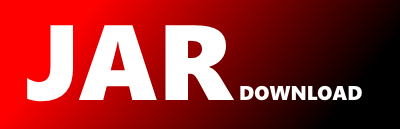
com.prowidesoftware.swift.model.mx.dic.EligiblePosition15 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Specifies the voting entitlement for rights holders.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "EligiblePosition15", propOrder = {
"acctId",
"blckChainAdrOrWllt",
"acctOwnr",
"hldgBal",
"rghtsHldr"
})
public class EligiblePosition15 {
@XmlElement(name = "AcctId")
protected String acctId;
@XmlElement(name = "BlckChainAdrOrWllt")
protected String blckChainAdrOrWllt;
@XmlElement(name = "AcctOwnr")
protected PartyIdentification241 acctOwnr;
@XmlElement(name = "HldgBal")
protected List hldgBal;
@XmlElement(name = "RghtsHldr")
protected List rghtsHldr;
/**
* Gets the value of the acctId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAcctId() {
return acctId;
}
/**
* Sets the value of the acctId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public EligiblePosition15 setAcctId(String value) {
this.acctId = value;
return this;
}
/**
* Gets the value of the blckChainAdrOrWllt property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBlckChainAdrOrWllt() {
return blckChainAdrOrWllt;
}
/**
* Sets the value of the blckChainAdrOrWllt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public EligiblePosition15 setBlckChainAdrOrWllt(String value) {
this.blckChainAdrOrWllt = value;
return this;
}
/**
* Gets the value of the acctOwnr property.
*
* @return
* possible object is
* {@link PartyIdentification241 }
*
*/
public PartyIdentification241 getAcctOwnr() {
return acctOwnr;
}
/**
* Sets the value of the acctOwnr property.
*
* @param value
* allowed object is
* {@link PartyIdentification241 }
*
*/
public EligiblePosition15 setAcctOwnr(PartyIdentification241 value) {
this.acctOwnr = value;
return this;
}
/**
* Gets the value of the hldgBal property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the hldgBal property.
*
*
* For example, to add a new item, do as follows:
*
* getHldgBal().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HoldingBalance11 }
*
*
* @return
* The value of the hldgBal property.
*/
public List getHldgBal() {
if (hldgBal == null) {
hldgBal = new ArrayList<>();
}
return this.hldgBal;
}
/**
* Gets the value of the rghtsHldr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rghtsHldr property.
*
*
* For example, to add a new item, do as follows:
*
* getRghtsHldr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyIdentification270 }
*
*
* @return
* The value of the rghtsHldr property.
*/
public List getRghtsHldr() {
if (rghtsHldr == null) {
rghtsHldr = new ArrayList<>();
}
return this.rghtsHldr;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the hldgBal list.
* @see #getHldgBal()
*
*/
public EligiblePosition15 addHldgBal(HoldingBalance11 hldgBal) {
getHldgBal().add(hldgBal);
return this;
}
/**
* Adds a new item to the rghtsHldr list.
* @see #getRghtsHldr()
*
*/
public EligiblePosition15 addRghtsHldr(PartyIdentification270 rghtsHldr) {
getRghtsHldr().add(rghtsHldr);
return this;
}
}