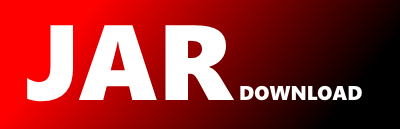
com.prowidesoftware.swift.model.mx.dic.AccountingStatementOfHoldings1 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Information about an accounting statement of holdings.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AccountingStatementOfHoldings1", propOrder = {
"msgPgntn",
"stmtGnlDtls",
"acctDtls",
"balForAcct",
"subAcctDtls",
"ttlVals",
"xtnsn"
})
public class AccountingStatementOfHoldings1 {
@XmlElement(name = "MsgPgntn", required = true)
protected Pagination msgPgntn;
@XmlElement(name = "StmtGnlDtls")
protected Statement4 stmtGnlDtls;
@XmlElement(name = "AcctDtls")
protected SafekeepingAccount1 acctDtls;
@XmlElement(name = "BalForAcct")
protected List balForAcct;
@XmlElement(name = "SubAcctDtls")
protected List subAcctDtls;
@XmlElement(name = "TtlVals")
protected TotalValueInPageAndStatement ttlVals;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the msgPgntn property.
*
* @return
* possible object is
* {@link Pagination }
*
*/
public Pagination getMsgPgntn() {
return msgPgntn;
}
/**
* Sets the value of the msgPgntn property.
*
* @param value
* allowed object is
* {@link Pagination }
*
*/
public AccountingStatementOfHoldings1 setMsgPgntn(Pagination value) {
this.msgPgntn = value;
return this;
}
/**
* Gets the value of the stmtGnlDtls property.
*
* @return
* possible object is
* {@link Statement4 }
*
*/
public Statement4 getStmtGnlDtls() {
return stmtGnlDtls;
}
/**
* Sets the value of the stmtGnlDtls property.
*
* @param value
* allowed object is
* {@link Statement4 }
*
*/
public AccountingStatementOfHoldings1 setStmtGnlDtls(Statement4 value) {
this.stmtGnlDtls = value;
return this;
}
/**
* Gets the value of the acctDtls property.
*
* @return
* possible object is
* {@link SafekeepingAccount1 }
*
*/
public SafekeepingAccount1 getAcctDtls() {
return acctDtls;
}
/**
* Sets the value of the acctDtls property.
*
* @param value
* allowed object is
* {@link SafekeepingAccount1 }
*
*/
public AccountingStatementOfHoldings1 setAcctDtls(SafekeepingAccount1 value) {
this.acctDtls = value;
return this;
}
/**
* Gets the value of the balForAcct property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the balForAcct property.
*
*
* For example, to add a new item, do as follows:
*
* getBalForAcct().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AggregateBalanceInformation2 }
*
*
* @return
* The value of the balForAcct property.
*/
public List getBalForAcct() {
if (balForAcct == null) {
balForAcct = new ArrayList<>();
}
return this.balForAcct;
}
/**
* Gets the value of the subAcctDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the subAcctDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getSubAcctDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SubAccountIdentification2 }
*
*
* @return
* The value of the subAcctDtls property.
*/
public List getSubAcctDtls() {
if (subAcctDtls == null) {
subAcctDtls = new ArrayList<>();
}
return this.subAcctDtls;
}
/**
* Gets the value of the ttlVals property.
*
* @return
* possible object is
* {@link TotalValueInPageAndStatement }
*
*/
public TotalValueInPageAndStatement getTtlVals() {
return ttlVals;
}
/**
* Sets the value of the ttlVals property.
*
* @param value
* allowed object is
* {@link TotalValueInPageAndStatement }
*
*/
public AccountingStatementOfHoldings1 setTtlVals(TotalValueInPageAndStatement value) {
this.ttlVals = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the balForAcct list.
* @see #getBalForAcct()
*
*/
public AccountingStatementOfHoldings1 addBalForAcct(AggregateBalanceInformation2 balForAcct) {
getBalForAcct().add(balForAcct);
return this;
}
/**
* Adds a new item to the subAcctDtls list.
* @see #getSubAcctDtls()
*
*/
public AccountingStatementOfHoldings1 addSubAcctDtls(SubAccountIdentification2 subAcctDtls) {
getSubAcctDtls().add(subAcctDtls);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public AccountingStatementOfHoldings1 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}