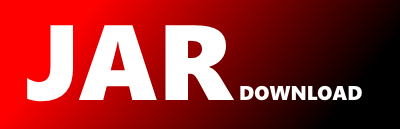
com.prowidesoftware.swift.model.mx.dic.AggregateBalanceInformation3 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Net position of a segregated holding, in a single security, within the overall position held in a securities account. A securities balance is calculated from the sum of securities' receipts minus the sum of securities' deliveries.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AggregateBalanceInformation3", propOrder = {
"aggtQty",
"daysAcrd",
"hldgVal",
"prvsHldgVal",
"acrdIntrstAmt",
"acrdIntrstAmtSgn",
"bookVal",
"sfkpgPlc",
"finInstrmDtls",
"pricDtls",
"fxDtls",
"balBrkdwnDtls",
"addtlBalBrkdwnDtls",
"balAtSfkpgPlc"
})
public class AggregateBalanceInformation3 {
@XmlElement(name = "AggtQty", required = true)
protected BalanceQuantity1Choice aggtQty;
@XmlElement(name = "DaysAcrd")
protected BigDecimal daysAcrd;
@XmlElement(name = "HldgVal", required = true)
protected List hldgVal;
@XmlElement(name = "PrvsHldgVal")
protected ActiveOrHistoricCurrencyAndAmount prvsHldgVal;
@XmlElement(name = "AcrdIntrstAmt")
protected ActiveOrHistoricCurrencyAndAmount acrdIntrstAmt;
@XmlElement(name = "AcrdIntrstAmtSgn")
protected Boolean acrdIntrstAmtSgn;
@XmlElement(name = "BookVal")
protected ActiveOrHistoricCurrencyAndAmount bookVal;
@XmlElement(name = "SfkpgPlc")
protected SafekeepingPlaceFormatChoice sfkpgPlc;
@XmlElement(name = "FinInstrmDtls", required = true)
protected FinancialInstrument13 finInstrmDtls;
@XmlElement(name = "PricDtls", required = true)
protected List pricDtls;
@XmlElement(name = "FXDtls")
protected ForeignExchangeTerms6 fxDtls;
@XmlElement(name = "BalBrkdwnDtls")
protected List balBrkdwnDtls;
@XmlElement(name = "AddtlBalBrkdwnDtls")
protected List addtlBalBrkdwnDtls;
@XmlElement(name = "BalAtSfkpgPlc")
protected List balAtSfkpgPlc;
/**
* Gets the value of the aggtQty property.
*
* @return
* possible object is
* {@link BalanceQuantity1Choice }
*
*/
public BalanceQuantity1Choice getAggtQty() {
return aggtQty;
}
/**
* Sets the value of the aggtQty property.
*
* @param value
* allowed object is
* {@link BalanceQuantity1Choice }
*
*/
public AggregateBalanceInformation3 setAggtQty(BalanceQuantity1Choice value) {
this.aggtQty = value;
return this;
}
/**
* Gets the value of the daysAcrd property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getDaysAcrd() {
return daysAcrd;
}
/**
* Sets the value of the daysAcrd property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public AggregateBalanceInformation3 setDaysAcrd(BigDecimal value) {
this.daysAcrd = value;
return this;
}
/**
* Gets the value of the hldgVal property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the hldgVal property.
*
*
* For example, to add a new item, do as follows:
*
* getHldgVal().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*
* @return
* The value of the hldgVal property.
*/
public List getHldgVal() {
if (hldgVal == null) {
hldgVal = new ArrayList<>();
}
return this.hldgVal;
}
/**
* Gets the value of the prvsHldgVal property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getPrvsHldgVal() {
return prvsHldgVal;
}
/**
* Sets the value of the prvsHldgVal property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public AggregateBalanceInformation3 setPrvsHldgVal(ActiveOrHistoricCurrencyAndAmount value) {
this.prvsHldgVal = value;
return this;
}
/**
* Gets the value of the acrdIntrstAmt property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getAcrdIntrstAmt() {
return acrdIntrstAmt;
}
/**
* Sets the value of the acrdIntrstAmt property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public AggregateBalanceInformation3 setAcrdIntrstAmt(ActiveOrHistoricCurrencyAndAmount value) {
this.acrdIntrstAmt = value;
return this;
}
/**
* Gets the value of the acrdIntrstAmtSgn property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAcrdIntrstAmtSgn() {
return acrdIntrstAmtSgn;
}
/**
* Sets the value of the acrdIntrstAmtSgn property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public AggregateBalanceInformation3 setAcrdIntrstAmtSgn(Boolean value) {
this.acrdIntrstAmtSgn = value;
return this;
}
/**
* Gets the value of the bookVal property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getBookVal() {
return bookVal;
}
/**
* Sets the value of the bookVal property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public AggregateBalanceInformation3 setBookVal(ActiveOrHistoricCurrencyAndAmount value) {
this.bookVal = value;
return this;
}
/**
* Gets the value of the sfkpgPlc property.
*
* @return
* possible object is
* {@link SafekeepingPlaceFormatChoice }
*
*/
public SafekeepingPlaceFormatChoice getSfkpgPlc() {
return sfkpgPlc;
}
/**
* Sets the value of the sfkpgPlc property.
*
* @param value
* allowed object is
* {@link SafekeepingPlaceFormatChoice }
*
*/
public AggregateBalanceInformation3 setSfkpgPlc(SafekeepingPlaceFormatChoice value) {
this.sfkpgPlc = value;
return this;
}
/**
* Gets the value of the finInstrmDtls property.
*
* @return
* possible object is
* {@link FinancialInstrument13 }
*
*/
public FinancialInstrument13 getFinInstrmDtls() {
return finInstrmDtls;
}
/**
* Sets the value of the finInstrmDtls property.
*
* @param value
* allowed object is
* {@link FinancialInstrument13 }
*
*/
public AggregateBalanceInformation3 setFinInstrmDtls(FinancialInstrument13 value) {
this.finInstrmDtls = value;
return this;
}
/**
* Gets the value of the pricDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pricDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getPricDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PriceInformation2 }
*
*
* @return
* The value of the pricDtls property.
*/
public List getPricDtls() {
if (pricDtls == null) {
pricDtls = new ArrayList<>();
}
return this.pricDtls;
}
/**
* Gets the value of the fxDtls property.
*
* @return
* possible object is
* {@link ForeignExchangeTerms6 }
*
*/
public ForeignExchangeTerms6 getFXDtls() {
return fxDtls;
}
/**
* Sets the value of the fxDtls property.
*
* @param value
* allowed object is
* {@link ForeignExchangeTerms6 }
*
*/
public AggregateBalanceInformation3 setFXDtls(ForeignExchangeTerms6 value) {
this.fxDtls = value;
return this;
}
/**
* Gets the value of the balBrkdwnDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the balBrkdwnDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getBalBrkdwnDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SubBalanceInformation2 }
*
*
* @return
* The value of the balBrkdwnDtls property.
*/
public List getBalBrkdwnDtls() {
if (balBrkdwnDtls == null) {
balBrkdwnDtls = new ArrayList<>();
}
return this.balBrkdwnDtls;
}
/**
* Gets the value of the addtlBalBrkdwnDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlBalBrkdwnDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlBalBrkdwnDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalBalanceInformation2 }
*
*
* @return
* The value of the addtlBalBrkdwnDtls property.
*/
public List getAddtlBalBrkdwnDtls() {
if (addtlBalBrkdwnDtls == null) {
addtlBalBrkdwnDtls = new ArrayList<>();
}
return this.addtlBalBrkdwnDtls;
}
/**
* Gets the value of the balAtSfkpgPlc property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the balAtSfkpgPlc property.
*
*
* For example, to add a new item, do as follows:
*
* getBalAtSfkpgPlc().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AggregateBalancePerSafekeepingPlace4 }
*
*
* @return
* The value of the balAtSfkpgPlc property.
*/
public List getBalAtSfkpgPlc() {
if (balAtSfkpgPlc == null) {
balAtSfkpgPlc = new ArrayList<>();
}
return this.balAtSfkpgPlc;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the hldgVal list.
* @see #getHldgVal()
*
*/
public AggregateBalanceInformation3 addHldgVal(ActiveOrHistoricCurrencyAndAmount hldgVal) {
getHldgVal().add(hldgVal);
return this;
}
/**
* Adds a new item to the pricDtls list.
* @see #getPricDtls()
*
*/
public AggregateBalanceInformation3 addPricDtls(PriceInformation2 pricDtls) {
getPricDtls().add(pricDtls);
return this;
}
/**
* Adds a new item to the balBrkdwnDtls list.
* @see #getBalBrkdwnDtls()
*
*/
public AggregateBalanceInformation3 addBalBrkdwnDtls(SubBalanceInformation2 balBrkdwnDtls) {
getBalBrkdwnDtls().add(balBrkdwnDtls);
return this;
}
/**
* Adds a new item to the addtlBalBrkdwnDtls list.
* @see #getAddtlBalBrkdwnDtls()
*
*/
public AggregateBalanceInformation3 addAddtlBalBrkdwnDtls(AdditionalBalanceInformation2 addtlBalBrkdwnDtls) {
getAddtlBalBrkdwnDtls().add(addtlBalBrkdwnDtls);
return this;
}
/**
* Adds a new item to the balAtSfkpgPlc list.
* @see #getBalAtSfkpgPlc()
*
*/
public AggregateBalanceInformation3 addBalAtSfkpgPlc(AggregateBalancePerSafekeepingPlace4 balAtSfkpgPlc) {
getBalAtSfkpgPlc().add(balAtSfkpgPlc);
return this;
}
}