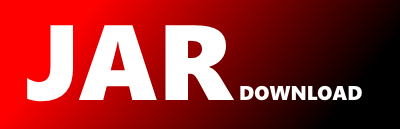
com.prowidesoftware.swift.model.mx.dic.AccountReport12 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Provides further details of the account report.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AccountReport12", propOrder = {
"id",
"rptPgntn",
"elctrncSeqNb",
"lglSeqNb",
"creDtTm",
"frToDt",
"cpyDplctInd",
"rptgSrc",
"acct",
"rltdAcct",
"intrst",
"bal",
"txsSummry",
"ntry",
"addtlRptInf"
})
public class AccountReport12 {
@XmlElement(name = "Id", required = true)
protected String id;
@XmlElement(name = "RptPgntn")
protected Pagination rptPgntn;
@XmlElement(name = "ElctrncSeqNb")
protected BigDecimal elctrncSeqNb;
@XmlElement(name = "LglSeqNb")
protected BigDecimal lglSeqNb;
@XmlElement(name = "CreDtTm", required = true, type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime creDtTm;
@XmlElement(name = "FrToDt")
protected DateTimePeriodDetails frToDt;
@XmlElement(name = "CpyDplctInd")
@XmlSchemaType(name = "string")
protected CopyDuplicate1Code cpyDplctInd;
@XmlElement(name = "RptgSrc")
protected ReportingSource1Choice rptgSrc;
@XmlElement(name = "Acct", required = true)
protected CashAccount25 acct;
@XmlElement(name = "RltdAcct")
protected CashAccount24 rltdAcct;
@XmlElement(name = "Intrst")
protected List intrst;
@XmlElement(name = "Bal")
protected List bal;
@XmlElement(name = "TxsSummry")
protected TotalTransactions3 txsSummry;
@XmlElement(name = "Ntry")
protected List ntry;
@XmlElement(name = "AddtlRptInf")
protected String addtlRptInf;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public AccountReport12 setId(String value) {
this.id = value;
return this;
}
/**
* Gets the value of the rptPgntn property.
*
* @return
* possible object is
* {@link Pagination }
*
*/
public Pagination getRptPgntn() {
return rptPgntn;
}
/**
* Sets the value of the rptPgntn property.
*
* @param value
* allowed object is
* {@link Pagination }
*
*/
public AccountReport12 setRptPgntn(Pagination value) {
this.rptPgntn = value;
return this;
}
/**
* Gets the value of the elctrncSeqNb property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getElctrncSeqNb() {
return elctrncSeqNb;
}
/**
* Sets the value of the elctrncSeqNb property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public AccountReport12 setElctrncSeqNb(BigDecimal value) {
this.elctrncSeqNb = value;
return this;
}
/**
* Gets the value of the lglSeqNb property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getLglSeqNb() {
return lglSeqNb;
}
/**
* Sets the value of the lglSeqNb property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public AccountReport12 setLglSeqNb(BigDecimal value) {
this.lglSeqNb = value;
return this;
}
/**
* Gets the value of the creDtTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getCreDtTm() {
return creDtTm;
}
/**
* Sets the value of the creDtTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public AccountReport12 setCreDtTm(OffsetDateTime value) {
this.creDtTm = value;
return this;
}
/**
* Gets the value of the frToDt property.
*
* @return
* possible object is
* {@link DateTimePeriodDetails }
*
*/
public DateTimePeriodDetails getFrToDt() {
return frToDt;
}
/**
* Sets the value of the frToDt property.
*
* @param value
* allowed object is
* {@link DateTimePeriodDetails }
*
*/
public AccountReport12 setFrToDt(DateTimePeriodDetails value) {
this.frToDt = value;
return this;
}
/**
* Gets the value of the cpyDplctInd property.
*
* @return
* possible object is
* {@link CopyDuplicate1Code }
*
*/
public CopyDuplicate1Code getCpyDplctInd() {
return cpyDplctInd;
}
/**
* Sets the value of the cpyDplctInd property.
*
* @param value
* allowed object is
* {@link CopyDuplicate1Code }
*
*/
public AccountReport12 setCpyDplctInd(CopyDuplicate1Code value) {
this.cpyDplctInd = value;
return this;
}
/**
* Gets the value of the rptgSrc property.
*
* @return
* possible object is
* {@link ReportingSource1Choice }
*
*/
public ReportingSource1Choice getRptgSrc() {
return rptgSrc;
}
/**
* Sets the value of the rptgSrc property.
*
* @param value
* allowed object is
* {@link ReportingSource1Choice }
*
*/
public AccountReport12 setRptgSrc(ReportingSource1Choice value) {
this.rptgSrc = value;
return this;
}
/**
* Gets the value of the acct property.
*
* @return
* possible object is
* {@link CashAccount25 }
*
*/
public CashAccount25 getAcct() {
return acct;
}
/**
* Sets the value of the acct property.
*
* @param value
* allowed object is
* {@link CashAccount25 }
*
*/
public AccountReport12 setAcct(CashAccount25 value) {
this.acct = value;
return this;
}
/**
* Gets the value of the rltdAcct property.
*
* @return
* possible object is
* {@link CashAccount24 }
*
*/
public CashAccount24 getRltdAcct() {
return rltdAcct;
}
/**
* Sets the value of the rltdAcct property.
*
* @param value
* allowed object is
* {@link CashAccount24 }
*
*/
public AccountReport12 setRltdAcct(CashAccount24 value) {
this.rltdAcct = value;
return this;
}
/**
* Gets the value of the intrst property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the intrst property.
*
*
* For example, to add a new item, do as follows:
*
* getIntrst().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AccountInterest3 }
*
*
* @return
* The value of the intrst property.
*/
public List getIntrst() {
if (intrst == null) {
intrst = new ArrayList<>();
}
return this.intrst;
}
/**
* Gets the value of the bal property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the bal property.
*
*
* For example, to add a new item, do as follows:
*
* getBal().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CashBalance3 }
*
*
* @return
* The value of the bal property.
*/
public List getBal() {
if (bal == null) {
bal = new ArrayList<>();
}
return this.bal;
}
/**
* Gets the value of the txsSummry property.
*
* @return
* possible object is
* {@link TotalTransactions3 }
*
*/
public TotalTransactions3 getTxsSummry() {
return txsSummry;
}
/**
* Sets the value of the txsSummry property.
*
* @param value
* allowed object is
* {@link TotalTransactions3 }
*
*/
public AccountReport12 setTxsSummry(TotalTransactions3 value) {
this.txsSummry = value;
return this;
}
/**
* Gets the value of the ntry property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the ntry property.
*
*
* For example, to add a new item, do as follows:
*
* getNtry().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ReportEntry3 }
*
*
* @return
* The value of the ntry property.
*/
public List getNtry() {
if (ntry == null) {
ntry = new ArrayList<>();
}
return this.ntry;
}
/**
* Gets the value of the addtlRptInf property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAddtlRptInf() {
return addtlRptInf;
}
/**
* Sets the value of the addtlRptInf property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public AccountReport12 setAddtlRptInf(String value) {
this.addtlRptInf = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the intrst list.
* @see #getIntrst()
*
*/
public AccountReport12 addIntrst(AccountInterest3 intrst) {
getIntrst().add(intrst);
return this;
}
/**
* Adds a new item to the bal list.
* @see #getBal()
*
*/
public AccountReport12 addBal(CashBalance3 bal) {
getBal().add(bal);
return this;
}
/**
* Adds a new item to the ntry list.
* @see #getNtry()
*
*/
public AccountReport12 addNtry(ReportEntry3 ntry) {
getNtry().add(ntry);
return this;
}
}