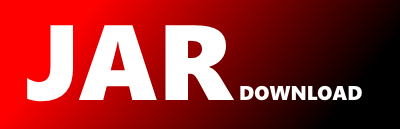
com.prowidesoftware.swift.model.mx.dic.BillingStatement1 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateTimeAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Details of the statement reporting the bank services billing.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "BillingStatement1", propOrder = {
"stmtId",
"frToDt",
"creDtTm",
"sts",
"acctChrtcs",
"rateData",
"ccyXchg",
"bal",
"compstn",
"svc",
"taxRgn",
"balAdjstmnt",
"svcAdjstmnt"
})
public class BillingStatement1 {
@XmlElement(name = "StmtId", required = true)
protected String stmtId;
@XmlElement(name = "FrToDt", required = true)
protected DatePeriod1 frToDt;
@XmlElement(name = "CreDtTm", required = true, type = String.class)
@XmlJavaTypeAdapter(IsoDateTimeAdapter.class)
@XmlSchemaType(name = "dateTime")
protected OffsetDateTime creDtTm;
@XmlElement(name = "Sts", required = true)
@XmlSchemaType(name = "string")
protected BillingStatementStatus1Code sts;
@XmlElement(name = "AcctChrtcs", required = true)
protected CashAccountCharacteristics1 acctChrtcs;
@XmlElement(name = "RateData")
protected List rateData;
@XmlElement(name = "CcyXchg")
protected List ccyXchg;
@XmlElement(name = "Bal")
protected List bal;
@XmlElement(name = "Compstn")
protected List compstn;
@XmlElement(name = "Svc")
protected List svc;
@XmlElement(name = "TaxRgn")
protected List taxRgn;
@XmlElement(name = "BalAdjstmnt")
protected List balAdjstmnt;
@XmlElement(name = "SvcAdjstmnt")
protected List svcAdjstmnt;
/**
* Gets the value of the stmtId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStmtId() {
return stmtId;
}
/**
* Sets the value of the stmtId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public BillingStatement1 setStmtId(String value) {
this.stmtId = value;
return this;
}
/**
* Gets the value of the frToDt property.
*
* @return
* possible object is
* {@link DatePeriod1 }
*
*/
public DatePeriod1 getFrToDt() {
return frToDt;
}
/**
* Sets the value of the frToDt property.
*
* @param value
* allowed object is
* {@link DatePeriod1 }
*
*/
public BillingStatement1 setFrToDt(DatePeriod1 value) {
this.frToDt = value;
return this;
}
/**
* Gets the value of the creDtTm property.
*
* @return
* possible object is
* {@link String }
*
*/
public OffsetDateTime getCreDtTm() {
return creDtTm;
}
/**
* Sets the value of the creDtTm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public BillingStatement1 setCreDtTm(OffsetDateTime value) {
this.creDtTm = value;
return this;
}
/**
* Gets the value of the sts property.
*
* @return
* possible object is
* {@link BillingStatementStatus1Code }
*
*/
public BillingStatementStatus1Code getSts() {
return sts;
}
/**
* Sets the value of the sts property.
*
* @param value
* allowed object is
* {@link BillingStatementStatus1Code }
*
*/
public BillingStatement1 setSts(BillingStatementStatus1Code value) {
this.sts = value;
return this;
}
/**
* Gets the value of the acctChrtcs property.
*
* @return
* possible object is
* {@link CashAccountCharacteristics1 }
*
*/
public CashAccountCharacteristics1 getAcctChrtcs() {
return acctChrtcs;
}
/**
* Sets the value of the acctChrtcs property.
*
* @param value
* allowed object is
* {@link CashAccountCharacteristics1 }
*
*/
public BillingStatement1 setAcctChrtcs(CashAccountCharacteristics1 value) {
this.acctChrtcs = value;
return this;
}
/**
* Gets the value of the rateData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rateData property.
*
*
* For example, to add a new item, do as follows:
*
* getRateData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BillingRate1 }
*
*
* @return
* The value of the rateData property.
*/
public List getRateData() {
if (rateData == null) {
rateData = new ArrayList<>();
}
return this.rateData;
}
/**
* Gets the value of the ccyXchg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the ccyXchg property.
*
*
* For example, to add a new item, do as follows:
*
* getCcyXchg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CurrencyExchange6 }
*
*
* @return
* The value of the ccyXchg property.
*/
public List getCcyXchg() {
if (ccyXchg == null) {
ccyXchg = new ArrayList<>();
}
return this.ccyXchg;
}
/**
* Gets the value of the bal property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the bal property.
*
*
* For example, to add a new item, do as follows:
*
* getBal().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BillingBalance1 }
*
*
* @return
* The value of the bal property.
*/
public List getBal() {
if (bal == null) {
bal = new ArrayList<>();
}
return this.bal;
}
/**
* Gets the value of the compstn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the compstn property.
*
*
* For example, to add a new item, do as follows:
*
* getCompstn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BillingCompensation1 }
*
*
* @return
* The value of the compstn property.
*/
public List getCompstn() {
if (compstn == null) {
compstn = new ArrayList<>();
}
return this.compstn;
}
/**
* Gets the value of the svc property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the svc property.
*
*
* For example, to add a new item, do as follows:
*
* getSvc().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BillingService1 }
*
*
* @return
* The value of the svc property.
*/
public List getSvc() {
if (svc == null) {
svc = new ArrayList<>();
}
return this.svc;
}
/**
* Gets the value of the taxRgn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the taxRgn property.
*
*
* For example, to add a new item, do as follows:
*
* getTaxRgn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BillingTaxRegion1 }
*
*
* @return
* The value of the taxRgn property.
*/
public List getTaxRgn() {
if (taxRgn == null) {
taxRgn = new ArrayList<>();
}
return this.taxRgn;
}
/**
* Gets the value of the balAdjstmnt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the balAdjstmnt property.
*
*
* For example, to add a new item, do as follows:
*
* getBalAdjstmnt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BalanceAdjustment1 }
*
*
* @return
* The value of the balAdjstmnt property.
*/
public List getBalAdjstmnt() {
if (balAdjstmnt == null) {
balAdjstmnt = new ArrayList<>();
}
return this.balAdjstmnt;
}
/**
* Gets the value of the svcAdjstmnt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the svcAdjstmnt property.
*
*
* For example, to add a new item, do as follows:
*
* getSvcAdjstmnt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BillingServiceAdjustment1 }
*
*
* @return
* The value of the svcAdjstmnt property.
*/
public List getSvcAdjstmnt() {
if (svcAdjstmnt == null) {
svcAdjstmnt = new ArrayList<>();
}
return this.svcAdjstmnt;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the rateData list.
* @see #getRateData()
*
*/
public BillingStatement1 addRateData(BillingRate1 rateData) {
getRateData().add(rateData);
return this;
}
/**
* Adds a new item to the ccyXchg list.
* @see #getCcyXchg()
*
*/
public BillingStatement1 addCcyXchg(CurrencyExchange6 ccyXchg) {
getCcyXchg().add(ccyXchg);
return this;
}
/**
* Adds a new item to the bal list.
* @see #getBal()
*
*/
public BillingStatement1 addBal(BillingBalance1 bal) {
getBal().add(bal);
return this;
}
/**
* Adds a new item to the compstn list.
* @see #getCompstn()
*
*/
public BillingStatement1 addCompstn(BillingCompensation1 compstn) {
getCompstn().add(compstn);
return this;
}
/**
* Adds a new item to the svc list.
* @see #getSvc()
*
*/
public BillingStatement1 addSvc(BillingService1 svc) {
getSvc().add(svc);
return this;
}
/**
* Adds a new item to the taxRgn list.
* @see #getTaxRgn()
*
*/
public BillingStatement1 addTaxRgn(BillingTaxRegion1 taxRgn) {
getTaxRgn().add(taxRgn);
return this;
}
/**
* Adds a new item to the balAdjstmnt list.
* @see #getBalAdjstmnt()
*
*/
public BillingStatement1 addBalAdjstmnt(BalanceAdjustment1 balAdjstmnt) {
getBalAdjstmnt().add(balAdjstmnt);
return this;
}
/**
* Adds a new item to the svcAdjstmnt list.
* @see #getSvcAdjstmnt()
*
*/
public BillingStatement1 addSvcAdjstmnt(BillingServiceAdjustment1 svcAdjstmnt) {
getSvcAdjstmnt().add(svcAdjstmnt);
return this;
}
}