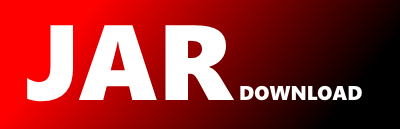
com.prowidesoftware.swift.model.mx.dic.ATMTransaction13 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Withdrawal transaction for which an authorisation is requested.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ATMTransaction13", propOrder = {
"cshDspnsd",
"txId",
"rcncltnId",
"acctData",
"prtctdAcctData",
"ttlReqdAmt",
"dtldReqdAmt",
"ccyConvsRslt",
"selctdMixTp",
"selctdMix",
"reqdRct",
"iccRltdData"
})
public class ATMTransaction13 {
@XmlElement(name = "CshDspnsd")
protected Boolean cshDspnsd;
@XmlElement(name = "TxId", required = true)
protected TransactionIdentifier1 txId;
@XmlElement(name = "RcncltnId")
protected String rcncltnId;
@XmlElement(name = "AcctData")
protected CardAccount7 acctData;
@XmlElement(name = "PrtctdAcctData")
protected ContentInformationType10 prtctdAcctData;
@XmlElement(name = "TtlReqdAmt")
protected AmountAndCurrency1 ttlReqdAmt;
@XmlElement(name = "DtldReqdAmt")
protected DetailedAmount12 dtldReqdAmt;
@XmlElement(name = "CcyConvsRslt")
protected CurrencyConversion9 ccyConvsRslt;
@XmlElement(name = "SelctdMixTp")
protected String selctdMixTp;
@XmlElement(name = "SelctdMix")
protected List selctdMix;
@XmlElement(name = "ReqdRct")
protected Boolean reqdRct;
@XmlElement(name = "ICCRltdData")
protected byte[] iccRltdData;
/**
* Gets the value of the cshDspnsd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCshDspnsd() {
return cshDspnsd;
}
/**
* Sets the value of the cshDspnsd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public ATMTransaction13 setCshDspnsd(Boolean value) {
this.cshDspnsd = value;
return this;
}
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link TransactionIdentifier1 }
*
*/
public TransactionIdentifier1 getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link TransactionIdentifier1 }
*
*/
public ATMTransaction13 setTxId(TransactionIdentifier1 value) {
this.txId = value;
return this;
}
/**
* Gets the value of the rcncltnId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRcncltnId() {
return rcncltnId;
}
/**
* Sets the value of the rcncltnId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ATMTransaction13 setRcncltnId(String value) {
this.rcncltnId = value;
return this;
}
/**
* Gets the value of the acctData property.
*
* @return
* possible object is
* {@link CardAccount7 }
*
*/
public CardAccount7 getAcctData() {
return acctData;
}
/**
* Sets the value of the acctData property.
*
* @param value
* allowed object is
* {@link CardAccount7 }
*
*/
public ATMTransaction13 setAcctData(CardAccount7 value) {
this.acctData = value;
return this;
}
/**
* Gets the value of the prtctdAcctData property.
*
* @return
* possible object is
* {@link ContentInformationType10 }
*
*/
public ContentInformationType10 getPrtctdAcctData() {
return prtctdAcctData;
}
/**
* Sets the value of the prtctdAcctData property.
*
* @param value
* allowed object is
* {@link ContentInformationType10 }
*
*/
public ATMTransaction13 setPrtctdAcctData(ContentInformationType10 value) {
this.prtctdAcctData = value;
return this;
}
/**
* Gets the value of the ttlReqdAmt property.
*
* @return
* possible object is
* {@link AmountAndCurrency1 }
*
*/
public AmountAndCurrency1 getTtlReqdAmt() {
return ttlReqdAmt;
}
/**
* Sets the value of the ttlReqdAmt property.
*
* @param value
* allowed object is
* {@link AmountAndCurrency1 }
*
*/
public ATMTransaction13 setTtlReqdAmt(AmountAndCurrency1 value) {
this.ttlReqdAmt = value;
return this;
}
/**
* Gets the value of the dtldReqdAmt property.
*
* @return
* possible object is
* {@link DetailedAmount12 }
*
*/
public DetailedAmount12 getDtldReqdAmt() {
return dtldReqdAmt;
}
/**
* Sets the value of the dtldReqdAmt property.
*
* @param value
* allowed object is
* {@link DetailedAmount12 }
*
*/
public ATMTransaction13 setDtldReqdAmt(DetailedAmount12 value) {
this.dtldReqdAmt = value;
return this;
}
/**
* Gets the value of the ccyConvsRslt property.
*
* @return
* possible object is
* {@link CurrencyConversion9 }
*
*/
public CurrencyConversion9 getCcyConvsRslt() {
return ccyConvsRslt;
}
/**
* Sets the value of the ccyConvsRslt property.
*
* @param value
* allowed object is
* {@link CurrencyConversion9 }
*
*/
public ATMTransaction13 setCcyConvsRslt(CurrencyConversion9 value) {
this.ccyConvsRslt = value;
return this;
}
/**
* Gets the value of the selctdMixTp property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSelctdMixTp() {
return selctdMixTp;
}
/**
* Sets the value of the selctdMixTp property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public ATMTransaction13 setSelctdMixTp(String value) {
this.selctdMixTp = value;
return this;
}
/**
* Gets the value of the selctdMix property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the selctdMix property.
*
*
* For example, to add a new item, do as follows:
*
* getSelctdMix().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ATMMediaMix1 }
*
*
* @return
* The value of the selctdMix property.
*/
public List getSelctdMix() {
if (selctdMix == null) {
selctdMix = new ArrayList<>();
}
return this.selctdMix;
}
/**
* Gets the value of the reqdRct property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isReqdRct() {
return reqdRct;
}
/**
* Sets the value of the reqdRct property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public ATMTransaction13 setReqdRct(Boolean value) {
this.reqdRct = value;
return this;
}
/**
* Gets the value of the iccRltdData property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getICCRltdData() {
return iccRltdData;
}
/**
* Sets the value of the iccRltdData property.
*
* @param value
* allowed object is
* byte[]
*/
public ATMTransaction13 setICCRltdData(byte[] value) {
this.iccRltdData = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the selctdMix list.
* @see #getSelctdMix()
*
*/
public ATMTransaction13 addSelctdMix(ATMMediaMix1 selctdMix) {
getSelctdMix().add(selctdMix);
return this;
}
}