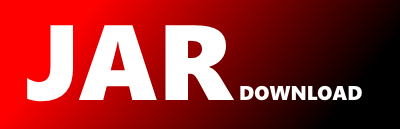
com.prowidesoftware.swift.model.mx.dic.CardPaymentEnvironment52 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Environment of the card payment transaction.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CardPaymentEnvironment52", propOrder = {
"acqrr",
"mrchnt",
"poi",
"card",
"cstmrDvc",
"wllt",
"pmtTkn",
"crdhldr",
"prtctdCrdhldrData"
})
public class CardPaymentEnvironment52 {
@XmlElement(name = "Acqrr")
protected Acquirer4 acqrr;
@XmlElement(name = "Mrchnt")
protected Organisation25 mrchnt;
@XmlElement(name = "POI")
protected PointOfInteraction5 poi;
@XmlElement(name = "Card", required = true)
protected PaymentCard20 card;
@XmlElement(name = "CstmrDvc")
protected CustomerDevice1 cstmrDvc;
@XmlElement(name = "Wllt")
protected CustomerDevice1 wllt;
@XmlElement(name = "PmtTkn")
protected CardPaymentToken3 pmtTkn;
@XmlElement(name = "Crdhldr")
protected Cardholder11 crdhldr;
@XmlElement(name = "PrtctdCrdhldrData")
protected ContentInformationType10 prtctdCrdhldrData;
/**
* Gets the value of the acqrr property.
*
* @return
* possible object is
* {@link Acquirer4 }
*
*/
public Acquirer4 getAcqrr() {
return acqrr;
}
/**
* Sets the value of the acqrr property.
*
* @param value
* allowed object is
* {@link Acquirer4 }
*
*/
public CardPaymentEnvironment52 setAcqrr(Acquirer4 value) {
this.acqrr = value;
return this;
}
/**
* Gets the value of the mrchnt property.
*
* @return
* possible object is
* {@link Organisation25 }
*
*/
public Organisation25 getMrchnt() {
return mrchnt;
}
/**
* Sets the value of the mrchnt property.
*
* @param value
* allowed object is
* {@link Organisation25 }
*
*/
public CardPaymentEnvironment52 setMrchnt(Organisation25 value) {
this.mrchnt = value;
return this;
}
/**
* Gets the value of the poi property.
*
* @return
* possible object is
* {@link PointOfInteraction5 }
*
*/
public PointOfInteraction5 getPOI() {
return poi;
}
/**
* Sets the value of the poi property.
*
* @param value
* allowed object is
* {@link PointOfInteraction5 }
*
*/
public CardPaymentEnvironment52 setPOI(PointOfInteraction5 value) {
this.poi = value;
return this;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link PaymentCard20 }
*
*/
public PaymentCard20 getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link PaymentCard20 }
*
*/
public CardPaymentEnvironment52 setCard(PaymentCard20 value) {
this.card = value;
return this;
}
/**
* Gets the value of the cstmrDvc property.
*
* @return
* possible object is
* {@link CustomerDevice1 }
*
*/
public CustomerDevice1 getCstmrDvc() {
return cstmrDvc;
}
/**
* Sets the value of the cstmrDvc property.
*
* @param value
* allowed object is
* {@link CustomerDevice1 }
*
*/
public CardPaymentEnvironment52 setCstmrDvc(CustomerDevice1 value) {
this.cstmrDvc = value;
return this;
}
/**
* Gets the value of the wllt property.
*
* @return
* possible object is
* {@link CustomerDevice1 }
*
*/
public CustomerDevice1 getWllt() {
return wllt;
}
/**
* Sets the value of the wllt property.
*
* @param value
* allowed object is
* {@link CustomerDevice1 }
*
*/
public CardPaymentEnvironment52 setWllt(CustomerDevice1 value) {
this.wllt = value;
return this;
}
/**
* Gets the value of the pmtTkn property.
*
* @return
* possible object is
* {@link CardPaymentToken3 }
*
*/
public CardPaymentToken3 getPmtTkn() {
return pmtTkn;
}
/**
* Sets the value of the pmtTkn property.
*
* @param value
* allowed object is
* {@link CardPaymentToken3 }
*
*/
public CardPaymentEnvironment52 setPmtTkn(CardPaymentToken3 value) {
this.pmtTkn = value;
return this;
}
/**
* Gets the value of the crdhldr property.
*
* @return
* possible object is
* {@link Cardholder11 }
*
*/
public Cardholder11 getCrdhldr() {
return crdhldr;
}
/**
* Sets the value of the crdhldr property.
*
* @param value
* allowed object is
* {@link Cardholder11 }
*
*/
public CardPaymentEnvironment52 setCrdhldr(Cardholder11 value) {
this.crdhldr = value;
return this;
}
/**
* Gets the value of the prtctdCrdhldrData property.
*
* @return
* possible object is
* {@link ContentInformationType10 }
*
*/
public ContentInformationType10 getPrtctdCrdhldrData() {
return prtctdCrdhldrData;
}
/**
* Sets the value of the prtctdCrdhldrData property.
*
* @param value
* allowed object is
* {@link ContentInformationType10 }
*
*/
public CardPaymentEnvironment52 setPrtctdCrdhldrData(ContentInformationType10 value) {
this.prtctdCrdhldrData = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy