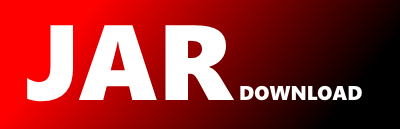
com.prowidesoftware.swift.model.mx.dic.AccountParties6 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Party related to an investment account.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AccountParties6", propOrder = {
"prncplAcctPty",
"scndryOwnr",
"bnfcry",
"pwrOfAttny",
"lglGuardn",
"sucssrOnDth",
"admstr",
"othrPty",
"grntr",
"sttlr"
})
public class AccountParties6 {
@XmlElement(name = "PrncplAcctPty", required = true)
protected AccountParties1Choice prncplAcctPty;
@XmlElement(name = "ScndryOwnr")
protected List scndryOwnr;
@XmlElement(name = "Bnfcry")
protected List bnfcry;
@XmlElement(name = "PwrOfAttny")
protected List pwrOfAttny;
@XmlElement(name = "LglGuardn")
protected List lglGuardn;
@XmlElement(name = "SucssrOnDth")
protected List sucssrOnDth;
@XmlElement(name = "Admstr")
protected InvestmentAccountOwnershipInformation6 admstr;
@XmlElement(name = "OthrPty")
protected List othrPty;
@XmlElement(name = "Grntr")
protected List grntr;
@XmlElement(name = "Sttlr")
protected List sttlr;
/**
* Gets the value of the prncplAcctPty property.
*
* @return
* possible object is
* {@link AccountParties1Choice }
*
*/
public AccountParties1Choice getPrncplAcctPty() {
return prncplAcctPty;
}
/**
* Sets the value of the prncplAcctPty property.
*
* @param value
* allowed object is
* {@link AccountParties1Choice }
*
*/
public AccountParties6 setPrncplAcctPty(AccountParties1Choice value) {
this.prncplAcctPty = value;
return this;
}
/**
* Gets the value of the scndryOwnr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the scndryOwnr property.
*
*
* For example, to add a new item, do as follows:
*
* getScndryOwnr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentAccountOwnershipInformation6 }
*
*
* @return
* The value of the scndryOwnr property.
*/
public List getScndryOwnr() {
if (scndryOwnr == null) {
scndryOwnr = new ArrayList<>();
}
return this.scndryOwnr;
}
/**
* Gets the value of the bnfcry property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the bnfcry property.
*
*
* For example, to add a new item, do as follows:
*
* getBnfcry().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentAccountOwnershipInformation6 }
*
*
* @return
* The value of the bnfcry property.
*/
public List getBnfcry() {
if (bnfcry == null) {
bnfcry = new ArrayList<>();
}
return this.bnfcry;
}
/**
* Gets the value of the pwrOfAttny property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pwrOfAttny property.
*
*
* For example, to add a new item, do as follows:
*
* getPwrOfAttny().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentAccountOwnershipInformation6 }
*
*
* @return
* The value of the pwrOfAttny property.
*/
public List getPwrOfAttny() {
if (pwrOfAttny == null) {
pwrOfAttny = new ArrayList<>();
}
return this.pwrOfAttny;
}
/**
* Gets the value of the lglGuardn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the lglGuardn property.
*
*
* For example, to add a new item, do as follows:
*
* getLglGuardn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentAccountOwnershipInformation6 }
*
*
* @return
* The value of the lglGuardn property.
*/
public List getLglGuardn() {
if (lglGuardn == null) {
lglGuardn = new ArrayList<>();
}
return this.lglGuardn;
}
/**
* Gets the value of the sucssrOnDth property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the sucssrOnDth property.
*
*
* For example, to add a new item, do as follows:
*
* getSucssrOnDth().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentAccountOwnershipInformation6 }
*
*
* @return
* The value of the sucssrOnDth property.
*/
public List getSucssrOnDth() {
if (sucssrOnDth == null) {
sucssrOnDth = new ArrayList<>();
}
return this.sucssrOnDth;
}
/**
* Gets the value of the admstr property.
*
* @return
* possible object is
* {@link InvestmentAccountOwnershipInformation6 }
*
*/
public InvestmentAccountOwnershipInformation6 getAdmstr() {
return admstr;
}
/**
* Sets the value of the admstr property.
*
* @param value
* allowed object is
* {@link InvestmentAccountOwnershipInformation6 }
*
*/
public AccountParties6 setAdmstr(InvestmentAccountOwnershipInformation6 value) {
this.admstr = value;
return this;
}
/**
* Gets the value of the othrPty property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrPty property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrPty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ExtendedParty3 }
*
*
* @return
* The value of the othrPty property.
*/
public List getOthrPty() {
if (othrPty == null) {
othrPty = new ArrayList<>();
}
return this.othrPty;
}
/**
* Gets the value of the grntr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the grntr property.
*
*
* For example, to add a new item, do as follows:
*
* getGrntr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentAccountOwnershipInformation6 }
*
*
* @return
* The value of the grntr property.
*/
public List getGrntr() {
if (grntr == null) {
grntr = new ArrayList<>();
}
return this.grntr;
}
/**
* Gets the value of the sttlr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the sttlr property.
*
*
* For example, to add a new item, do as follows:
*
* getSttlr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link InvestmentAccountOwnershipInformation6 }
*
*
* @return
* The value of the sttlr property.
*/
public List getSttlr() {
if (sttlr == null) {
sttlr = new ArrayList<>();
}
return this.sttlr;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the scndryOwnr list.
* @see #getScndryOwnr()
*
*/
public AccountParties6 addScndryOwnr(InvestmentAccountOwnershipInformation6 scndryOwnr) {
getScndryOwnr().add(scndryOwnr);
return this;
}
/**
* Adds a new item to the bnfcry list.
* @see #getBnfcry()
*
*/
public AccountParties6 addBnfcry(InvestmentAccountOwnershipInformation6 bnfcry) {
getBnfcry().add(bnfcry);
return this;
}
/**
* Adds a new item to the pwrOfAttny list.
* @see #getPwrOfAttny()
*
*/
public AccountParties6 addPwrOfAttny(InvestmentAccountOwnershipInformation6 pwrOfAttny) {
getPwrOfAttny().add(pwrOfAttny);
return this;
}
/**
* Adds a new item to the lglGuardn list.
* @see #getLglGuardn()
*
*/
public AccountParties6 addLglGuardn(InvestmentAccountOwnershipInformation6 lglGuardn) {
getLglGuardn().add(lglGuardn);
return this;
}
/**
* Adds a new item to the sucssrOnDth list.
* @see #getSucssrOnDth()
*
*/
public AccountParties6 addSucssrOnDth(InvestmentAccountOwnershipInformation6 sucssrOnDth) {
getSucssrOnDth().add(sucssrOnDth);
return this;
}
/**
* Adds a new item to the othrPty list.
* @see #getOthrPty()
*
*/
public AccountParties6 addOthrPty(ExtendedParty3 othrPty) {
getOthrPty().add(othrPty);
return this;
}
/**
* Adds a new item to the grntr list.
* @see #getGrntr()
*
*/
public AccountParties6 addGrntr(InvestmentAccountOwnershipInformation6 grntr) {
getGrntr().add(grntr);
return this;
}
/**
* Adds a new item to the sttlr list.
* @see #getSttlr()
*
*/
public AccountParties6 addSttlr(InvestmentAccountOwnershipInformation6 sttlr) {
getSttlr().add(sttlr);
return this;
}
}