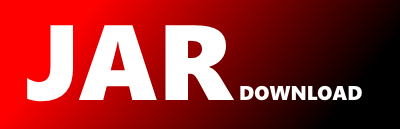
com.prowidesoftware.swift.model.mx.dic.CCPBackTestingDefinitionReportV01 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* The CCPBackTestingDefinitionReport message is sent from the central counterparty to the national competent authority. It is used to inform the national competent authority of the methodology used to carry out backtesting.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CCPBackTestingDefinitionReportV01", propOrder = {
"mthdlgy",
"splmtryData"
})
public class CCPBackTestingDefinitionReportV01 {
@XmlElement(name = "Mthdlgy", required = true)
protected List mthdlgy;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the mthdlgy property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the mthdlgy property.
*
*
* For example, to add a new item, do as follows:
*
* getMthdlgy().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BackTestingMethodology1 }
*
*
* @return
* The value of the mthdlgy property.
*/
public List getMthdlgy() {
if (mthdlgy == null) {
mthdlgy = new ArrayList<>();
}
return this.mthdlgy;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the mthdlgy list.
* @see #getMthdlgy()
*
*/
public CCPBackTestingDefinitionReportV01 addMthdlgy(BackTestingMethodology1 mthdlgy) {
getMthdlgy().add(mthdlgy);
return this;
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public CCPBackTestingDefinitionReportV01 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}