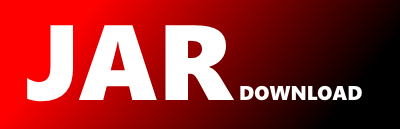
com.prowidesoftware.swift.model.mx.dic.CollateralSubstitutionConfirmationV01 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* This message is sent by:
*
* - the collateral taker or its collateral manager to the collateral giver or its collateral manager,
*
* - the collateral giver or its collateral manager to the collateral taker or its collateral manager.
*
* This message confirms the collateral delivery.
*
* Usage: The collateral taker will only release the return of collateral when the new piece of collateral is received. The collateral giver sends the collateral taker the notification that the collateral substitution (that is new piece(s) of collateral) have been released. In the event that multiple pieces of collateral are being delivered in place of the collateral due to be returned by the giver, this message should only be generated once all collateral pieces have been agreed between both parties. Then the taker confirms that the collateral substitution (that is all pieces have been received) and acknowledges return of collateral.
*
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CollateralSubstitutionConfirmationV01", propOrder = {
"id",
"ptyA",
"svcgPtyA",
"ptyB",
"svcgPtyB",
"agrmt",
"confDtls",
"splmtryData"
})
public class CollateralSubstitutionConfirmationV01 {
@XmlElement(name = "Id", required = true)
protected TransactionAndDocumentIdentification3 id;
@XmlElement(name = "PtyA", required = true)
protected PartyIdentification33Choice ptyA;
@XmlElement(name = "SvcgPtyA")
protected PartyIdentification33Choice svcgPtyA;
@XmlElement(name = "PtyB", required = true)
protected PartyIdentification33Choice ptyB;
@XmlElement(name = "SvcgPtyB")
protected PartyIdentification33Choice svcgPtyB;
@XmlElement(name = "Agrmt")
protected Agreement2 agrmt;
@XmlElement(name = "ConfDtls", required = true)
protected CollateralConfirmation1 confDtls;
@XmlElement(name = "SplmtryData")
protected List splmtryData;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link TransactionAndDocumentIdentification3 }
*
*/
public TransactionAndDocumentIdentification3 getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link TransactionAndDocumentIdentification3 }
*
*/
public CollateralSubstitutionConfirmationV01 setId(TransactionAndDocumentIdentification3 value) {
this.id = value;
return this;
}
/**
* Gets the value of the ptyA property.
*
* @return
* possible object is
* {@link PartyIdentification33Choice }
*
*/
public PartyIdentification33Choice getPtyA() {
return ptyA;
}
/**
* Sets the value of the ptyA property.
*
* @param value
* allowed object is
* {@link PartyIdentification33Choice }
*
*/
public CollateralSubstitutionConfirmationV01 setPtyA(PartyIdentification33Choice value) {
this.ptyA = value;
return this;
}
/**
* Gets the value of the svcgPtyA property.
*
* @return
* possible object is
* {@link PartyIdentification33Choice }
*
*/
public PartyIdentification33Choice getSvcgPtyA() {
return svcgPtyA;
}
/**
* Sets the value of the svcgPtyA property.
*
* @param value
* allowed object is
* {@link PartyIdentification33Choice }
*
*/
public CollateralSubstitutionConfirmationV01 setSvcgPtyA(PartyIdentification33Choice value) {
this.svcgPtyA = value;
return this;
}
/**
* Gets the value of the ptyB property.
*
* @return
* possible object is
* {@link PartyIdentification33Choice }
*
*/
public PartyIdentification33Choice getPtyB() {
return ptyB;
}
/**
* Sets the value of the ptyB property.
*
* @param value
* allowed object is
* {@link PartyIdentification33Choice }
*
*/
public CollateralSubstitutionConfirmationV01 setPtyB(PartyIdentification33Choice value) {
this.ptyB = value;
return this;
}
/**
* Gets the value of the svcgPtyB property.
*
* @return
* possible object is
* {@link PartyIdentification33Choice }
*
*/
public PartyIdentification33Choice getSvcgPtyB() {
return svcgPtyB;
}
/**
* Sets the value of the svcgPtyB property.
*
* @param value
* allowed object is
* {@link PartyIdentification33Choice }
*
*/
public CollateralSubstitutionConfirmationV01 setSvcgPtyB(PartyIdentification33Choice value) {
this.svcgPtyB = value;
return this;
}
/**
* Gets the value of the agrmt property.
*
* @return
* possible object is
* {@link Agreement2 }
*
*/
public Agreement2 getAgrmt() {
return agrmt;
}
/**
* Sets the value of the agrmt property.
*
* @param value
* allowed object is
* {@link Agreement2 }
*
*/
public CollateralSubstitutionConfirmationV01 setAgrmt(Agreement2 value) {
this.agrmt = value;
return this;
}
/**
* Gets the value of the confDtls property.
*
* @return
* possible object is
* {@link CollateralConfirmation1 }
*
*/
public CollateralConfirmation1 getConfDtls() {
return confDtls;
}
/**
* Sets the value of the confDtls property.
*
* @param value
* allowed object is
* {@link CollateralConfirmation1 }
*
*/
public CollateralSubstitutionConfirmationV01 setConfDtls(CollateralConfirmation1 value) {
this.confDtls = value;
return this;
}
/**
* Gets the value of the splmtryData property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the splmtryData property.
*
*
* For example, to add a new item, do as follows:
*
* getSplmtryData().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SupplementaryData1 }
*
*
* @return
* The value of the splmtryData property.
*/
public List getSplmtryData() {
if (splmtryData == null) {
splmtryData = new ArrayList<>();
}
return this.splmtryData;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the splmtryData list.
* @see #getSplmtryData()
*
*/
public CollateralSubstitutionConfirmationV01 addSplmtryData(SupplementaryData1 splmtryData) {
getSplmtryData().add(splmtryData);
return this;
}
}