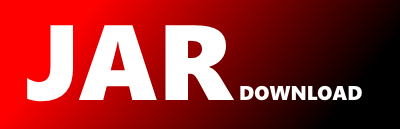
com.prowidesoftware.swift.model.mx.dic.SettlementMonetarySummation1 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Specifies a collection of monetary totals for this settlement.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SettlementMonetarySummation1", propOrder = {
"lineTtlAmt",
"allwncTtlAmt",
"ttlDscntAmt",
"chrgTtlAmt",
"ttlPrepdAmt",
"taxTtlAmt",
"taxBsisAmt",
"rndgAmt",
"grdTtlAmt",
"infAmt"
})
public class SettlementMonetarySummation1 {
@XmlElement(name = "LineTtlAmt")
protected List lineTtlAmt;
@XmlElement(name = "AllwncTtlAmt")
protected List allwncTtlAmt;
@XmlElement(name = "TtlDscntAmt")
protected List ttlDscntAmt;
@XmlElement(name = "ChrgTtlAmt")
protected List chrgTtlAmt;
@XmlElement(name = "TtlPrepdAmt")
protected List ttlPrepdAmt;
@XmlElement(name = "TaxTtlAmt")
protected List taxTtlAmt;
@XmlElement(name = "TaxBsisAmt")
protected List taxBsisAmt;
@XmlElement(name = "RndgAmt")
protected List rndgAmt;
@XmlElement(name = "GrdTtlAmt")
protected List grdTtlAmt;
@XmlElement(name = "InfAmt")
protected List infAmt;
/**
* Gets the value of the lineTtlAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the lineTtlAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getLineTtlAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getLineTtlAmt() {
if (lineTtlAmt == null) {
lineTtlAmt = new ArrayList();
}
return this.lineTtlAmt;
}
/**
* Gets the value of the allwncTtlAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the allwncTtlAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getAllwncTtlAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getAllwncTtlAmt() {
if (allwncTtlAmt == null) {
allwncTtlAmt = new ArrayList();
}
return this.allwncTtlAmt;
}
/**
* Gets the value of the ttlDscntAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ttlDscntAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getTtlDscntAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getTtlDscntAmt() {
if (ttlDscntAmt == null) {
ttlDscntAmt = new ArrayList();
}
return this.ttlDscntAmt;
}
/**
* Gets the value of the chrgTtlAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the chrgTtlAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getChrgTtlAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getChrgTtlAmt() {
if (chrgTtlAmt == null) {
chrgTtlAmt = new ArrayList();
}
return this.chrgTtlAmt;
}
/**
* Gets the value of the ttlPrepdAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ttlPrepdAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getTtlPrepdAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getTtlPrepdAmt() {
if (ttlPrepdAmt == null) {
ttlPrepdAmt = new ArrayList();
}
return this.ttlPrepdAmt;
}
/**
* Gets the value of the taxTtlAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the taxTtlAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getTaxTtlAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getTaxTtlAmt() {
if (taxTtlAmt == null) {
taxTtlAmt = new ArrayList();
}
return this.taxTtlAmt;
}
/**
* Gets the value of the taxBsisAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the taxBsisAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getTaxBsisAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getTaxBsisAmt() {
if (taxBsisAmt == null) {
taxBsisAmt = new ArrayList();
}
return this.taxBsisAmt;
}
/**
* Gets the value of the rndgAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the rndgAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getRndgAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getRndgAmt() {
if (rndgAmt == null) {
rndgAmt = new ArrayList();
}
return this.rndgAmt;
}
/**
* Gets the value of the grdTtlAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the grdTtlAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getGrdTtlAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getGrdTtlAmt() {
if (grdTtlAmt == null) {
grdTtlAmt = new ArrayList();
}
return this.grdTtlAmt;
}
/**
* Gets the value of the infAmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the infAmt property.
*
*
* For example, to add a new item, do as follows:
*
* getInfAmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyAndAmount }
*
*
*/
public List getInfAmt() {
if (infAmt == null) {
infAmt = new ArrayList();
}
return this.infAmt;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the lineTtlAmt list.
* @see #getLineTtlAmt()
*
*/
public SettlementMonetarySummation1 addLineTtlAmt(CurrencyAndAmount lineTtlAmt) {
getLineTtlAmt().add(lineTtlAmt);
return this;
}
/**
* Adds a new item to the allwncTtlAmt list.
* @see #getAllwncTtlAmt()
*
*/
public SettlementMonetarySummation1 addAllwncTtlAmt(CurrencyAndAmount allwncTtlAmt) {
getAllwncTtlAmt().add(allwncTtlAmt);
return this;
}
/**
* Adds a new item to the ttlDscntAmt list.
* @see #getTtlDscntAmt()
*
*/
public SettlementMonetarySummation1 addTtlDscntAmt(CurrencyAndAmount ttlDscntAmt) {
getTtlDscntAmt().add(ttlDscntAmt);
return this;
}
/**
* Adds a new item to the chrgTtlAmt list.
* @see #getChrgTtlAmt()
*
*/
public SettlementMonetarySummation1 addChrgTtlAmt(CurrencyAndAmount chrgTtlAmt) {
getChrgTtlAmt().add(chrgTtlAmt);
return this;
}
/**
* Adds a new item to the ttlPrepdAmt list.
* @see #getTtlPrepdAmt()
*
*/
public SettlementMonetarySummation1 addTtlPrepdAmt(CurrencyAndAmount ttlPrepdAmt) {
getTtlPrepdAmt().add(ttlPrepdAmt);
return this;
}
/**
* Adds a new item to the taxTtlAmt list.
* @see #getTaxTtlAmt()
*
*/
public SettlementMonetarySummation1 addTaxTtlAmt(CurrencyAndAmount taxTtlAmt) {
getTaxTtlAmt().add(taxTtlAmt);
return this;
}
/**
* Adds a new item to the taxBsisAmt list.
* @see #getTaxBsisAmt()
*
*/
public SettlementMonetarySummation1 addTaxBsisAmt(CurrencyAndAmount taxBsisAmt) {
getTaxBsisAmt().add(taxBsisAmt);
return this;
}
/**
* Adds a new item to the rndgAmt list.
* @see #getRndgAmt()
*
*/
public SettlementMonetarySummation1 addRndgAmt(CurrencyAndAmount rndgAmt) {
getRndgAmt().add(rndgAmt);
return this;
}
/**
* Adds a new item to the grdTtlAmt list.
* @see #getGrdTtlAmt()
*
*/
public SettlementMonetarySummation1 addGrdTtlAmt(CurrencyAndAmount grdTtlAmt) {
getGrdTtlAmt().add(grdTtlAmt);
return this;
}
/**
* Adds a new item to the infAmt list.
* @see #getInfAmt()
*
*/
public SettlementMonetarySummation1 addInfAmt(CurrencyAndAmount infAmt) {
getInfAmt().add(infAmt);
return this;
}
}