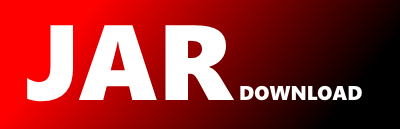
com.prowidesoftware.swift.model.mx.dic.RedemptionBulkOrderV03 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An instructing party, for example, an investment manager or its authorised representative sends the RedemptionBulkOrder message to the executing party, for example, a transfer agent, to instruct a redemption from a financial instrument for two or more accounts.
* Usage
* The RedemptionBulkOrder message is used to bulk several individual orders into one bulk order. The individual orders come from different instructing parties, that is, account owners, but are for the same financial instrument. The RedemptionBulkOrder can result in one single bulk cash settlement or several individual cash settlements.
* This message will be typically used by a party collecting orders, that is, a concentrator, bulking these individual orders into one bulk order before sending it to an executing party.
* For a single redemption order, the RedemptionOrder message, not the RedemptionBulkOrder message, must be used.
* If there are redemption orders for different financial instruments but for the same account, then the RedemptionOrder must be used.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RedemptionBulkOrderV03", propOrder = {
"msgId",
"poolRef",
"prvsRef",
"blkOrdrDtls",
"rltdPtyDtls",
"cpyDtls",
"xtnsn"
})
public class RedemptionBulkOrderV03 {
@XmlElement(name = "MsgId", required = true)
protected MessageIdentification1 msgId;
@XmlElement(name = "PoolRef")
protected AdditionalReference3 poolRef;
@XmlElement(name = "PrvsRef")
protected List prvsRef;
@XmlElement(name = "BlkOrdrDtls", required = true)
protected RedemptionBulkOrder4 blkOrdrDtls;
@XmlElement(name = "RltdPtyDtls")
protected List rltdPtyDtls;
@XmlElement(name = "CpyDtls")
protected CopyInformation2 cpyDtls;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the msgId property.
*
* @return
* possible object is
* {@link MessageIdentification1 }
*
*/
public MessageIdentification1 getMsgId() {
return msgId;
}
/**
* Sets the value of the msgId property.
*
* @param value
* allowed object is
* {@link MessageIdentification1 }
*
*/
public RedemptionBulkOrderV03 setMsgId(MessageIdentification1 value) {
this.msgId = value;
return this;
}
/**
* Gets the value of the poolRef property.
*
* @return
* possible object is
* {@link AdditionalReference3 }
*
*/
public AdditionalReference3 getPoolRef() {
return poolRef;
}
/**
* Sets the value of the poolRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference3 }
*
*/
public RedemptionBulkOrderV03 setPoolRef(AdditionalReference3 value) {
this.poolRef = value;
return this;
}
/**
* Gets the value of the prvsRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the prvsRef property.
*
*
* For example, to add a new item, do as follows:
*
* getPrvsRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalReference3 }
*
*
*/
public List getPrvsRef() {
if (prvsRef == null) {
prvsRef = new ArrayList();
}
return this.prvsRef;
}
/**
* Gets the value of the blkOrdrDtls property.
*
* @return
* possible object is
* {@link RedemptionBulkOrder4 }
*
*/
public RedemptionBulkOrder4 getBlkOrdrDtls() {
return blkOrdrDtls;
}
/**
* Sets the value of the blkOrdrDtls property.
*
* @param value
* allowed object is
* {@link RedemptionBulkOrder4 }
*
*/
public RedemptionBulkOrderV03 setBlkOrdrDtls(RedemptionBulkOrder4 value) {
this.blkOrdrDtls = value;
return this;
}
/**
* Gets the value of the rltdPtyDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the rltdPtyDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getRltdPtyDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary8 }
*
*
*/
public List getRltdPtyDtls() {
if (rltdPtyDtls == null) {
rltdPtyDtls = new ArrayList();
}
return this.rltdPtyDtls;
}
/**
* Gets the value of the cpyDtls property.
*
* @return
* possible object is
* {@link CopyInformation2 }
*
*/
public CopyInformation2 getCpyDtls() {
return cpyDtls;
}
/**
* Sets the value of the cpyDtls property.
*
* @param value
* allowed object is
* {@link CopyInformation2 }
*
*/
public RedemptionBulkOrderV03 setCpyDtls(CopyInformation2 value) {
this.cpyDtls = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the prvsRef list.
* @see #getPrvsRef()
*
*/
public RedemptionBulkOrderV03 addPrvsRef(AdditionalReference3 prvsRef) {
getPrvsRef().add(prvsRef);
return this;
}
/**
* Adds a new item to the rltdPtyDtls list.
* @see #getRltdPtyDtls()
*
*/
public RedemptionBulkOrderV03 addRltdPtyDtls(Intermediary8 rltdPtyDtls) {
getRltdPtyDtls().add(rltdPtyDtls);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public RedemptionBulkOrderV03 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}