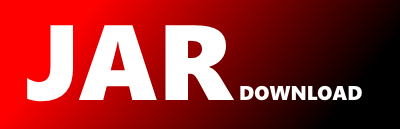
com.prowidesoftware.swift.model.mx.dic.FinancialInstrument83 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Identification of a security or other asset.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FinancialInstrument83", propOrder = {
"lineId",
"instrm",
"qty",
"prtlInstdQty",
"orgnlPctgInstd",
"trfTp",
"addtlAsst",
"notAvlbl",
"convs",
"unitsDtls",
"clntRef",
"ctrPtyRef",
"bizFlowTp",
"avrgAcqstnPric",
"trfCcy",
"ttlBookVal",
"orgnlCost",
"latstValtn",
"trfeeAcct",
"trfr",
"intrmyInf",
"reqdTradDt",
"reqdSttlmDt",
"fctvTrfDt",
"fctvSttlmDt",
"pmtDtls",
"crstllstnDtls",
"taxValtnPt",
"sttlmPtiesDtls",
"addtlInf"
})
public class FinancialInstrument83 {
@XmlElement(name = "LineId")
protected String lineId;
@XmlElement(name = "Instrm", required = true)
protected FinancialInstrument63Choice instrm;
@XmlElement(name = "Qty")
protected Quantity47 qty;
@XmlElement(name = "PrtlInstdQty")
protected Boolean prtlInstdQty;
@XmlElement(name = "OrgnlPctgInstd")
protected BigDecimal orgnlPctgInstd;
@XmlElement(name = "TrfTp", required = true)
protected TransferType2Choice trfTp;
@XmlElement(name = "AddtlAsst")
protected Boolean addtlAsst;
@XmlElement(name = "NotAvlbl")
protected Boolean notAvlbl;
@XmlElement(name = "Convs")
protected Conversion1 convs;
@XmlElement(name = "UnitsDtls")
protected List unitsDtls;
@XmlElement(name = "ClntRef")
protected AdditionalReference10 clntRef;
@XmlElement(name = "CtrPtyRef")
protected AdditionalReference10 ctrPtyRef;
@XmlElement(name = "BizFlowTp")
@XmlSchemaType(name = "string")
protected BusinessFlowType1Code bizFlowTp;
@XmlElement(name = "AvrgAcqstnPric")
protected ActiveCurrencyAndAmount avrgAcqstnPric;
@XmlElement(name = "TrfCcy")
protected String trfCcy;
@XmlElement(name = "TtlBookVal")
protected DateAndAmount2 ttlBookVal;
@XmlElement(name = "OrgnlCost")
protected ActiveCurrencyAnd13DecimalAmount orgnlCost;
@XmlElement(name = "LatstValtn")
protected DateAndAmount2 latstValtn;
@XmlElement(name = "TrfeeAcct")
protected Account28 trfeeAcct;
@XmlElement(name = "Trfr")
protected Account28 trfr;
@XmlElement(name = "IntrmyInf")
protected List intrmyInf;
@XmlElement(name = "ReqdTradDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate reqdTradDt;
@XmlElement(name = "ReqdSttlmDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate reqdSttlmDt;
@XmlElement(name = "FctvTrfDt")
protected DateAndDateTime2Choice fctvTrfDt;
@XmlElement(name = "FctvSttlmDt")
protected DateAndDateTime2Choice fctvSttlmDt;
@XmlElement(name = "PmtDtls")
protected PaymentInstrument14 pmtDtls;
@XmlElement(name = "CrstllstnDtls")
protected List crstllstnDtls;
@XmlElement(name = "TaxValtnPt")
protected Tax36 taxValtnPt;
@XmlElement(name = "SttlmPtiesDtls")
protected FundSettlementParameters17 sttlmPtiesDtls;
@XmlElement(name = "AddtlInf")
protected List addtlInf;
/**
* Gets the value of the lineId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLineId() {
return lineId;
}
/**
* Sets the value of the lineId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrument83 setLineId(String value) {
this.lineId = value;
return this;
}
/**
* Gets the value of the instrm property.
*
* @return
* possible object is
* {@link FinancialInstrument63Choice }
*
*/
public FinancialInstrument63Choice getInstrm() {
return instrm;
}
/**
* Sets the value of the instrm property.
*
* @param value
* allowed object is
* {@link FinancialInstrument63Choice }
*
*/
public FinancialInstrument83 setInstrm(FinancialInstrument63Choice value) {
this.instrm = value;
return this;
}
/**
* Gets the value of the qty property.
*
* @return
* possible object is
* {@link Quantity47 }
*
*/
public Quantity47 getQty() {
return qty;
}
/**
* Sets the value of the qty property.
*
* @param value
* allowed object is
* {@link Quantity47 }
*
*/
public FinancialInstrument83 setQty(Quantity47 value) {
this.qty = value;
return this;
}
/**
* Gets the value of the prtlInstdQty property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isPrtlInstdQty() {
return prtlInstdQty;
}
/**
* Sets the value of the prtlInstdQty property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public FinancialInstrument83 setPrtlInstdQty(Boolean value) {
this.prtlInstdQty = value;
return this;
}
/**
* Gets the value of the orgnlPctgInstd property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getOrgnlPctgInstd() {
return orgnlPctgInstd;
}
/**
* Sets the value of the orgnlPctgInstd property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrument83 setOrgnlPctgInstd(BigDecimal value) {
this.orgnlPctgInstd = value;
return this;
}
/**
* Gets the value of the trfTp property.
*
* @return
* possible object is
* {@link TransferType2Choice }
*
*/
public TransferType2Choice getTrfTp() {
return trfTp;
}
/**
* Sets the value of the trfTp property.
*
* @param value
* allowed object is
* {@link TransferType2Choice }
*
*/
public FinancialInstrument83 setTrfTp(TransferType2Choice value) {
this.trfTp = value;
return this;
}
/**
* Gets the value of the addtlAsst property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAddtlAsst() {
return addtlAsst;
}
/**
* Sets the value of the addtlAsst property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public FinancialInstrument83 setAddtlAsst(Boolean value) {
this.addtlAsst = value;
return this;
}
/**
* Gets the value of the notAvlbl property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isNotAvlbl() {
return notAvlbl;
}
/**
* Sets the value of the notAvlbl property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public FinancialInstrument83 setNotAvlbl(Boolean value) {
this.notAvlbl = value;
return this;
}
/**
* Gets the value of the convs property.
*
* @return
* possible object is
* {@link Conversion1 }
*
*/
public Conversion1 getConvs() {
return convs;
}
/**
* Sets the value of the convs property.
*
* @param value
* allowed object is
* {@link Conversion1 }
*
*/
public FinancialInstrument83 setConvs(Conversion1 value) {
this.convs = value;
return this;
}
/**
* Gets the value of the unitsDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the unitsDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getUnitsDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Unit11 }
*
*
* @return
* The value of the unitsDtls property.
*/
public List getUnitsDtls() {
if (unitsDtls == null) {
unitsDtls = new ArrayList<>();
}
return this.unitsDtls;
}
/**
* Gets the value of the clntRef property.
*
* @return
* possible object is
* {@link AdditionalReference10 }
*
*/
public AdditionalReference10 getClntRef() {
return clntRef;
}
/**
* Sets the value of the clntRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference10 }
*
*/
public FinancialInstrument83 setClntRef(AdditionalReference10 value) {
this.clntRef = value;
return this;
}
/**
* Gets the value of the ctrPtyRef property.
*
* @return
* possible object is
* {@link AdditionalReference10 }
*
*/
public AdditionalReference10 getCtrPtyRef() {
return ctrPtyRef;
}
/**
* Sets the value of the ctrPtyRef property.
*
* @param value
* allowed object is
* {@link AdditionalReference10 }
*
*/
public FinancialInstrument83 setCtrPtyRef(AdditionalReference10 value) {
this.ctrPtyRef = value;
return this;
}
/**
* Gets the value of the bizFlowTp property.
*
* @return
* possible object is
* {@link BusinessFlowType1Code }
*
*/
public BusinessFlowType1Code getBizFlowTp() {
return bizFlowTp;
}
/**
* Sets the value of the bizFlowTp property.
*
* @param value
* allowed object is
* {@link BusinessFlowType1Code }
*
*/
public FinancialInstrument83 setBizFlowTp(BusinessFlowType1Code value) {
this.bizFlowTp = value;
return this;
}
/**
* Gets the value of the avrgAcqstnPric property.
*
* @return
* possible object is
* {@link ActiveCurrencyAndAmount }
*
*/
public ActiveCurrencyAndAmount getAvrgAcqstnPric() {
return avrgAcqstnPric;
}
/**
* Sets the value of the avrgAcqstnPric property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAndAmount }
*
*/
public FinancialInstrument83 setAvrgAcqstnPric(ActiveCurrencyAndAmount value) {
this.avrgAcqstnPric = value;
return this;
}
/**
* Gets the value of the trfCcy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTrfCcy() {
return trfCcy;
}
/**
* Sets the value of the trfCcy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrument83 setTrfCcy(String value) {
this.trfCcy = value;
return this;
}
/**
* Gets the value of the ttlBookVal property.
*
* @return
* possible object is
* {@link DateAndAmount2 }
*
*/
public DateAndAmount2 getTtlBookVal() {
return ttlBookVal;
}
/**
* Sets the value of the ttlBookVal property.
*
* @param value
* allowed object is
* {@link DateAndAmount2 }
*
*/
public FinancialInstrument83 setTtlBookVal(DateAndAmount2 value) {
this.ttlBookVal = value;
return this;
}
/**
* Gets the value of the orgnlCost property.
*
* @return
* possible object is
* {@link ActiveCurrencyAnd13DecimalAmount }
*
*/
public ActiveCurrencyAnd13DecimalAmount getOrgnlCost() {
return orgnlCost;
}
/**
* Sets the value of the orgnlCost property.
*
* @param value
* allowed object is
* {@link ActiveCurrencyAnd13DecimalAmount }
*
*/
public FinancialInstrument83 setOrgnlCost(ActiveCurrencyAnd13DecimalAmount value) {
this.orgnlCost = value;
return this;
}
/**
* Gets the value of the latstValtn property.
*
* @return
* possible object is
* {@link DateAndAmount2 }
*
*/
public DateAndAmount2 getLatstValtn() {
return latstValtn;
}
/**
* Sets the value of the latstValtn property.
*
* @param value
* allowed object is
* {@link DateAndAmount2 }
*
*/
public FinancialInstrument83 setLatstValtn(DateAndAmount2 value) {
this.latstValtn = value;
return this;
}
/**
* Gets the value of the trfeeAcct property.
*
* @return
* possible object is
* {@link Account28 }
*
*/
public Account28 getTrfeeAcct() {
return trfeeAcct;
}
/**
* Sets the value of the trfeeAcct property.
*
* @param value
* allowed object is
* {@link Account28 }
*
*/
public FinancialInstrument83 setTrfeeAcct(Account28 value) {
this.trfeeAcct = value;
return this;
}
/**
* Gets the value of the trfr property.
*
* @return
* possible object is
* {@link Account28 }
*
*/
public Account28 getTrfr() {
return trfr;
}
/**
* Sets the value of the trfr property.
*
* @param value
* allowed object is
* {@link Account28 }
*
*/
public FinancialInstrument83 setTrfr(Account28 value) {
this.trfr = value;
return this;
}
/**
* Gets the value of the intrmyInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the intrmyInf property.
*
*
* For example, to add a new item, do as follows:
*
* getIntrmyInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Intermediary43 }
*
*
* @return
* The value of the intrmyInf property.
*/
public List getIntrmyInf() {
if (intrmyInf == null) {
intrmyInf = new ArrayList<>();
}
return this.intrmyInf;
}
/**
* Gets the value of the reqdTradDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getReqdTradDt() {
return reqdTradDt;
}
/**
* Sets the value of the reqdTradDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrument83 setReqdTradDt(LocalDate value) {
this.reqdTradDt = value;
return this;
}
/**
* Gets the value of the reqdSttlmDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getReqdSttlmDt() {
return reqdSttlmDt;
}
/**
* Sets the value of the reqdSttlmDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrument83 setReqdSttlmDt(LocalDate value) {
this.reqdSttlmDt = value;
return this;
}
/**
* Gets the value of the fctvTrfDt property.
*
* @return
* possible object is
* {@link DateAndDateTime2Choice }
*
*/
public DateAndDateTime2Choice getFctvTrfDt() {
return fctvTrfDt;
}
/**
* Sets the value of the fctvTrfDt property.
*
* @param value
* allowed object is
* {@link DateAndDateTime2Choice }
*
*/
public FinancialInstrument83 setFctvTrfDt(DateAndDateTime2Choice value) {
this.fctvTrfDt = value;
return this;
}
/**
* Gets the value of the fctvSttlmDt property.
*
* @return
* possible object is
* {@link DateAndDateTime2Choice }
*
*/
public DateAndDateTime2Choice getFctvSttlmDt() {
return fctvSttlmDt;
}
/**
* Sets the value of the fctvSttlmDt property.
*
* @param value
* allowed object is
* {@link DateAndDateTime2Choice }
*
*/
public FinancialInstrument83 setFctvSttlmDt(DateAndDateTime2Choice value) {
this.fctvSttlmDt = value;
return this;
}
/**
* Gets the value of the pmtDtls property.
*
* @return
* possible object is
* {@link PaymentInstrument14 }
*
*/
public PaymentInstrument14 getPmtDtls() {
return pmtDtls;
}
/**
* Sets the value of the pmtDtls property.
*
* @param value
* allowed object is
* {@link PaymentInstrument14 }
*
*/
public FinancialInstrument83 setPmtDtls(PaymentInstrument14 value) {
this.pmtDtls = value;
return this;
}
/**
* Gets the value of the crstllstnDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the crstllstnDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getCrstllstnDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Crystallisation2 }
*
*
* @return
* The value of the crstllstnDtls property.
*/
public List getCrstllstnDtls() {
if (crstllstnDtls == null) {
crstllstnDtls = new ArrayList<>();
}
return this.crstllstnDtls;
}
/**
* Gets the value of the taxValtnPt property.
*
* @return
* possible object is
* {@link Tax36 }
*
*/
public Tax36 getTaxValtnPt() {
return taxValtnPt;
}
/**
* Sets the value of the taxValtnPt property.
*
* @param value
* allowed object is
* {@link Tax36 }
*
*/
public FinancialInstrument83 setTaxValtnPt(Tax36 value) {
this.taxValtnPt = value;
return this;
}
/**
* Gets the value of the sttlmPtiesDtls property.
*
* @return
* possible object is
* {@link FundSettlementParameters17 }
*
*/
public FundSettlementParameters17 getSttlmPtiesDtls() {
return sttlmPtiesDtls;
}
/**
* Sets the value of the sttlmPtiesDtls property.
*
* @param value
* allowed object is
* {@link FundSettlementParameters17 }
*
*/
public FinancialInstrument83 setSttlmPtiesDtls(FundSettlementParameters17 value) {
this.sttlmPtiesDtls = value;
return this;
}
/**
* Gets the value of the addtlInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlInf property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditionalInformation15 }
*
*
* @return
* The value of the addtlInf property.
*/
public List getAddtlInf() {
if (addtlInf == null) {
addtlInf = new ArrayList<>();
}
return this.addtlInf;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the unitsDtls list.
* @see #getUnitsDtls()
*
*/
public FinancialInstrument83 addUnitsDtls(Unit11 unitsDtls) {
getUnitsDtls().add(unitsDtls);
return this;
}
/**
* Adds a new item to the intrmyInf list.
* @see #getIntrmyInf()
*
*/
public FinancialInstrument83 addIntrmyInf(Intermediary43 intrmyInf) {
getIntrmyInf().add(intrmyInf);
return this;
}
/**
* Adds a new item to the crstllstnDtls list.
* @see #getCrstllstnDtls()
*
*/
public FinancialInstrument83 addCrstllstnDtls(Crystallisation2 crstllstnDtls) {
getCrstllstnDtls().add(crstllstnDtls);
return this;
}
/**
* Adds a new item to the addtlInf list.
* @see #getAddtlInf()
*
*/
public FinancialInstrument83 addAddtlInf(AdditionalInformation15 addtlInf) {
getAddtlInf().add(addtlInf);
return this;
}
}