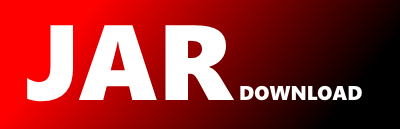
com.prowidesoftware.swift.model.mx.dic.AcceptorConfigurationContent10 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Content of the acceptor configuration.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AcceptorConfigurationContent10", propOrder = {
"rplcCfgtn",
"tmsPrtcolParams",
"acqrrPrtcolParams",
"svcPrvdrParams",
"mrchntParams",
"termnlParams",
"applParams",
"hstComParams",
"sctyParams",
"saleToPOIParams",
"termnlPackg"
})
public class AcceptorConfigurationContent10 {
@XmlElement(name = "RplcCfgtn")
protected Boolean rplcCfgtn;
@XmlElement(name = "TMSPrtcolParams")
protected List tmsPrtcolParams;
@XmlElement(name = "AcqrrPrtcolParams")
protected List acqrrPrtcolParams;
@XmlElement(name = "SvcPrvdrParams")
protected List svcPrvdrParams;
@XmlElement(name = "MrchntParams")
protected List mrchntParams;
@XmlElement(name = "TermnlParams")
protected List termnlParams;
@XmlElement(name = "ApplParams")
protected List applParams;
@XmlElement(name = "HstComParams")
protected List hstComParams;
@XmlElement(name = "SctyParams")
protected List sctyParams;
@XmlElement(name = "SaleToPOIParams")
protected List saleToPOIParams;
@XmlElement(name = "TermnlPackg")
protected List termnlPackg;
/**
* Gets the value of the rplcCfgtn property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRplcCfgtn() {
return rplcCfgtn;
}
/**
* Sets the value of the rplcCfgtn property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public AcceptorConfigurationContent10 setRplcCfgtn(Boolean value) {
this.rplcCfgtn = value;
return this;
}
/**
* Gets the value of the tmsPrtcolParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the tmsPrtcolParams property.
*
*
* For example, to add a new item, do as follows:
*
* getTMSPrtcolParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TMSProtocolParameters5 }
*
*
* @return
* The value of the tmsPrtcolParams property.
*/
public List getTMSPrtcolParams() {
if (tmsPrtcolParams == null) {
tmsPrtcolParams = new ArrayList<>();
}
return this.tmsPrtcolParams;
}
/**
* Gets the value of the acqrrPrtcolParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the acqrrPrtcolParams property.
*
*
* For example, to add a new item, do as follows:
*
* getAcqrrPrtcolParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AcquirerProtocolParameters14 }
*
*
* @return
* The value of the acqrrPrtcolParams property.
*/
public List getAcqrrPrtcolParams() {
if (acqrrPrtcolParams == null) {
acqrrPrtcolParams = new ArrayList<>();
}
return this.acqrrPrtcolParams;
}
/**
* Gets the value of the svcPrvdrParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the svcPrvdrParams property.
*
*
* For example, to add a new item, do as follows:
*
* getSvcPrvdrParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ServiceProviderParameters1 }
*
*
* @return
* The value of the svcPrvdrParams property.
*/
public List getSvcPrvdrParams() {
if (svcPrvdrParams == null) {
svcPrvdrParams = new ArrayList<>();
}
return this.svcPrvdrParams;
}
/**
* Gets the value of the mrchntParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the mrchntParams property.
*
*
* For example, to add a new item, do as follows:
*
* getMrchntParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link MerchantConfigurationParameters6 }
*
*
* @return
* The value of the mrchntParams property.
*/
public List getMrchntParams() {
if (mrchntParams == null) {
mrchntParams = new ArrayList<>();
}
return this.mrchntParams;
}
/**
* Gets the value of the termnlParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the termnlParams property.
*
*
* For example, to add a new item, do as follows:
*
* getTermnlParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentTerminalParameters8 }
*
*
* @return
* The value of the termnlParams property.
*/
public List getTermnlParams() {
if (termnlParams == null) {
termnlParams = new ArrayList<>();
}
return this.termnlParams;
}
/**
* Gets the value of the applParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the applParams property.
*
*
* For example, to add a new item, do as follows:
*
* getApplParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ApplicationParameters10 }
*
*
* @return
* The value of the applParams property.
*/
public List getApplParams() {
if (applParams == null) {
applParams = new ArrayList<>();
}
return this.applParams;
}
/**
* Gets the value of the hstComParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the hstComParams property.
*
*
* For example, to add a new item, do as follows:
*
* getHstComParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HostCommunicationParameter6 }
*
*
* @return
* The value of the hstComParams property.
*/
public List getHstComParams() {
if (hstComParams == null) {
hstComParams = new ArrayList<>();
}
return this.hstComParams;
}
/**
* Gets the value of the sctyParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the sctyParams property.
*
*
* For example, to add a new item, do as follows:
*
* getSctyParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SecurityParameters13 }
*
*
* @return
* The value of the sctyParams property.
*/
public List getSctyParams() {
if (sctyParams == null) {
sctyParams = new ArrayList<>();
}
return this.sctyParams;
}
/**
* Gets the value of the saleToPOIParams property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the saleToPOIParams property.
*
*
* For example, to add a new item, do as follows:
*
* getSaleToPOIParams().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SaleToPOIProtocolParameter1 }
*
*
* @return
* The value of the saleToPOIParams property.
*/
public List getSaleToPOIParams() {
if (saleToPOIParams == null) {
saleToPOIParams = new ArrayList<>();
}
return this.saleToPOIParams;
}
/**
* Gets the value of the termnlPackg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the termnlPackg property.
*
*
* For example, to add a new item, do as follows:
*
* getTermnlPackg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TerminalPackageType2 }
*
*
* @return
* The value of the termnlPackg property.
*/
public List getTermnlPackg() {
if (termnlPackg == null) {
termnlPackg = new ArrayList<>();
}
return this.termnlPackg;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the tMSPrtcolParams list.
* @see #getTMSPrtcolParams()
*
*/
public AcceptorConfigurationContent10 addTMSPrtcolParams(TMSProtocolParameters5 tMSPrtcolParams) {
getTMSPrtcolParams().add(tMSPrtcolParams);
return this;
}
/**
* Adds a new item to the acqrrPrtcolParams list.
* @see #getAcqrrPrtcolParams()
*
*/
public AcceptorConfigurationContent10 addAcqrrPrtcolParams(AcquirerProtocolParameters14 acqrrPrtcolParams) {
getAcqrrPrtcolParams().add(acqrrPrtcolParams);
return this;
}
/**
* Adds a new item to the svcPrvdrParams list.
* @see #getSvcPrvdrParams()
*
*/
public AcceptorConfigurationContent10 addSvcPrvdrParams(ServiceProviderParameters1 svcPrvdrParams) {
getSvcPrvdrParams().add(svcPrvdrParams);
return this;
}
/**
* Adds a new item to the mrchntParams list.
* @see #getMrchntParams()
*
*/
public AcceptorConfigurationContent10 addMrchntParams(MerchantConfigurationParameters6 mrchntParams) {
getMrchntParams().add(mrchntParams);
return this;
}
/**
* Adds a new item to the termnlParams list.
* @see #getTermnlParams()
*
*/
public AcceptorConfigurationContent10 addTermnlParams(PaymentTerminalParameters8 termnlParams) {
getTermnlParams().add(termnlParams);
return this;
}
/**
* Adds a new item to the applParams list.
* @see #getApplParams()
*
*/
public AcceptorConfigurationContent10 addApplParams(ApplicationParameters10 applParams) {
getApplParams().add(applParams);
return this;
}
/**
* Adds a new item to the hstComParams list.
* @see #getHstComParams()
*
*/
public AcceptorConfigurationContent10 addHstComParams(HostCommunicationParameter6 hstComParams) {
getHstComParams().add(hstComParams);
return this;
}
/**
* Adds a new item to the sctyParams list.
* @see #getSctyParams()
*
*/
public AcceptorConfigurationContent10 addSctyParams(SecurityParameters13 sctyParams) {
getSctyParams().add(sctyParams);
return this;
}
/**
* Adds a new item to the saleToPOIParams list.
* @see #getSaleToPOIParams()
*
*/
public AcceptorConfigurationContent10 addSaleToPOIParams(SaleToPOIProtocolParameter1 saleToPOIParams) {
getSaleToPOIParams().add(saleToPOIParams);
return this;
}
/**
* Adds a new item to the termnlPackg list.
* @see #getTermnlPackg()
*
*/
public AcceptorConfigurationContent10 addTermnlPackg(TerminalPackageType2 termnlPackg) {
getTermnlPackg().add(termnlPackg);
return this;
}
}