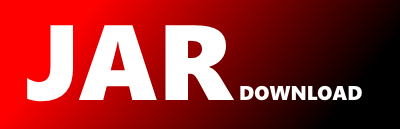
com.prowidesoftware.swift.model.mx.dic.BaselineMatchReportV03 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Scope
* The BaselineMatchReport message is sent by the matching application to the parties involved in the establishment of a transaction.
* The message is used to inform about either the successful establishment of a transaction (baseline) or the mis-matches found between two baseline initiation messages.
* Usage
* The BaselineMatchReport message can be sent by the matching application to
* - the parties involved in the establishment of a transaction in the push-through mode, that is the senders of InitialBaselineSubmission and BaselineReSubmission messages including the instruction push-through. In the outlined scenario the message is used to inform either about the successful establishment of a transaction in the matching application or about mis-matches found between two baseline initiation messages,or
* - the party establishing a transaction in the lodge mode, that is the sender of an InitialBaselineSubmission message including the instruction lodge. In the outlined scenario the message is used to inform about the successful establishment of a transaction in the matching application.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "BaselineMatchReportV03", propOrder = {
"rptId",
"txId",
"estblishdBaselnId",
"txSts",
"usrTxRef",
"buyr",
"sellr",
"buyrBk",
"sellrBk",
"baselnEstblishmtTrils",
"cmpardDocRef",
"rpt",
"reqForActn"
})
public class BaselineMatchReportV03 {
@XmlElement(name = "RptId", required = true)
protected MessageIdentification1 rptId;
@XmlElement(name = "TxId", required = true)
protected SimpleIdentificationInformation txId;
@XmlElement(name = "EstblishdBaselnId")
protected DocumentIdentification3 estblishdBaselnId;
@XmlElement(name = "TxSts", required = true)
protected TransactionStatus4 txSts;
@XmlElement(name = "UsrTxRef")
protected List usrTxRef;
@XmlElement(name = "Buyr", required = true)
protected PartyIdentification26 buyr;
@XmlElement(name = "Sellr", required = true)
protected PartyIdentification26 sellr;
@XmlElement(name = "BuyrBk", required = true)
protected BICIdentification1 buyrBk;
@XmlElement(name = "SellrBk", required = true)
protected BICIdentification1 sellrBk;
@XmlElement(name = "BaselnEstblishmtTrils", required = true)
protected Limit1 baselnEstblishmtTrils;
@XmlElement(name = "CmpardDocRef", required = true)
protected List cmpardDocRef;
@XmlElement(name = "Rpt", required = true)
protected MisMatchReport3 rpt;
@XmlElement(name = "ReqForActn")
protected PendingActivity2 reqForActn;
/**
* Gets the value of the rptId property.
*
* @return
* possible object is
* {@link MessageIdentification1 }
*
*/
public MessageIdentification1 getRptId() {
return rptId;
}
/**
* Sets the value of the rptId property.
*
* @param value
* allowed object is
* {@link MessageIdentification1 }
*
*/
public BaselineMatchReportV03 setRptId(MessageIdentification1 value) {
this.rptId = value;
return this;
}
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link SimpleIdentificationInformation }
*
*/
public SimpleIdentificationInformation getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link SimpleIdentificationInformation }
*
*/
public BaselineMatchReportV03 setTxId(SimpleIdentificationInformation value) {
this.txId = value;
return this;
}
/**
* Gets the value of the estblishdBaselnId property.
*
* @return
* possible object is
* {@link DocumentIdentification3 }
*
*/
public DocumentIdentification3 getEstblishdBaselnId() {
return estblishdBaselnId;
}
/**
* Sets the value of the estblishdBaselnId property.
*
* @param value
* allowed object is
* {@link DocumentIdentification3 }
*
*/
public BaselineMatchReportV03 setEstblishdBaselnId(DocumentIdentification3 value) {
this.estblishdBaselnId = value;
return this;
}
/**
* Gets the value of the txSts property.
*
* @return
* possible object is
* {@link TransactionStatus4 }
*
*/
public TransactionStatus4 getTxSts() {
return txSts;
}
/**
* Sets the value of the txSts property.
*
* @param value
* allowed object is
* {@link TransactionStatus4 }
*
*/
public BaselineMatchReportV03 setTxSts(TransactionStatus4 value) {
this.txSts = value;
return this;
}
/**
* Gets the value of the usrTxRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the usrTxRef property.
*
*
* For example, to add a new item, do as follows:
*
* getUsrTxRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DocumentIdentification5 }
*
*
* @return
* The value of the usrTxRef property.
*/
public List getUsrTxRef() {
if (usrTxRef == null) {
usrTxRef = new ArrayList<>();
}
return this.usrTxRef;
}
/**
* Gets the value of the buyr property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getBuyr() {
return buyr;
}
/**
* Sets the value of the buyr property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public BaselineMatchReportV03 setBuyr(PartyIdentification26 value) {
this.buyr = value;
return this;
}
/**
* Gets the value of the sellr property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getSellr() {
return sellr;
}
/**
* Sets the value of the sellr property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public BaselineMatchReportV03 setSellr(PartyIdentification26 value) {
this.sellr = value;
return this;
}
/**
* Gets the value of the buyrBk property.
*
* @return
* possible object is
* {@link BICIdentification1 }
*
*/
public BICIdentification1 getBuyrBk() {
return buyrBk;
}
/**
* Sets the value of the buyrBk property.
*
* @param value
* allowed object is
* {@link BICIdentification1 }
*
*/
public BaselineMatchReportV03 setBuyrBk(BICIdentification1 value) {
this.buyrBk = value;
return this;
}
/**
* Gets the value of the sellrBk property.
*
* @return
* possible object is
* {@link BICIdentification1 }
*
*/
public BICIdentification1 getSellrBk() {
return sellrBk;
}
/**
* Sets the value of the sellrBk property.
*
* @param value
* allowed object is
* {@link BICIdentification1 }
*
*/
public BaselineMatchReportV03 setSellrBk(BICIdentification1 value) {
this.sellrBk = value;
return this;
}
/**
* Gets the value of the baselnEstblishmtTrils property.
*
* @return
* possible object is
* {@link Limit1 }
*
*/
public Limit1 getBaselnEstblishmtTrils() {
return baselnEstblishmtTrils;
}
/**
* Sets the value of the baselnEstblishmtTrils property.
*
* @param value
* allowed object is
* {@link Limit1 }
*
*/
public BaselineMatchReportV03 setBaselnEstblishmtTrils(Limit1 value) {
this.baselnEstblishmtTrils = value;
return this;
}
/**
* Gets the value of the cmpardDocRef property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cmpardDocRef property.
*
*
* For example, to add a new item, do as follows:
*
* getCmpardDocRef().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DocumentIdentification4 }
*
*
* @return
* The value of the cmpardDocRef property.
*/
public List getCmpardDocRef() {
if (cmpardDocRef == null) {
cmpardDocRef = new ArrayList<>();
}
return this.cmpardDocRef;
}
/**
* Gets the value of the rpt property.
*
* @return
* possible object is
* {@link MisMatchReport3 }
*
*/
public MisMatchReport3 getRpt() {
return rpt;
}
/**
* Sets the value of the rpt property.
*
* @param value
* allowed object is
* {@link MisMatchReport3 }
*
*/
public BaselineMatchReportV03 setRpt(MisMatchReport3 value) {
this.rpt = value;
return this;
}
/**
* Gets the value of the reqForActn property.
*
* @return
* possible object is
* {@link PendingActivity2 }
*
*/
public PendingActivity2 getReqForActn() {
return reqForActn;
}
/**
* Sets the value of the reqForActn property.
*
* @param value
* allowed object is
* {@link PendingActivity2 }
*
*/
public BaselineMatchReportV03 setReqForActn(PendingActivity2 value) {
this.reqForActn = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the usrTxRef list.
* @see #getUsrTxRef()
*
*/
public BaselineMatchReportV03 addUsrTxRef(DocumentIdentification5 usrTxRef) {
getUsrTxRef().add(usrTxRef);
return this;
}
/**
* Adds a new item to the cmpardDocRef list.
* @see #getCmpardDocRef()
*
*/
public BaselineMatchReportV03 addCmpardDocRef(DocumentIdentification4 cmpardDocRef) {
getCmpardDocRef().add(cmpardDocRef);
return this;
}
}