
/**
* Sets the value of the sttlmTerms property.
*
* @param value
* allowed object is
* {@link SettlementTerms3 }
*
*/
public Baseline4 setSttlmTerms(SettlementTerms3 value) {
this.sttlmTerms = value;
return this;
}
/**
* Gets the value of the pmtOblgtn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the pmtOblgtn property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtOblgtn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentObligation2 }
*
*
*/
public List getPmtOblgtn() {
if (pmtOblgtn == null) {
pmtOblgtn = new ArrayList();
}
return this.pmtOblgtn;
}
/**
* Gets the value of the latstMtchDt property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getLatstMtchDt() {
return latstMtchDt;
}
/**
* Sets the value of the latstMtchDt property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public Baseline4 setLatstMtchDt(XMLGregorianCalendar value) {
this.latstMtchDt = value;
return this;
}
/**
* Gets the value of the comrclDataSetReqrd property.
*
* @return
* possible object is
* {@link RequiredSubmission2 }
*
*/
public RequiredSubmission2 getComrclDataSetReqrd() {
return comrclDataSetReqrd;
}
/**
* Sets the value of the comrclDataSetReqrd property.
*
* @param value
* allowed object is
* {@link RequiredSubmission2 }
*
*/
public Baseline4 setComrclDataSetReqrd(RequiredSubmission2 value) {
this.comrclDataSetReqrd = value;
return this;
}
/**
* Gets the value of the trnsprtDataSetReqrd property.
*
* @return
* possible object is
* {@link RequiredSubmission2 }
*
*/
public RequiredSubmission2 getTrnsprtDataSetReqrd() {
return trnsprtDataSetReqrd;
}
/**
* Sets the value of the trnsprtDataSetReqrd property.
*
* @param value
* allowed object is
* {@link RequiredSubmission2 }
*
*/
public Baseline4 setTrnsprtDataSetReqrd(RequiredSubmission2 value) {
this.trnsprtDataSetReqrd = value;
return this;
}
/**
* Gets the value of the insrncDataSetReqrd property.
*
* @return
* possible object is
* {@link RequiredSubmission3 }
*
*/
public RequiredSubmission3 getInsrncDataSetReqrd() {
return insrncDataSetReqrd;
}
/**
* Sets the value of the insrncDataSetReqrd property.
*
* @param value
* allowed object is
* {@link RequiredSubmission3 }
*
*/
public Baseline4 setInsrncDataSetReqrd(RequiredSubmission3 value) {
this.insrncDataSetReqrd = value;
return this;
}
/**
* Gets the value of the certDataSetReqrd property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the certDataSetReqrd property.
*
*
* For example, to add a new item, do as follows:
*
* getCertDataSetReqrd().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RequiredSubmission4 }
*
*
*/
public List getCertDataSetReqrd() {
if (certDataSetReqrd == null) {
certDataSetReqrd = new ArrayList();
}
return this.certDataSetReqrd;
}
/**
* Gets the value of the othrCertDataSetReqrd property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the othrCertDataSetReqrd property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrCertDataSetReqrd().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RequiredSubmission5 }
*
*
*/
public List getOthrCertDataSetReqrd() {
if (othrCertDataSetReqrd == null) {
othrCertDataSetReqrd = new ArrayList();
}
return this.othrCertDataSetReqrd;
}
/**
* Gets the value of the inttToPayXpctd property.
*
*/
public boolean isInttToPayXpctd() {
return inttToPayXpctd;
}
/**
* Sets the value of the inttToPayXpctd property.
*
*/
public Baseline4 setInttToPayXpctd(boolean value) {
this.inttToPayXpctd = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the buyrSdSubmitgBk list.
* @see #getBuyrSdSubmitgBk()
*
*/
public Baseline4 addBuyrSdSubmitgBk(BICIdentification1 buyrSdSubmitgBk) {
getBuyrSdSubmitgBk().add(buyrSdSubmitgBk);
return this;
}
/**
* Adds a new item to the sellrSdSubmitgBk list.
* @see #getSellrSdSubmitgBk()
*
*/
public Baseline4 addSellrSdSubmitgBk(BICIdentification1 sellrSdSubmitgBk) {
getSellrSdSubmitgBk().add(sellrSdSubmitgBk);
return this;
}
/**
* Adds a new item to the pmtTerms list.
* @see #getPmtTerms()
*
*/
public Baseline4 addPmtTerms(PaymentTerms5 pmtTerms) {
getPmtTerms().add(pmtTerms);
return this;
}
/**
* Adds a new item to the pmtOblgtn list.
* @see #getPmtOblgtn()
*
*/
public Baseline4 addPmtOblgtn(PaymentObligation2 pmtOblgtn) {
getPmtOblgtn().add(pmtOblgtn);
return this;
}
/**
* Adds a new item to the certDataSetReqrd list.
* @see #getCertDataSetReqrd()
*
*/
public Baseline4 addCertDataSetReqrd(RequiredSubmission4 certDataSetReqrd) {
getCertDataSetReqrd().add(certDataSetReqrd);
return this;
}
/**
* Adds a new item to the othrCertDataSetReqrd list.
* @see #getOthrCertDataSetReqrd()
*
*/
public Baseline4 addOthrCertDataSetReqrd(RequiredSubmission5 othrCertDataSetReqrd) {
getOthrCertDataSetReqrd().add(othrCertDataSetReqrd);
return this;
}
}