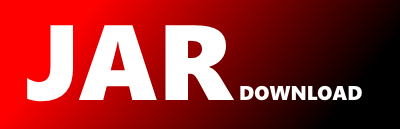
com.prowidesoftware.swift.model.mx.dic.HoldingAsset2 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Information on the assets held by the money market fund.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "HoldingAsset2", propOrder = {
"mnyMktInstrmHldg",
"scrtstnAsstBckdComrclPprHldg",
"derivHldg",
"mnyMktFndHldgInf",
"dpstAncllryLqdAsstHldg",
"rpAgrmtHldg"
})
public class HoldingAsset2 {
@XmlElement(name = "MnyMktInstrmHldg")
protected List mnyMktInstrmHldg;
@XmlElement(name = "ScrtstnAsstBckdComrclPprHldg")
protected List scrtstnAsstBckdComrclPprHldg;
@XmlElement(name = "DerivHldg")
protected List derivHldg;
@XmlElement(name = "MnyMktFndHldgInf")
protected List mnyMktFndHldgInf;
@XmlElement(name = "DpstAncllryLqdAsstHldg")
protected List dpstAncllryLqdAsstHldg;
@XmlElement(name = "RpAgrmtHldg")
protected List rpAgrmtHldg;
/**
* Gets the value of the mnyMktInstrmHldg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the mnyMktInstrmHldg property.
*
*
* For example, to add a new item, do as follows:
*
* getMnyMktInstrmHldg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Financialinstrument78 }
*
*
*/
public List getMnyMktInstrmHldg() {
if (mnyMktInstrmHldg == null) {
mnyMktInstrmHldg = new ArrayList();
}
return this.mnyMktInstrmHldg;
}
/**
* Gets the value of the scrtstnAsstBckdComrclPprHldg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the scrtstnAsstBckdComrclPprHldg property.
*
*
* For example, to add a new item, do as follows:
*
* getScrtstnAsstBckdComrclPprHldg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Financialinstrument78 }
*
*
*/
public List getScrtstnAsstBckdComrclPprHldg() {
if (scrtstnAsstBckdComrclPprHldg == null) {
scrtstnAsstBckdComrclPprHldg = new ArrayList();
}
return this.scrtstnAsstBckdComrclPprHldg;
}
/**
* Gets the value of the derivHldg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the derivHldg property.
*
*
* For example, to add a new item, do as follows:
*
* getDerivHldg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Financialinstrument78 }
*
*
*/
public List getDerivHldg() {
if (derivHldg == null) {
derivHldg = new ArrayList();
}
return this.derivHldg;
}
/**
* Gets the value of the mnyMktFndHldgInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the mnyMktFndHldgInf property.
*
*
* For example, to add a new item, do as follows:
*
* getMnyMktFndHldgInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Financialinstrument78 }
*
*
*/
public List getMnyMktFndHldgInf() {
if (mnyMktFndHldgInf == null) {
mnyMktFndHldgInf = new ArrayList();
}
return this.mnyMktFndHldgInf;
}
/**
* Gets the value of the dpstAncllryLqdAsstHldg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the dpstAncllryLqdAsstHldg property.
*
*
* For example, to add a new item, do as follows:
*
* getDpstAncllryLqdAsstHldg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Financialinstrument78 }
*
*
*/
public List getDpstAncllryLqdAsstHldg() {
if (dpstAncllryLqdAsstHldg == null) {
dpstAncllryLqdAsstHldg = new ArrayList();
}
return this.dpstAncllryLqdAsstHldg;
}
/**
* Gets the value of the rpAgrmtHldg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the rpAgrmtHldg property.
*
*
* For example, to add a new item, do as follows:
*
* getRpAgrmtHldg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Financialinstrument78 }
*
*
*/
public List getRpAgrmtHldg() {
if (rpAgrmtHldg == null) {
rpAgrmtHldg = new ArrayList();
}
return this.rpAgrmtHldg;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the mnyMktInstrmHldg list.
* @see #getMnyMktInstrmHldg()
*
*/
public HoldingAsset2 addMnyMktInstrmHldg(Financialinstrument78 mnyMktInstrmHldg) {
getMnyMktInstrmHldg().add(mnyMktInstrmHldg);
return this;
}
/**
* Adds a new item to the scrtstnAsstBckdComrclPprHldg list.
* @see #getScrtstnAsstBckdComrclPprHldg()
*
*/
public HoldingAsset2 addScrtstnAsstBckdComrclPprHldg(Financialinstrument78 scrtstnAsstBckdComrclPprHldg) {
getScrtstnAsstBckdComrclPprHldg().add(scrtstnAsstBckdComrclPprHldg);
return this;
}
/**
* Adds a new item to the derivHldg list.
* @see #getDerivHldg()
*
*/
public HoldingAsset2 addDerivHldg(Financialinstrument78 derivHldg) {
getDerivHldg().add(derivHldg);
return this;
}
/**
* Adds a new item to the mnyMktFndHldgInf list.
* @see #getMnyMktFndHldgInf()
*
*/
public HoldingAsset2 addMnyMktFndHldgInf(Financialinstrument78 mnyMktFndHldgInf) {
getMnyMktFndHldgInf().add(mnyMktFndHldgInf);
return this;
}
/**
* Adds a new item to the dpstAncllryLqdAsstHldg list.
* @see #getDpstAncllryLqdAsstHldg()
*
*/
public HoldingAsset2 addDpstAncllryLqdAsstHldg(Financialinstrument78 dpstAncllryLqdAsstHldg) {
getDpstAncllryLqdAsstHldg().add(dpstAncllryLqdAsstHldg);
return this;
}
/**
* Adds a new item to the rpAgrmtHldg list.
* @see #getRpAgrmtHldg()
*
*/
public HoldingAsset2 addRpAgrmtHldg(Financialinstrument78 rpAgrmtHldg) {
getRpAgrmtHldg().add(rpAgrmtHldg);
return this;
}
}