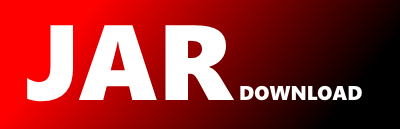
com.prowidesoftware.swift.model.mx.dic.BankToCustomerStatementV03 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The BankToCustomerStatement message is sent by the account servicer to an account owner or to a party authorised by the account owner to receive the message. It is used to inform the account owner, or authorised party, of the entries booked to the account, and to provide the owner with balance information on the account at a given point in time.
* Usage
* The BankToCustomerStatement message can contain reports for more than one account. It provides information for cash management and/or reconciliation.
* It contains information on booked entries only.
* It can include underlying details of transactions that have been included in the entry.
* The message is exchanged as defined between the account servicer and the account owner. It provides information on items that have been booked to the account and also balance information. Depending on services and schedule agreed between banks and their customers, statements may be generated and exchanged accordingly, for example for intraday or prior day periods.
* It is possible that the receiver of the message is not the account owner, but a party entitled through arrangement with the account owner to receive the account information (also known as recipient).
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "BankToCustomerStatementV03", propOrder = {
"grpHdr",
"stmt",
"splmtryData"
})
public class BankToCustomerStatementV03 {
@XmlElement(name = "GrpHdr", required = true)
protected GroupHeader58 grpHdr;
@XmlElement(name = "Stmt", required = true)
protected List stmt;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the grpHdr property.
*
* @return
* possible object is
* {@link GroupHeader58 }
*
*/
public GroupHeader58 getGrpHdr() {
return grpHdr;
}
/**
* Sets the value of the grpHdr property.
*
* @param value
* allowed object is
* {@link GroupHeader58 }
*
*/
public BankToCustomerStatementV03 setGrpHdr(GroupHeader58 value) {
this.grpHdr = value;
return this;
}
/**
* Gets the value of the stmt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the stmt property.
*
*
* For example, to add a new item, do as follows:
*
* getStmt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AccountStatement3 }
*
*
* @return
* The value of the stmt property.
*/
public List getStmt() {
if (stmt == null) {
stmt = new ArrayList<>();
}
return this.stmt;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the stmt list.
* @see #getStmt()
*
*/
public BankToCustomerStatementV03 addStmt(AccountStatement3 stmt) {
getStmt().add(stmt);
return this;
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public BankToCustomerStatementV03 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}