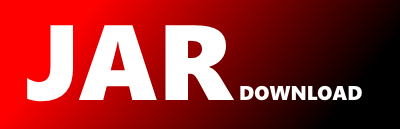
com.prowidesoftware.swift.model.mx.dic.AlternativeFundsAccountingStatementOfHoldingsV01 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* An account servicer, for example, a transfer agent or administrator, sends the AlternativeFundsAccountingStatementOfHoldings message to the account owner, for example, an investment manager, custodian, fund manager or an account owner's designated agent, to provide detailed holdings of the portfolio at a specified moment in time.
* There may be one or more parties between the account servicer and the account owner.
* The message provides, at a moment in time, valuations of the portfolio together with details of each financial instrument holding.
* Usage
* The AlternativeFundsAccountingStatementOfHoldings message is sent by the account servicer to the account owner:
* - at a frequency agreed bilaterally between the account servicer and the account owner,
* - as a response to a request for statement sent by the account owner.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AlternativeFundsAccountingStatementOfHoldingsV01", propOrder = {
"msgId",
"prvsRef",
"rltdRef",
"msgPgntn",
"invstmtAcctDtls",
"stmtGnlDtls",
"rltdPtyDtls",
"balForAcct",
"ttlVals",
"xtnsn"
})
public class AlternativeFundsAccountingStatementOfHoldingsV01 {
@XmlElement(name = "MsgId", required = true)
protected MessageIdentification1 msgId;
@XmlElement(name = "PrvsRef")
protected List prvsRef;
@XmlElement(name = "RltdRef")
protected List rltdRef;
@XmlElement(name = "MsgPgntn", required = true)
protected Pagination msgPgntn;
@XmlElement(name = "InvstmtAcctDtls", required = true)
protected InvestmentAccount30 invstmtAcctDtls;
@XmlElement(name = "StmtGnlDtls", required = true)
protected Statement10 stmtGnlDtls;
@XmlElement(name = "RltdPtyDtls")
protected List rltdPtyDtls;
@XmlElement(name = "BalForAcct")
protected List balForAcct;
@XmlElement(name = "TtlVals")
protected TotalValueInPageAndStatement ttlVals;
@XmlElement(name = "Xtnsn")
protected List xtnsn;
/**
* Gets the value of the msgId property.
*
* @return
* possible object is
* {@link MessageIdentification1 }
*
*/
public MessageIdentification1 getMsgId() {
return msgId;
}
/**
* Sets the value of the msgId property.
*
* @param value
* allowed object is
* {@link MessageIdentification1 }
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 setMsgId(MessageIdentification1 value) {
this.msgId = value;
return this;
}
/**
* Gets the value of the prvsRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the prvsRef property.
*
*
* For example, to add a new item, do as follows:
*
* getPrvsRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalReference4 }
*
*
* @return
* The value of the prvsRef property.
*/
public List getPrvsRef() {
if (prvsRef == null) {
prvsRef = new ArrayList<>();
}
return this.prvsRef;
}
/**
* Gets the value of the rltdRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rltdRef property.
*
*
* For example, to add a new item, do as follows:
*
* getRltdRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalReference4 }
*
*
* @return
* The value of the rltdRef property.
*/
public List getRltdRef() {
if (rltdRef == null) {
rltdRef = new ArrayList<>();
}
return this.rltdRef;
}
/**
* Gets the value of the msgPgntn property.
*
* @return
* possible object is
* {@link Pagination }
*
*/
public Pagination getMsgPgntn() {
return msgPgntn;
}
/**
* Sets the value of the msgPgntn property.
*
* @param value
* allowed object is
* {@link Pagination }
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 setMsgPgntn(Pagination value) {
this.msgPgntn = value;
return this;
}
/**
* Gets the value of the invstmtAcctDtls property.
*
* @return
* possible object is
* {@link InvestmentAccount30 }
*
*/
public InvestmentAccount30 getInvstmtAcctDtls() {
return invstmtAcctDtls;
}
/**
* Sets the value of the invstmtAcctDtls property.
*
* @param value
* allowed object is
* {@link InvestmentAccount30 }
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 setInvstmtAcctDtls(InvestmentAccount30 value) {
this.invstmtAcctDtls = value;
return this;
}
/**
* Gets the value of the stmtGnlDtls property.
*
* @return
* possible object is
* {@link Statement10 }
*
*/
public Statement10 getStmtGnlDtls() {
return stmtGnlDtls;
}
/**
* Sets the value of the stmtGnlDtls property.
*
* @param value
* allowed object is
* {@link Statement10 }
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 setStmtGnlDtls(Statement10 value) {
this.stmtGnlDtls = value;
return this;
}
/**
* Gets the value of the rltdPtyDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rltdPtyDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getRltdPtyDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary17 }
*
*
* @return
* The value of the rltdPtyDtls property.
*/
public List getRltdPtyDtls() {
if (rltdPtyDtls == null) {
rltdPtyDtls = new ArrayList<>();
}
return this.rltdPtyDtls;
}
/**
* Gets the value of the balForAcct property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the balForAcct property.
*
*
* For example, to add a new item, do as follows:
*
* getBalForAcct().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AggregateBalanceInformation5 }
*
*
* @return
* The value of the balForAcct property.
*/
public List getBalForAcct() {
if (balForAcct == null) {
balForAcct = new ArrayList<>();
}
return this.balForAcct;
}
/**
* Gets the value of the ttlVals property.
*
* @return
* possible object is
* {@link TotalValueInPageAndStatement }
*
*/
public TotalValueInPageAndStatement getTtlVals() {
return ttlVals;
}
/**
* Sets the value of the ttlVals property.
*
* @param value
* allowed object is
* {@link TotalValueInPageAndStatement }
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 setTtlVals(TotalValueInPageAndStatement value) {
this.ttlVals = value;
return this;
}
/**
* Gets the value of the xtnsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the xtnsn property.
*
*
* For example, to add a new item, do as follows:
*
* getXtnsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension1 }
*
*
* @return
* The value of the xtnsn property.
*/
public List getXtnsn() {
if (xtnsn == null) {
xtnsn = new ArrayList<>();
}
return this.xtnsn;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the prvsRef list.
* @see #getPrvsRef()
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 addPrvsRef(AdditionalReference4 prvsRef) {
getPrvsRef().add(prvsRef);
return this;
}
/**
* Adds a new item to the rltdRef list.
* @see #getRltdRef()
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 addRltdRef(AdditionalReference4 rltdRef) {
getRltdRef().add(rltdRef);
return this;
}
/**
* Adds a new item to the rltdPtyDtls list.
* @see #getRltdPtyDtls()
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 addRltdPtyDtls(Intermediary17 rltdPtyDtls) {
getRltdPtyDtls().add(rltdPtyDtls);
return this;
}
/**
* Adds a new item to the balForAcct list.
* @see #getBalForAcct()
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 addBalForAcct(AggregateBalanceInformation5 balForAcct) {
getBalForAcct().add(balForAcct);
return this;
}
/**
* Adds a new item to the xtnsn list.
* @see #getXtnsn()
*
*/
public AlternativeFundsAccountingStatementOfHoldingsV01 addXtnsn(Extension1 xtnsn) {
getXtnsn().add(xtnsn);
return this;
}
}