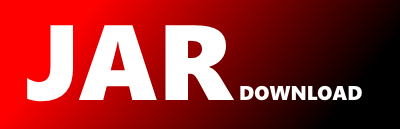
com.prowidesoftware.swift.model.mx.dic.FinancialInstrumentAttributes16 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pw-iso20022 Show documentation
Show all versions of pw-iso20022 Show documentation
Prowide Library for ISO 20022 messages
The newest version!
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Description of the financial instrument.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FinancialInstrumentAttributes16", propOrder = {
"sctyId",
"plcOfListg",
"dayCntBsis",
"clssfctnTp",
"optnStyle",
"dnmtnCcy",
"nxtCpnDt",
"fltgRateFxgDt",
"mtrtyDt",
"isseDt",
"nxtCllblDt",
"putblDt",
"dtdDt",
"convsDt",
"prvsFctr",
"nxtFctr",
"intrstRate",
"nxtIntrstRate",
"minNmnlQty",
"minExrcblQty",
"minExrcblMltplQty",
"ctrctSz",
"issePric"
})
public class FinancialInstrumentAttributes16 {
@XmlElement(name = "SctyId", required = true)
protected SecurityIdentification14 sctyId;
@XmlElement(name = "PlcOfListg")
protected MarketIdentification2 plcOfListg;
@XmlElement(name = "DayCntBsis")
protected InterestComputationMethodFormat1Choice dayCntBsis;
@XmlElement(name = "ClssfctnTp")
protected ClassificationType2Choice clssfctnTp;
@XmlElement(name = "OptnStyle")
protected OptionStyle4Choice optnStyle;
@XmlElement(name = "DnmtnCcy")
protected String dnmtnCcy;
@XmlElement(name = "NxtCpnDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate nxtCpnDt;
@XmlElement(name = "FltgRateFxgDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate fltgRateFxgDt;
@XmlElement(name = "MtrtyDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate mtrtyDt;
@XmlElement(name = "IsseDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate isseDt;
@XmlElement(name = "NxtCllblDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate nxtCllblDt;
@XmlElement(name = "PutblDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate putblDt;
@XmlElement(name = "DtdDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate dtdDt;
@XmlElement(name = "ConvsDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate convsDt;
@XmlElement(name = "PrvsFctr")
protected RateFormat3Choice prvsFctr;
@XmlElement(name = "NxtFctr")
protected RateFormat3Choice nxtFctr;
@XmlElement(name = "IntrstRate")
protected RateFormat3Choice intrstRate;
@XmlElement(name = "NxtIntrstRate")
protected RateFormat3Choice nxtIntrstRate;
@XmlElement(name = "MinNmnlQty")
protected FinancialInstrumentQuantity1Choice minNmnlQty;
@XmlElement(name = "MinExrcblQty")
protected FinancialInstrumentQuantity1Choice minExrcblQty;
@XmlElement(name = "MinExrcblMltplQty")
protected FinancialInstrumentQuantity1Choice minExrcblMltplQty;
@XmlElement(name = "CtrctSz")
protected FinancialInstrumentQuantity1Choice ctrctSz;
@XmlElement(name = "IssePric")
protected PriceFormat19Choice issePric;
/**
* Gets the value of the sctyId property.
*
* @return
* possible object is
* {@link SecurityIdentification14 }
*
*/
public SecurityIdentification14 getSctyId() {
return sctyId;
}
/**
* Sets the value of the sctyId property.
*
* @param value
* allowed object is
* {@link SecurityIdentification14 }
*
*/
public FinancialInstrumentAttributes16 setSctyId(SecurityIdentification14 value) {
this.sctyId = value;
return this;
}
/**
* Gets the value of the plcOfListg property.
*
* @return
* possible object is
* {@link MarketIdentification2 }
*
*/
public MarketIdentification2 getPlcOfListg() {
return plcOfListg;
}
/**
* Sets the value of the plcOfListg property.
*
* @param value
* allowed object is
* {@link MarketIdentification2 }
*
*/
public FinancialInstrumentAttributes16 setPlcOfListg(MarketIdentification2 value) {
this.plcOfListg = value;
return this;
}
/**
* Gets the value of the dayCntBsis property.
*
* @return
* possible object is
* {@link InterestComputationMethodFormat1Choice }
*
*/
public InterestComputationMethodFormat1Choice getDayCntBsis() {
return dayCntBsis;
}
/**
* Sets the value of the dayCntBsis property.
*
* @param value
* allowed object is
* {@link InterestComputationMethodFormat1Choice }
*
*/
public FinancialInstrumentAttributes16 setDayCntBsis(InterestComputationMethodFormat1Choice value) {
this.dayCntBsis = value;
return this;
}
/**
* Gets the value of the clssfctnTp property.
*
* @return
* possible object is
* {@link ClassificationType2Choice }
*
*/
public ClassificationType2Choice getClssfctnTp() {
return clssfctnTp;
}
/**
* Sets the value of the clssfctnTp property.
*
* @param value
* allowed object is
* {@link ClassificationType2Choice }
*
*/
public FinancialInstrumentAttributes16 setClssfctnTp(ClassificationType2Choice value) {
this.clssfctnTp = value;
return this;
}
/**
* Gets the value of the optnStyle property.
*
* @return
* possible object is
* {@link OptionStyle4Choice }
*
*/
public OptionStyle4Choice getOptnStyle() {
return optnStyle;
}
/**
* Sets the value of the optnStyle property.
*
* @param value
* allowed object is
* {@link OptionStyle4Choice }
*
*/
public FinancialInstrumentAttributes16 setOptnStyle(OptionStyle4Choice value) {
this.optnStyle = value;
return this;
}
/**
* Gets the value of the dnmtnCcy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDnmtnCcy() {
return dnmtnCcy;
}
/**
* Sets the value of the dnmtnCcy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setDnmtnCcy(String value) {
this.dnmtnCcy = value;
return this;
}
/**
* Gets the value of the nxtCpnDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getNxtCpnDt() {
return nxtCpnDt;
}
/**
* Sets the value of the nxtCpnDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setNxtCpnDt(LocalDate value) {
this.nxtCpnDt = value;
return this;
}
/**
* Gets the value of the fltgRateFxgDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getFltgRateFxgDt() {
return fltgRateFxgDt;
}
/**
* Sets the value of the fltgRateFxgDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setFltgRateFxgDt(LocalDate value) {
this.fltgRateFxgDt = value;
return this;
}
/**
* Gets the value of the mtrtyDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getMtrtyDt() {
return mtrtyDt;
}
/**
* Sets the value of the mtrtyDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setMtrtyDt(LocalDate value) {
this.mtrtyDt = value;
return this;
}
/**
* Gets the value of the isseDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getIsseDt() {
return isseDt;
}
/**
* Sets the value of the isseDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setIsseDt(LocalDate value) {
this.isseDt = value;
return this;
}
/**
* Gets the value of the nxtCllblDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getNxtCllblDt() {
return nxtCllblDt;
}
/**
* Sets the value of the nxtCllblDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setNxtCllblDt(LocalDate value) {
this.nxtCllblDt = value;
return this;
}
/**
* Gets the value of the putblDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getPutblDt() {
return putblDt;
}
/**
* Sets the value of the putblDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setPutblDt(LocalDate value) {
this.putblDt = value;
return this;
}
/**
* Gets the value of the dtdDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getDtdDt() {
return dtdDt;
}
/**
* Sets the value of the dtdDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setDtdDt(LocalDate value) {
this.dtdDt = value;
return this;
}
/**
* Gets the value of the convsDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getConvsDt() {
return convsDt;
}
/**
* Sets the value of the convsDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes16 setConvsDt(LocalDate value) {
this.convsDt = value;
return this;
}
/**
* Gets the value of the prvsFctr property.
*
* @return
* possible object is
* {@link RateFormat3Choice }
*
*/
public RateFormat3Choice getPrvsFctr() {
return prvsFctr;
}
/**
* Sets the value of the prvsFctr property.
*
* @param value
* allowed object is
* {@link RateFormat3Choice }
*
*/
public FinancialInstrumentAttributes16 setPrvsFctr(RateFormat3Choice value) {
this.prvsFctr = value;
return this;
}
/**
* Gets the value of the nxtFctr property.
*
* @return
* possible object is
* {@link RateFormat3Choice }
*
*/
public RateFormat3Choice getNxtFctr() {
return nxtFctr;
}
/**
* Sets the value of the nxtFctr property.
*
* @param value
* allowed object is
* {@link RateFormat3Choice }
*
*/
public FinancialInstrumentAttributes16 setNxtFctr(RateFormat3Choice value) {
this.nxtFctr = value;
return this;
}
/**
* Gets the value of the intrstRate property.
*
* @return
* possible object is
* {@link RateFormat3Choice }
*
*/
public RateFormat3Choice getIntrstRate() {
return intrstRate;
}
/**
* Sets the value of the intrstRate property.
*
* @param value
* allowed object is
* {@link RateFormat3Choice }
*
*/
public FinancialInstrumentAttributes16 setIntrstRate(RateFormat3Choice value) {
this.intrstRate = value;
return this;
}
/**
* Gets the value of the nxtIntrstRate property.
*
* @return
* possible object is
* {@link RateFormat3Choice }
*
*/
public RateFormat3Choice getNxtIntrstRate() {
return nxtIntrstRate;
}
/**
* Sets the value of the nxtIntrstRate property.
*
* @param value
* allowed object is
* {@link RateFormat3Choice }
*
*/
public FinancialInstrumentAttributes16 setNxtIntrstRate(RateFormat3Choice value) {
this.nxtIntrstRate = value;
return this;
}
/**
* Gets the value of the minNmnlQty property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentQuantity1Choice getMinNmnlQty() {
return minNmnlQty;
}
/**
* Sets the value of the minNmnlQty property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentAttributes16 setMinNmnlQty(FinancialInstrumentQuantity1Choice value) {
this.minNmnlQty = value;
return this;
}
/**
* Gets the value of the minExrcblQty property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentQuantity1Choice getMinExrcblQty() {
return minExrcblQty;
}
/**
* Sets the value of the minExrcblQty property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentAttributes16 setMinExrcblQty(FinancialInstrumentQuantity1Choice value) {
this.minExrcblQty = value;
return this;
}
/**
* Gets the value of the minExrcblMltplQty property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentQuantity1Choice getMinExrcblMltplQty() {
return minExrcblMltplQty;
}
/**
* Sets the value of the minExrcblMltplQty property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentAttributes16 setMinExrcblMltplQty(FinancialInstrumentQuantity1Choice value) {
this.minExrcblMltplQty = value;
return this;
}
/**
* Gets the value of the ctrctSz property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentQuantity1Choice getCtrctSz() {
return ctrctSz;
}
/**
* Sets the value of the ctrctSz property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1Choice }
*
*/
public FinancialInstrumentAttributes16 setCtrctSz(FinancialInstrumentQuantity1Choice value) {
this.ctrctSz = value;
return this;
}
/**
* Gets the value of the issePric property.
*
* @return
* possible object is
* {@link PriceFormat19Choice }
*
*/
public PriceFormat19Choice getIssePric() {
return issePric;
}
/**
* Sets the value of the issePric property.
*
* @param value
* allowed object is
* {@link PriceFormat19Choice }
*
*/
public FinancialInstrumentAttributes16 setIssePric(PriceFormat19Choice value) {
this.issePric = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy