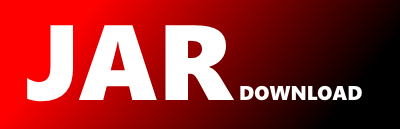
com.prowidesoftware.swift.model.mx.dic.Environment22 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Environment related to the reconciliation of the transaction.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Environment22", propOrder = {
"acqrr",
"orgtr",
"sndr",
"rcvr",
"accptr",
"pyer",
"pyee",
"termnl",
"issr",
"card",
"dstn",
"cstmrDvc",
"wllt",
"tkn",
"crdhldr"
})
public class Environment22 {
@XmlElement(name = "Acqrr")
protected PartyIdentification263 acqrr;
@XmlElement(name = "Orgtr")
protected PartyIdentification263 orgtr;
@XmlElement(name = "Sndr")
protected PartyIdentification263 sndr;
@XmlElement(name = "Rcvr")
protected PartyIdentification263 rcvr;
@XmlElement(name = "Accptr")
protected PartyIdentification254 accptr;
@XmlElement(name = "Pyer")
protected PartyIdentification257 pyer;
@XmlElement(name = "Pyee")
protected PartyIdentification257 pyee;
@XmlElement(name = "Termnl")
protected Terminal4 termnl;
@XmlElement(name = "Issr")
protected PartyIdentification263 issr;
@XmlElement(name = "Card")
protected CardData8 card;
@XmlElement(name = "Dstn")
protected PartyIdentification263 dstn;
@XmlElement(name = "CstmrDvc")
protected CustomerDevice4 cstmrDvc;
@XmlElement(name = "Wllt")
protected Wallet2 wllt;
@XmlElement(name = "Tkn")
protected Token3 tkn;
@XmlElement(name = "Crdhldr")
protected Cardholder19 crdhldr;
/**
* Gets the value of the acqrr property.
*
* @return
* possible object is
* {@link PartyIdentification263 }
*
*/
public PartyIdentification263 getAcqrr() {
return acqrr;
}
/**
* Sets the value of the acqrr property.
*
* @param value
* allowed object is
* {@link PartyIdentification263 }
*
*/
public Environment22 setAcqrr(PartyIdentification263 value) {
this.acqrr = value;
return this;
}
/**
* Gets the value of the orgtr property.
*
* @return
* possible object is
* {@link PartyIdentification263 }
*
*/
public PartyIdentification263 getOrgtr() {
return orgtr;
}
/**
* Sets the value of the orgtr property.
*
* @param value
* allowed object is
* {@link PartyIdentification263 }
*
*/
public Environment22 setOrgtr(PartyIdentification263 value) {
this.orgtr = value;
return this;
}
/**
* Gets the value of the sndr property.
*
* @return
* possible object is
* {@link PartyIdentification263 }
*
*/
public PartyIdentification263 getSndr() {
return sndr;
}
/**
* Sets the value of the sndr property.
*
* @param value
* allowed object is
* {@link PartyIdentification263 }
*
*/
public Environment22 setSndr(PartyIdentification263 value) {
this.sndr = value;
return this;
}
/**
* Gets the value of the rcvr property.
*
* @return
* possible object is
* {@link PartyIdentification263 }
*
*/
public PartyIdentification263 getRcvr() {
return rcvr;
}
/**
* Sets the value of the rcvr property.
*
* @param value
* allowed object is
* {@link PartyIdentification263 }
*
*/
public Environment22 setRcvr(PartyIdentification263 value) {
this.rcvr = value;
return this;
}
/**
* Gets the value of the accptr property.
*
* @return
* possible object is
* {@link PartyIdentification254 }
*
*/
public PartyIdentification254 getAccptr() {
return accptr;
}
/**
* Sets the value of the accptr property.
*
* @param value
* allowed object is
* {@link PartyIdentification254 }
*
*/
public Environment22 setAccptr(PartyIdentification254 value) {
this.accptr = value;
return this;
}
/**
* Gets the value of the pyer property.
*
* @return
* possible object is
* {@link PartyIdentification257 }
*
*/
public PartyIdentification257 getPyer() {
return pyer;
}
/**
* Sets the value of the pyer property.
*
* @param value
* allowed object is
* {@link PartyIdentification257 }
*
*/
public Environment22 setPyer(PartyIdentification257 value) {
this.pyer = value;
return this;
}
/**
* Gets the value of the pyee property.
*
* @return
* possible object is
* {@link PartyIdentification257 }
*
*/
public PartyIdentification257 getPyee() {
return pyee;
}
/**
* Sets the value of the pyee property.
*
* @param value
* allowed object is
* {@link PartyIdentification257 }
*
*/
public Environment22 setPyee(PartyIdentification257 value) {
this.pyee = value;
return this;
}
/**
* Gets the value of the termnl property.
*
* @return
* possible object is
* {@link Terminal4 }
*
*/
public Terminal4 getTermnl() {
return termnl;
}
/**
* Sets the value of the termnl property.
*
* @param value
* allowed object is
* {@link Terminal4 }
*
*/
public Environment22 setTermnl(Terminal4 value) {
this.termnl = value;
return this;
}
/**
* Gets the value of the issr property.
*
* @return
* possible object is
* {@link PartyIdentification263 }
*
*/
public PartyIdentification263 getIssr() {
return issr;
}
/**
* Sets the value of the issr property.
*
* @param value
* allowed object is
* {@link PartyIdentification263 }
*
*/
public Environment22 setIssr(PartyIdentification263 value) {
this.issr = value;
return this;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link CardData8 }
*
*/
public CardData8 getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link CardData8 }
*
*/
public Environment22 setCard(CardData8 value) {
this.card = value;
return this;
}
/**
* Gets the value of the dstn property.
*
* @return
* possible object is
* {@link PartyIdentification263 }
*
*/
public PartyIdentification263 getDstn() {
return dstn;
}
/**
* Sets the value of the dstn property.
*
* @param value
* allowed object is
* {@link PartyIdentification263 }
*
*/
public Environment22 setDstn(PartyIdentification263 value) {
this.dstn = value;
return this;
}
/**
* Gets the value of the cstmrDvc property.
*
* @return
* possible object is
* {@link CustomerDevice4 }
*
*/
public CustomerDevice4 getCstmrDvc() {
return cstmrDvc;
}
/**
* Sets the value of the cstmrDvc property.
*
* @param value
* allowed object is
* {@link CustomerDevice4 }
*
*/
public Environment22 setCstmrDvc(CustomerDevice4 value) {
this.cstmrDvc = value;
return this;
}
/**
* Gets the value of the wllt property.
*
* @return
* possible object is
* {@link Wallet2 }
*
*/
public Wallet2 getWllt() {
return wllt;
}
/**
* Sets the value of the wllt property.
*
* @param value
* allowed object is
* {@link Wallet2 }
*
*/
public Environment22 setWllt(Wallet2 value) {
this.wllt = value;
return this;
}
/**
* Gets the value of the tkn property.
*
* @return
* possible object is
* {@link Token3 }
*
*/
public Token3 getTkn() {
return tkn;
}
/**
* Sets the value of the tkn property.
*
* @param value
* allowed object is
* {@link Token3 }
*
*/
public Environment22 setTkn(Token3 value) {
this.tkn = value;
return this;
}
/**
* Gets the value of the crdhldr property.
*
* @return
* possible object is
* {@link Cardholder19 }
*
*/
public Cardholder19 getCrdhldr() {
return crdhldr;
}
/**
* Sets the value of the crdhldr property.
*
* @param value
* allowed object is
* {@link Cardholder19 }
*
*/
public Environment22 setCrdhldr(Cardholder19 value) {
this.crdhldr = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy