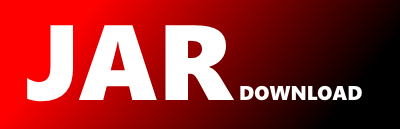
com.prowidesoftware.swift.model.mx.dic.AggregateBalancePerSafekeepingPlace24 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Net position of a segregated holding, in a single security, within the overall position held in a securities account at a specified place of safekeeping.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AggregateBalancePerSafekeepingPlace24", propOrder = {
"sfkpgPlc",
"plcOfListg",
"pldgee",
"aggtBal",
"avlblBal",
"notAvlblBal",
"pricDtls",
"fxDtls",
"daysAcrd",
"acctBaseCcyAmts",
"instrmCcyAmts",
"qtyBrkdwn",
"xpsrTp",
"balBrkdwn",
"addtlBalBrkdwn",
"hldgAddtlDtls"
})
public class AggregateBalancePerSafekeepingPlace24 {
@XmlElement(name = "SfkpgPlc", required = true)
protected SafekeepingPlaceFormat3Choice sfkpgPlc;
@XmlElement(name = "PlcOfListg")
protected MarketIdentification3Choice plcOfListg;
@XmlElement(name = "Pldgee")
protected PledgeeFormat1Choice pldgee;
@XmlElement(name = "AggtBal", required = true)
protected Balance1 aggtBal;
@XmlElement(name = "AvlblBal")
protected BalanceQuantity5Choice avlblBal;
@XmlElement(name = "NotAvlblBal")
protected BalanceQuantity5Choice notAvlblBal;
@XmlElement(name = "PricDtls")
protected List pricDtls;
@XmlElement(name = "FXDtls")
protected List fxDtls;
@XmlElement(name = "DaysAcrd")
protected BigDecimal daysAcrd;
@XmlElement(name = "AcctBaseCcyAmts")
protected BalanceAmounts3 acctBaseCcyAmts;
@XmlElement(name = "InstrmCcyAmts")
protected BalanceAmounts3 instrmCcyAmts;
@XmlElement(name = "QtyBrkdwn")
protected List qtyBrkdwn;
@XmlElement(name = "XpsrTp")
protected ExposureType12Choice xpsrTp;
@XmlElement(name = "BalBrkdwn")
protected List balBrkdwn;
@XmlElement(name = "AddtlBalBrkdwn")
protected List addtlBalBrkdwn;
@XmlElement(name = "HldgAddtlDtls")
protected String hldgAddtlDtls;
/**
* Gets the value of the sfkpgPlc property.
*
* @return
* possible object is
* {@link SafekeepingPlaceFormat3Choice }
*
*/
public SafekeepingPlaceFormat3Choice getSfkpgPlc() {
return sfkpgPlc;
}
/**
* Sets the value of the sfkpgPlc property.
*
* @param value
* allowed object is
* {@link SafekeepingPlaceFormat3Choice }
*
*/
public AggregateBalancePerSafekeepingPlace24 setSfkpgPlc(SafekeepingPlaceFormat3Choice value) {
this.sfkpgPlc = value;
return this;
}
/**
* Gets the value of the plcOfListg property.
*
* @return
* possible object is
* {@link MarketIdentification3Choice }
*
*/
public MarketIdentification3Choice getPlcOfListg() {
return plcOfListg;
}
/**
* Sets the value of the plcOfListg property.
*
* @param value
* allowed object is
* {@link MarketIdentification3Choice }
*
*/
public AggregateBalancePerSafekeepingPlace24 setPlcOfListg(MarketIdentification3Choice value) {
this.plcOfListg = value;
return this;
}
/**
* Gets the value of the pldgee property.
*
* @return
* possible object is
* {@link PledgeeFormat1Choice }
*
*/
public PledgeeFormat1Choice getPldgee() {
return pldgee;
}
/**
* Sets the value of the pldgee property.
*
* @param value
* allowed object is
* {@link PledgeeFormat1Choice }
*
*/
public AggregateBalancePerSafekeepingPlace24 setPldgee(PledgeeFormat1Choice value) {
this.pldgee = value;
return this;
}
/**
* Gets the value of the aggtBal property.
*
* @return
* possible object is
* {@link Balance1 }
*
*/
public Balance1 getAggtBal() {
return aggtBal;
}
/**
* Sets the value of the aggtBal property.
*
* @param value
* allowed object is
* {@link Balance1 }
*
*/
public AggregateBalancePerSafekeepingPlace24 setAggtBal(Balance1 value) {
this.aggtBal = value;
return this;
}
/**
* Gets the value of the avlblBal property.
*
* @return
* possible object is
* {@link BalanceQuantity5Choice }
*
*/
public BalanceQuantity5Choice getAvlblBal() {
return avlblBal;
}
/**
* Sets the value of the avlblBal property.
*
* @param value
* allowed object is
* {@link BalanceQuantity5Choice }
*
*/
public AggregateBalancePerSafekeepingPlace24 setAvlblBal(BalanceQuantity5Choice value) {
this.avlblBal = value;
return this;
}
/**
* Gets the value of the notAvlblBal property.
*
* @return
* possible object is
* {@link BalanceQuantity5Choice }
*
*/
public BalanceQuantity5Choice getNotAvlblBal() {
return notAvlblBal;
}
/**
* Sets the value of the notAvlblBal property.
*
* @param value
* allowed object is
* {@link BalanceQuantity5Choice }
*
*/
public AggregateBalancePerSafekeepingPlace24 setNotAvlblBal(BalanceQuantity5Choice value) {
this.notAvlblBal = value;
return this;
}
/**
* Gets the value of the pricDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pricDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getPricDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PriceInformation5 }
*
*
* @return
* The value of the pricDtls property.
*/
public List getPricDtls() {
if (pricDtls == null) {
pricDtls = new ArrayList<>();
}
return this.pricDtls;
}
/**
* Gets the value of the fxDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the fxDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getFXDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ForeignExchangeTerms14 }
*
*
* @return
* The value of the fxDtls property.
*/
public List getFXDtls() {
if (fxDtls == null) {
fxDtls = new ArrayList<>();
}
return this.fxDtls;
}
/**
* Gets the value of the daysAcrd property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getDaysAcrd() {
return daysAcrd;
}
/**
* Sets the value of the daysAcrd property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public AggregateBalancePerSafekeepingPlace24 setDaysAcrd(BigDecimal value) {
this.daysAcrd = value;
return this;
}
/**
* Gets the value of the acctBaseCcyAmts property.
*
* @return
* possible object is
* {@link BalanceAmounts3 }
*
*/
public BalanceAmounts3 getAcctBaseCcyAmts() {
return acctBaseCcyAmts;
}
/**
* Sets the value of the acctBaseCcyAmts property.
*
* @param value
* allowed object is
* {@link BalanceAmounts3 }
*
*/
public AggregateBalancePerSafekeepingPlace24 setAcctBaseCcyAmts(BalanceAmounts3 value) {
this.acctBaseCcyAmts = value;
return this;
}
/**
* Gets the value of the instrmCcyAmts property.
*
* @return
* possible object is
* {@link BalanceAmounts3 }
*
*/
public BalanceAmounts3 getInstrmCcyAmts() {
return instrmCcyAmts;
}
/**
* Sets the value of the instrmCcyAmts property.
*
* @param value
* allowed object is
* {@link BalanceAmounts3 }
*
*/
public AggregateBalancePerSafekeepingPlace24 setInstrmCcyAmts(BalanceAmounts3 value) {
this.instrmCcyAmts = value;
return this;
}
/**
* Gets the value of the qtyBrkdwn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the qtyBrkdwn property.
*
*
* For example, to add a new item, do as follows:
*
* getQtyBrkdwn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link QuantityBreakdown23 }
*
*
* @return
* The value of the qtyBrkdwn property.
*/
public List getQtyBrkdwn() {
if (qtyBrkdwn == null) {
qtyBrkdwn = new ArrayList<>();
}
return this.qtyBrkdwn;
}
/**
* Gets the value of the xpsrTp property.
*
* @return
* possible object is
* {@link ExposureType12Choice }
*
*/
public ExposureType12Choice getXpsrTp() {
return xpsrTp;
}
/**
* Sets the value of the xpsrTp property.
*
* @param value
* allowed object is
* {@link ExposureType12Choice }
*
*/
public AggregateBalancePerSafekeepingPlace24 setXpsrTp(ExposureType12Choice value) {
this.xpsrTp = value;
return this;
}
/**
* Gets the value of the balBrkdwn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the balBrkdwn property.
*
*
* For example, to add a new item, do as follows:
*
* getBalBrkdwn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SubBalanceInformation11 }
*
*
* @return
* The value of the balBrkdwn property.
*/
public List getBalBrkdwn() {
if (balBrkdwn == null) {
balBrkdwn = new ArrayList<>();
}
return this.balBrkdwn;
}
/**
* Gets the value of the addtlBalBrkdwn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlBalBrkdwn property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlBalBrkdwn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalBalanceInformation11 }
*
*
* @return
* The value of the addtlBalBrkdwn property.
*/
public List getAddtlBalBrkdwn() {
if (addtlBalBrkdwn == null) {
addtlBalBrkdwn = new ArrayList<>();
}
return this.addtlBalBrkdwn;
}
/**
* Gets the value of the hldgAddtlDtls property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHldgAddtlDtls() {
return hldgAddtlDtls;
}
/**
* Sets the value of the hldgAddtlDtls property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public AggregateBalancePerSafekeepingPlace24 setHldgAddtlDtls(String value) {
this.hldgAddtlDtls = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the pricDtls list.
* @see #getPricDtls()
*
*/
public AggregateBalancePerSafekeepingPlace24 addPricDtls(PriceInformation5 pricDtls) {
getPricDtls().add(pricDtls);
return this;
}
/**
* Adds a new item to the fXDtls list.
* @see #getFXDtls()
*
*/
public AggregateBalancePerSafekeepingPlace24 addFXDtls(ForeignExchangeTerms14 fXDtls) {
getFXDtls().add(fXDtls);
return this;
}
/**
* Adds a new item to the qtyBrkdwn list.
* @see #getQtyBrkdwn()
*
*/
public AggregateBalancePerSafekeepingPlace24 addQtyBrkdwn(QuantityBreakdown23 qtyBrkdwn) {
getQtyBrkdwn().add(qtyBrkdwn);
return this;
}
/**
* Adds a new item to the balBrkdwn list.
* @see #getBalBrkdwn()
*
*/
public AggregateBalancePerSafekeepingPlace24 addBalBrkdwn(SubBalanceInformation11 balBrkdwn) {
getBalBrkdwn().add(balBrkdwn);
return this;
}
/**
* Adds a new item to the addtlBalBrkdwn list.
* @see #getAddtlBalBrkdwn()
*
*/
public AggregateBalancePerSafekeepingPlace24 addAddtlBalBrkdwn(AdditionalBalanceInformation11 addtlBalBrkdwn) {
getAddtlBalBrkdwn().add(addtlBalBrkdwn);
return this;
}
}