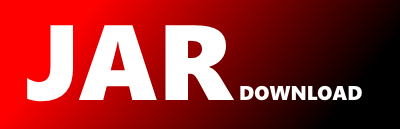
com.prowidesoftware.swift.model.mx.dic.CardPaymentTransaction40 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Transaction information in the completion advice message.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CardPaymentTransaction40", propOrder = {
"txCaptr",
"txTp",
"addtlSvc",
"svcAttr",
"mrchntCtgyCd",
"saleRefId",
"txId",
"orgnlTx",
"txSucss",
"rvsl",
"mrchntOvrrd",
"failrRsn",
"initrTxId",
"rcptTxId",
"rcncltnId",
"intrchngData",
"txDtls",
"authstnRslt",
"txVrfctnRslt",
"addtlTxData"
})
public class CardPaymentTransaction40 {
@XmlElement(name = "TxCaptr")
protected Boolean txCaptr;
@XmlElement(name = "TxTp", required = true)
@XmlSchemaType(name = "string")
protected CardPaymentServiceType5Code txTp;
@XmlElement(name = "AddtlSvc")
@XmlSchemaType(name = "string")
protected List addtlSvc;
@XmlElement(name = "SvcAttr")
@XmlSchemaType(name = "string")
protected CardPaymentServiceType3Code svcAttr;
@XmlElement(name = "MrchntCtgyCd", required = true)
protected String mrchntCtgyCd;
@XmlElement(name = "SaleRefId")
protected String saleRefId;
@XmlElement(name = "TxId", required = true)
protected TransactionIdentifier1 txId;
@XmlElement(name = "OrgnlTx")
protected CardPaymentTransaction37 orgnlTx;
@XmlElement(name = "TxSucss")
protected boolean txSucss;
@XmlElement(name = "Rvsl")
protected Boolean rvsl;
@XmlElement(name = "MrchntOvrrd")
protected Boolean mrchntOvrrd;
@XmlElement(name = "FailrRsn")
@XmlSchemaType(name = "string")
protected List failrRsn;
@XmlElement(name = "InitrTxId")
protected String initrTxId;
@XmlElement(name = "RcptTxId")
protected String rcptTxId;
@XmlElement(name = "RcncltnId")
protected String rcncltnId;
@XmlElement(name = "IntrchngData")
protected String intrchngData;
@XmlElement(name = "TxDtls", required = true)
protected CardPaymentTransactionDetails21 txDtls;
@XmlElement(name = "AuthstnRslt")
protected AuthorisationResult5 authstnRslt;
@XmlElement(name = "TxVrfctnRslt")
protected List txVrfctnRslt;
@XmlElement(name = "AddtlTxData")
protected List addtlTxData;
/**
* Gets the value of the txCaptr property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTxCaptr() {
return txCaptr;
}
/**
* Sets the value of the txCaptr property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public CardPaymentTransaction40 setTxCaptr(Boolean value) {
this.txCaptr = value;
return this;
}
/**
* Gets the value of the txTp property.
*
* @return
* possible object is
* {@link CardPaymentServiceType5Code }
*
*/
public CardPaymentServiceType5Code getTxTp() {
return txTp;
}
/**
* Sets the value of the txTp property.
*
* @param value
* allowed object is
* {@link CardPaymentServiceType5Code }
*
*/
public CardPaymentTransaction40 setTxTp(CardPaymentServiceType5Code value) {
this.txTp = value;
return this;
}
/**
* Gets the value of the addtlSvc property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlSvc property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlSvc().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CardPaymentServiceType6Code }
*
*
* @return
* The value of the addtlSvc property.
*/
public List getAddtlSvc() {
if (addtlSvc == null) {
addtlSvc = new ArrayList<>();
}
return this.addtlSvc;
}
/**
* Gets the value of the svcAttr property.
*
* @return
* possible object is
* {@link CardPaymentServiceType3Code }
*
*/
public CardPaymentServiceType3Code getSvcAttr() {
return svcAttr;
}
/**
* Sets the value of the svcAttr property.
*
* @param value
* allowed object is
* {@link CardPaymentServiceType3Code }
*
*/
public CardPaymentTransaction40 setSvcAttr(CardPaymentServiceType3Code value) {
this.svcAttr = value;
return this;
}
/**
* Gets the value of the mrchntCtgyCd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMrchntCtgyCd() {
return mrchntCtgyCd;
}
/**
* Sets the value of the mrchntCtgyCd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public CardPaymentTransaction40 setMrchntCtgyCd(String value) {
this.mrchntCtgyCd = value;
return this;
}
/**
* Gets the value of the saleRefId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSaleRefId() {
return saleRefId;
}
/**
* Sets the value of the saleRefId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public CardPaymentTransaction40 setSaleRefId(String value) {
this.saleRefId = value;
return this;
}
/**
* Gets the value of the txId property.
*
* @return
* possible object is
* {@link TransactionIdentifier1 }
*
*/
public TransactionIdentifier1 getTxId() {
return txId;
}
/**
* Sets the value of the txId property.
*
* @param value
* allowed object is
* {@link TransactionIdentifier1 }
*
*/
public CardPaymentTransaction40 setTxId(TransactionIdentifier1 value) {
this.txId = value;
return this;
}
/**
* Gets the value of the orgnlTx property.
*
* @return
* possible object is
* {@link CardPaymentTransaction37 }
*
*/
public CardPaymentTransaction37 getOrgnlTx() {
return orgnlTx;
}
/**
* Sets the value of the orgnlTx property.
*
* @param value
* allowed object is
* {@link CardPaymentTransaction37 }
*
*/
public CardPaymentTransaction40 setOrgnlTx(CardPaymentTransaction37 value) {
this.orgnlTx = value;
return this;
}
/**
* Gets the value of the txSucss property.
*
*/
public boolean isTxSucss() {
return txSucss;
}
/**
* Sets the value of the txSucss property.
*
*/
public CardPaymentTransaction40 setTxSucss(boolean value) {
this.txSucss = value;
return this;
}
/**
* Gets the value of the rvsl property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRvsl() {
return rvsl;
}
/**
* Sets the value of the rvsl property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public CardPaymentTransaction40 setRvsl(Boolean value) {
this.rvsl = value;
return this;
}
/**
* Gets the value of the mrchntOvrrd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isMrchntOvrrd() {
return mrchntOvrrd;
}
/**
* Sets the value of the mrchntOvrrd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public CardPaymentTransaction40 setMrchntOvrrd(Boolean value) {
this.mrchntOvrrd = value;
return this;
}
/**
* Gets the value of the failrRsn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the failrRsn property.
*
*
* For example, to add a new item, do as follows:
*
* getFailrRsn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FailureReason3Code }
*
*
* @return
* The value of the failrRsn property.
*/
public List getFailrRsn() {
if (failrRsn == null) {
failrRsn = new ArrayList<>();
}
return this.failrRsn;
}
/**
* Gets the value of the initrTxId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInitrTxId() {
return initrTxId;
}
/**
* Sets the value of the initrTxId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public CardPaymentTransaction40 setInitrTxId(String value) {
this.initrTxId = value;
return this;
}
/**
* Gets the value of the rcptTxId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRcptTxId() {
return rcptTxId;
}
/**
* Sets the value of the rcptTxId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public CardPaymentTransaction40 setRcptTxId(String value) {
this.rcptTxId = value;
return this;
}
/**
* Gets the value of the rcncltnId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRcncltnId() {
return rcncltnId;
}
/**
* Sets the value of the rcncltnId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public CardPaymentTransaction40 setRcncltnId(String value) {
this.rcncltnId = value;
return this;
}
/**
* Gets the value of the intrchngData property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIntrchngData() {
return intrchngData;
}
/**
* Sets the value of the intrchngData property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public CardPaymentTransaction40 setIntrchngData(String value) {
this.intrchngData = value;
return this;
}
/**
* Gets the value of the txDtls property.
*
* @return
* possible object is
* {@link CardPaymentTransactionDetails21 }
*
*/
public CardPaymentTransactionDetails21 getTxDtls() {
return txDtls;
}
/**
* Sets the value of the txDtls property.
*
* @param value
* allowed object is
* {@link CardPaymentTransactionDetails21 }
*
*/
public CardPaymentTransaction40 setTxDtls(CardPaymentTransactionDetails21 value) {
this.txDtls = value;
return this;
}
/**
* Gets the value of the authstnRslt property.
*
* @return
* possible object is
* {@link AuthorisationResult5 }
*
*/
public AuthorisationResult5 getAuthstnRslt() {
return authstnRslt;
}
/**
* Sets the value of the authstnRslt property.
*
* @param value
* allowed object is
* {@link AuthorisationResult5 }
*
*/
public CardPaymentTransaction40 setAuthstnRslt(AuthorisationResult5 value) {
this.authstnRslt = value;
return this;
}
/**
* Gets the value of the txVrfctnRslt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the txVrfctnRslt property.
*
*
* For example, to add a new item, do as follows:
*
* getTxVrfctnRslt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TransactionVerificationResult3 }
*
*
* @return
* The value of the txVrfctnRslt property.
*/
public List getTxVrfctnRslt() {
if (txVrfctnRslt == null) {
txVrfctnRslt = new ArrayList<>();
}
return this.txVrfctnRslt;
}
/**
* Gets the value of the addtlTxData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlTxData property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlTxData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the addtlTxData property.
*/
public List getAddtlTxData() {
if (addtlTxData == null) {
addtlTxData = new ArrayList<>();
}
return this.addtlTxData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the addtlSvc list.
* @see #getAddtlSvc()
*
*/
public CardPaymentTransaction40 addAddtlSvc(CardPaymentServiceType6Code addtlSvc) {
getAddtlSvc().add(addtlSvc);
return this;
}
/**
* Adds a new item to the failrRsn list.
* @see #getFailrRsn()
*
*/
public CardPaymentTransaction40 addFailrRsn(FailureReason3Code failrRsn) {
getFailrRsn().add(failrRsn);
return this;
}
/**
* Adds a new item to the txVrfctnRslt list.
* @see #getTxVrfctnRslt()
*
*/
public CardPaymentTransaction40 addTxVrfctnRslt(TransactionVerificationResult3 txVrfctnRslt) {
getTxVrfctnRslt().add(txVrfctnRslt);
return this;
}
/**
* Adds a new item to the addtlTxData list.
* @see #getAddtlTxData()
*
*/
public CardPaymentTransaction40 addAddtlTxData(String addtlTxData) {
getAddtlTxData().add(addtlTxData);
return this;
}
}