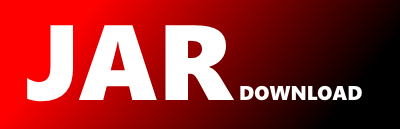
com.prowidesoftware.swift.model.mx.dic.CommercialDataSet5 Maven / Gradle / Ivy
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Goods or services that are part of a commercial trade agreement.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CommercialDataSet5", propOrder = {
"dataSetId",
"comrclDocRef",
"buyr",
"sellr",
"bllTo",
"goods",
"pmtTerms",
"sttlmTerms"
})
public class CommercialDataSet5 {
@XmlElement(name = "DataSetId", required = true)
protected DocumentIdentification1 dataSetId;
@XmlElement(name = "ComrclDocRef", required = true)
protected InvoiceIdentification1 comrclDocRef;
@XmlElement(name = "Buyr", required = true)
protected PartyIdentification26 buyr;
@XmlElement(name = "Sellr", required = true)
protected PartyIdentification26 sellr;
@XmlElement(name = "BllTo")
protected PartyIdentification26 bllTo;
@XmlElement(name = "Goods", required = true)
protected List goods;
@XmlElement(name = "PmtTerms", required = true)
protected List pmtTerms;
@XmlElement(name = "SttlmTerms", required = true)
protected SettlementTerms3 sttlmTerms;
/**
* Gets the value of the dataSetId property.
*
* @return
* possible object is
* {@link DocumentIdentification1 }
*
*/
public DocumentIdentification1 getDataSetId() {
return dataSetId;
}
/**
* Sets the value of the dataSetId property.
*
* @param value
* allowed object is
* {@link DocumentIdentification1 }
*
*/
public CommercialDataSet5 setDataSetId(DocumentIdentification1 value) {
this.dataSetId = value;
return this;
}
/**
* Gets the value of the comrclDocRef property.
*
* @return
* possible object is
* {@link InvoiceIdentification1 }
*
*/
public InvoiceIdentification1 getComrclDocRef() {
return comrclDocRef;
}
/**
* Sets the value of the comrclDocRef property.
*
* @param value
* allowed object is
* {@link InvoiceIdentification1 }
*
*/
public CommercialDataSet5 setComrclDocRef(InvoiceIdentification1 value) {
this.comrclDocRef = value;
return this;
}
/**
* Gets the value of the buyr property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getBuyr() {
return buyr;
}
/**
* Sets the value of the buyr property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public CommercialDataSet5 setBuyr(PartyIdentification26 value) {
this.buyr = value;
return this;
}
/**
* Gets the value of the sellr property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getSellr() {
return sellr;
}
/**
* Sets the value of the sellr property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public CommercialDataSet5 setSellr(PartyIdentification26 value) {
this.sellr = value;
return this;
}
/**
* Gets the value of the bllTo property.
*
* @return
* possible object is
* {@link PartyIdentification26 }
*
*/
public PartyIdentification26 getBllTo() {
return bllTo;
}
/**
* Sets the value of the bllTo property.
*
* @param value
* allowed object is
* {@link PartyIdentification26 }
*
*/
public CommercialDataSet5 setBllTo(PartyIdentification26 value) {
this.bllTo = value;
return this;
}
/**
* Gets the value of the goods property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the goods property.
*
*
* For example, to add a new item, do as follows:
*
* getGoods().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LineItem15 }
*
*
* @return
* The value of the goods property.
*/
public List getGoods() {
if (goods == null) {
goods = new ArrayList<>();
}
return this.goods;
}
/**
* Gets the value of the pmtTerms property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmtTerms property.
*
*
* For example, to add a new item, do as follows:
*
* getPmtTerms().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PaymentTerms4 }
*
*
* @return
* The value of the pmtTerms property.
*/
public List getPmtTerms() {
if (pmtTerms == null) {
pmtTerms = new ArrayList<>();
}
return this.pmtTerms;
}
/**
* Gets the value of the sttlmTerms property.
*
* @return
* possible object is
* {@link SettlementTerms3 }
*
*/
public SettlementTerms3 getSttlmTerms() {
return sttlmTerms;
}
/**
* Sets the value of the sttlmTerms property.
*
* @param value
* allowed object is
* {@link SettlementTerms3 }
*
*/
public CommercialDataSet5 setSttlmTerms(SettlementTerms3 value) {
this.sttlmTerms = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the goods list.
* @see #getGoods()
*
*/
public CommercialDataSet5 addGoods(LineItem15 goods) {
getGoods().add(goods);
return this;
}
/**
* Adds a new item to the pmtTerms list.
* @see #getPmtTerms()
*
*/
public CommercialDataSet5 addPmtTerms(PaymentTerms4 pmtTerms) {
getPmtTerms().add(pmtTerms);
return this;
}
}