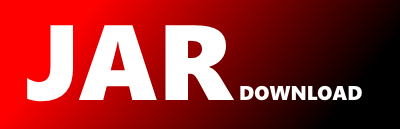
com.prowidesoftware.swift.model.mx.dic.Undertaking3 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Independent undertaking, such as a demand guarantee or standby letter of credit, that provides financial assurance, to be honoured on the presentation of documents that comply with its terms and conditions.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Undertaking3", propOrder = {
"id",
"nm",
"tp",
"issncTp",
"applcnt",
"issr",
"bnfcry",
"dtOfIssnc",
"plcOfIsse",
"advsgPty",
"scndAdvsgPty",
"udrtkgAmt",
"xpryDtls",
"confInd",
"confPtyTp",
"addtlPty",
"govncRulesAndLaw",
"undrlygTx",
"presntnDtls",
"udrtkgTermsAndConds",
"mltplDmndInd",
"prtlDmndInd",
"confChrgsPyblBy",
"trfChrgsPyblBy",
"automtcAmtVartn",
"dlvryChanl",
"trfInd",
"nclsdFile",
"addtlInf",
"reqdLclUdrtkg"
})
public class Undertaking3 {
@XmlElement(name = "Id", required = true)
protected String id;
@XmlElement(name = "Nm", required = true)
@XmlSchemaType(name = "string")
protected UndertakingIssuanceName1Code nm;
@XmlElement(name = "Tp")
protected UndertakingType1Choice tp;
@XmlElement(name = "IssncTp", required = true)
@XmlSchemaType(name = "string")
protected IssuanceType1Code issncTp;
@XmlElement(name = "Applcnt")
protected List applcnt;
@XmlElement(name = "Issr", required = true)
protected PartyIdentification43 issr;
@XmlElement(name = "Bnfcry", required = true)
protected List bnfcry;
@XmlElement(name = "DtOfIssnc", required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar dtOfIssnc;
@XmlElement(name = "PlcOfIsse")
protected PostalAddress12 plcOfIsse;
@XmlElement(name = "AdvsgPty")
protected PartyIdentification43 advsgPty;
@XmlElement(name = "ScndAdvsgPty")
protected PartyIdentification43 scndAdvsgPty;
@XmlElement(name = "UdrtkgAmt", required = true)
protected UndertakingAmount1 udrtkgAmt;
@XmlElement(name = "XpryDtls", required = true)
protected ExpiryDetails1 xpryDtls;
@XmlElement(name = "ConfInd")
protected Boolean confInd;
@XmlElement(name = "ConfPtyTp")
protected String confPtyTp;
@XmlElement(name = "AddtlPty")
protected List addtlPty;
@XmlElement(name = "GovncRulesAndLaw", required = true)
protected GovernanceRules1 govncRulesAndLaw;
@XmlElement(name = "UndrlygTx")
protected List undrlygTx;
@XmlElement(name = "PresntnDtls")
protected Presentation1 presntnDtls;
@XmlElement(name = "UdrtkgTermsAndConds", required = true)
protected List udrtkgTermsAndConds;
@XmlElement(name = "MltplDmndInd")
protected Boolean mltplDmndInd;
@XmlElement(name = "PrtlDmndInd")
protected Boolean prtlDmndInd;
@XmlElement(name = "ConfChrgsPyblBy")
protected String confChrgsPyblBy;
@XmlElement(name = "TrfChrgsPyblBy")
protected String trfChrgsPyblBy;
@XmlElement(name = "AutomtcAmtVartn")
protected List automtcAmtVartn;
@XmlElement(name = "DlvryChanl")
protected CommunicationChannel1 dlvryChanl;
@XmlElement(name = "TrfInd")
protected Boolean trfInd;
@XmlElement(name = "NclsdFile")
protected List nclsdFile;
@XmlElement(name = "AddtlInf")
protected List addtlInf;
@XmlElement(name = "ReqdLclUdrtkg")
protected Undertaking4 reqdLclUdrtkg;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Undertaking3 setId(String value) {
this.id = value;
return this;
}
/**
* Gets the value of the nm property.
*
* @return
* possible object is
* {@link UndertakingIssuanceName1Code }
*
*/
public UndertakingIssuanceName1Code getNm() {
return nm;
}
/**
* Sets the value of the nm property.
*
* @param value
* allowed object is
* {@link UndertakingIssuanceName1Code }
*
*/
public Undertaking3 setNm(UndertakingIssuanceName1Code value) {
this.nm = value;
return this;
}
/**
* Gets the value of the tp property.
*
* @return
* possible object is
* {@link UndertakingType1Choice }
*
*/
public UndertakingType1Choice getTp() {
return tp;
}
/**
* Sets the value of the tp property.
*
* @param value
* allowed object is
* {@link UndertakingType1Choice }
*
*/
public Undertaking3 setTp(UndertakingType1Choice value) {
this.tp = value;
return this;
}
/**
* Gets the value of the issncTp property.
*
* @return
* possible object is
* {@link IssuanceType1Code }
*
*/
public IssuanceType1Code getIssncTp() {
return issncTp;
}
/**
* Sets the value of the issncTp property.
*
* @param value
* allowed object is
* {@link IssuanceType1Code }
*
*/
public Undertaking3 setIssncTp(IssuanceType1Code value) {
this.issncTp = value;
return this;
}
/**
* Gets the value of the applcnt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the applcnt property.
*
*
* For example, to add a new item, do as follows:
*
* getApplcnt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyIdentification43 }
*
*
*/
public List getApplcnt() {
if (applcnt == null) {
applcnt = new ArrayList();
}
return this.applcnt;
}
/**
* Gets the value of the issr property.
*
* @return
* possible object is
* {@link PartyIdentification43 }
*
*/
public PartyIdentification43 getIssr() {
return issr;
}
/**
* Sets the value of the issr property.
*
* @param value
* allowed object is
* {@link PartyIdentification43 }
*
*/
public Undertaking3 setIssr(PartyIdentification43 value) {
this.issr = value;
return this;
}
/**
* Gets the value of the bnfcry property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the bnfcry property.
*
*
* For example, to add a new item, do as follows:
*
* getBnfcry().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyIdentification43 }
*
*
*/
public List getBnfcry() {
if (bnfcry == null) {
bnfcry = new ArrayList();
}
return this.bnfcry;
}
/**
* Gets the value of the dtOfIssnc property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDtOfIssnc() {
return dtOfIssnc;
}
/**
* Sets the value of the dtOfIssnc property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public Undertaking3 setDtOfIssnc(XMLGregorianCalendar value) {
this.dtOfIssnc = value;
return this;
}
/**
* Gets the value of the plcOfIsse property.
*
* @return
* possible object is
* {@link PostalAddress12 }
*
*/
public PostalAddress12 getPlcOfIsse() {
return plcOfIsse;
}
/**
* Sets the value of the plcOfIsse property.
*
* @param value
* allowed object is
* {@link PostalAddress12 }
*
*/
public Undertaking3 setPlcOfIsse(PostalAddress12 value) {
this.plcOfIsse = value;
return this;
}
/**
* Gets the value of the advsgPty property.
*
* @return
* possible object is
* {@link PartyIdentification43 }
*
*/
public PartyIdentification43 getAdvsgPty() {
return advsgPty;
}
/**
* Sets the value of the advsgPty property.
*
* @param value
* allowed object is
* {@link PartyIdentification43 }
*
*/
public Undertaking3 setAdvsgPty(PartyIdentification43 value) {
this.advsgPty = value;
return this;
}
/**
* Gets the value of the scndAdvsgPty property.
*
* @return
* possible object is
* {@link PartyIdentification43 }
*
*/
public PartyIdentification43 getScndAdvsgPty() {
return scndAdvsgPty;
}
/**
* Sets the value of the scndAdvsgPty property.
*
* @param value
* allowed object is
* {@link PartyIdentification43 }
*
*/
public Undertaking3 setScndAdvsgPty(PartyIdentification43 value) {
this.scndAdvsgPty = value;
return this;
}
/**
* Gets the value of the udrtkgAmt property.
*
* @return
* possible object is
* {@link UndertakingAmount1 }
*
*/
public UndertakingAmount1 getUdrtkgAmt() {
return udrtkgAmt;
}
/**
* Sets the value of the udrtkgAmt property.
*
* @param value
* allowed object is
* {@link UndertakingAmount1 }
*
*/
public Undertaking3 setUdrtkgAmt(UndertakingAmount1 value) {
this.udrtkgAmt = value;
return this;
}
/**
* Gets the value of the xpryDtls property.
*
* @return
* possible object is
* {@link ExpiryDetails1 }
*
*/
public ExpiryDetails1 getXpryDtls() {
return xpryDtls;
}
/**
* Sets the value of the xpryDtls property.
*
* @param value
* allowed object is
* {@link ExpiryDetails1 }
*
*/
public Undertaking3 setXpryDtls(ExpiryDetails1 value) {
this.xpryDtls = value;
return this;
}
/**
* Gets the value of the confInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isConfInd() {
return confInd;
}
/**
* Sets the value of the confInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public Undertaking3 setConfInd(Boolean value) {
this.confInd = value;
return this;
}
/**
* Gets the value of the confPtyTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getConfPtyTp() {
return confPtyTp;
}
/**
* Sets the value of the confPtyTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Undertaking3 setConfPtyTp(String value) {
this.confPtyTp = value;
return this;
}
/**
* Gets the value of the addtlPty property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the addtlPty property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlPty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyAndType1 }
*
*
*/
public List getAddtlPty() {
if (addtlPty == null) {
addtlPty = new ArrayList();
}
return this.addtlPty;
}
/**
* Gets the value of the govncRulesAndLaw property.
*
* @return
* possible object is
* {@link GovernanceRules1 }
*
*/
public GovernanceRules1 getGovncRulesAndLaw() {
return govncRulesAndLaw;
}
/**
* Sets the value of the govncRulesAndLaw property.
*
* @param value
* allowed object is
* {@link GovernanceRules1 }
*
*/
public Undertaking3 setGovncRulesAndLaw(GovernanceRules1 value) {
this.govncRulesAndLaw = value;
return this;
}
/**
* Gets the value of the undrlygTx property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the undrlygTx property.
*
*
* For example, to add a new item, do as follows:
*
* getUndrlygTx().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link UnderlyingTradeTransaction1 }
*
*
*/
public List getUndrlygTx() {
if (undrlygTx == null) {
undrlygTx = new ArrayList();
}
return this.undrlygTx;
}
/**
* Gets the value of the presntnDtls property.
*
* @return
* possible object is
* {@link Presentation1 }
*
*/
public Presentation1 getPresntnDtls() {
return presntnDtls;
}
/**
* Sets the value of the presntnDtls property.
*
* @param value
* allowed object is
* {@link Presentation1 }
*
*/
public Undertaking3 setPresntnDtls(Presentation1 value) {
this.presntnDtls = value;
return this;
}
/**
* Gets the value of the udrtkgTermsAndConds property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the udrtkgTermsAndConds property.
*
*
* For example, to add a new item, do as follows:
*
* getUdrtkgTermsAndConds().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Narrative1 }
*
*
*/
public List getUdrtkgTermsAndConds() {
if (udrtkgTermsAndConds == null) {
udrtkgTermsAndConds = new ArrayList();
}
return this.udrtkgTermsAndConds;
}
/**
* Gets the value of the mltplDmndInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isMltplDmndInd() {
return mltplDmndInd;
}
/**
* Sets the value of the mltplDmndInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public Undertaking3 setMltplDmndInd(Boolean value) {
this.mltplDmndInd = value;
return this;
}
/**
* Gets the value of the prtlDmndInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isPrtlDmndInd() {
return prtlDmndInd;
}
/**
* Sets the value of the prtlDmndInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public Undertaking3 setPrtlDmndInd(Boolean value) {
this.prtlDmndInd = value;
return this;
}
/**
* Gets the value of the confChrgsPyblBy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getConfChrgsPyblBy() {
return confChrgsPyblBy;
}
/**
* Sets the value of the confChrgsPyblBy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Undertaking3 setConfChrgsPyblBy(String value) {
this.confChrgsPyblBy = value;
return this;
}
/**
* Gets the value of the trfChrgsPyblBy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTrfChrgsPyblBy() {
return trfChrgsPyblBy;
}
/**
* Sets the value of the trfChrgsPyblBy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Undertaking3 setTrfChrgsPyblBy(String value) {
this.trfChrgsPyblBy = value;
return this;
}
/**
* Gets the value of the automtcAmtVartn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the automtcAmtVartn property.
*
*
* For example, to add a new item, do as follows:
*
* getAutomtcAmtVartn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AutomaticVariation1 }
*
*
*/
public List getAutomtcAmtVartn() {
if (automtcAmtVartn == null) {
automtcAmtVartn = new ArrayList();
}
return this.automtcAmtVartn;
}
/**
* Gets the value of the dlvryChanl property.
*
* @return
* possible object is
* {@link CommunicationChannel1 }
*
*/
public CommunicationChannel1 getDlvryChanl() {
return dlvryChanl;
}
/**
* Sets the value of the dlvryChanl property.
*
* @param value
* allowed object is
* {@link CommunicationChannel1 }
*
*/
public Undertaking3 setDlvryChanl(CommunicationChannel1 value) {
this.dlvryChanl = value;
return this;
}
/**
* Gets the value of the trfInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTrfInd() {
return trfInd;
}
/**
* Sets the value of the trfInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public Undertaking3 setTrfInd(Boolean value) {
this.trfInd = value;
return this;
}
/**
* Gets the value of the nclsdFile property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the nclsdFile property.
*
*
* For example, to add a new item, do as follows:
*
* getNclsdFile().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Document9 }
*
*
*/
public List getNclsdFile() {
if (nclsdFile == null) {
nclsdFile = new ArrayList();
}
return this.nclsdFile;
}
/**
* Gets the value of the addtlInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the addtlInf property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getAddtlInf() {
if (addtlInf == null) {
addtlInf = new ArrayList();
}
return this.addtlInf;
}
/**
* Gets the value of the reqdLclUdrtkg property.
*
* @return
* possible object is
* {@link Undertaking4 }
*
*/
public Undertaking4 getReqdLclUdrtkg() {
return reqdLclUdrtkg;
}
/**
* Sets the value of the reqdLclUdrtkg property.
*
* @param value
* allowed object is
* {@link Undertaking4 }
*
*/
public Undertaking3 setReqdLclUdrtkg(Undertaking4 value) {
this.reqdLclUdrtkg = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the applcnt list.
* @see #getApplcnt()
*
*/
public Undertaking3 addApplcnt(PartyIdentification43 applcnt) {
getApplcnt().add(applcnt);
return this;
}
/**
* Adds a new item to the bnfcry list.
* @see #getBnfcry()
*
*/
public Undertaking3 addBnfcry(PartyIdentification43 bnfcry) {
getBnfcry().add(bnfcry);
return this;
}
/**
* Adds a new item to the addtlPty list.
* @see #getAddtlPty()
*
*/
public Undertaking3 addAddtlPty(PartyAndType1 addtlPty) {
getAddtlPty().add(addtlPty);
return this;
}
/**
* Adds a new item to the undrlygTx list.
* @see #getUndrlygTx()
*
*/
public Undertaking3 addUndrlygTx(UnderlyingTradeTransaction1 undrlygTx) {
getUndrlygTx().add(undrlygTx);
return this;
}
/**
* Adds a new item to the udrtkgTermsAndConds list.
* @see #getUdrtkgTermsAndConds()
*
*/
public Undertaking3 addUdrtkgTermsAndConds(Narrative1 udrtkgTermsAndConds) {
getUdrtkgTermsAndConds().add(udrtkgTermsAndConds);
return this;
}
/**
* Adds a new item to the automtcAmtVartn list.
* @see #getAutomtcAmtVartn()
*
*/
public Undertaking3 addAutomtcAmtVartn(AutomaticVariation1 automtcAmtVartn) {
getAutomtcAmtVartn().add(automtcAmtVartn);
return this;
}
/**
* Adds a new item to the nclsdFile list.
* @see #getNclsdFile()
*
*/
public Undertaking3 addNclsdFile(Document9 nclsdFile) {
getNclsdFile().add(nclsdFile);
return this;
}
/**
* Adds a new item to the addtlInf list.
* @see #getAddtlInf()
*
*/
public Undertaking3 addAddtlInf(String addtlInf) {
getAddtlInf().add(addtlInf);
return this;
}
}