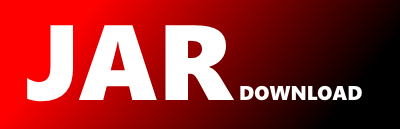
com.prowidesoftware.swift.model.mx.dic.FinancialInstrumentAttributes78 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Elements characterising a financial instrument.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FinancialInstrumentAttributes78", propOrder = {
"plcOfListg",
"dayCntBsis",
"regnForm",
"pmtFrqcy",
"pmtSts",
"varblRateChngFrqcy",
"clssfctnTp",
"optnStyle",
"optnTp",
"dnmtnCcy",
"cpnDt",
"xpryDt",
"fltgRateFxgDt",
"mtrtyDt",
"isseDt",
"nxtCllblDt",
"putblDt",
"dtdDt",
"frstPmtDt",
"prvsFctr",
"curFctr",
"nxtFctr",
"intrstRate",
"yldToMtrtyRate",
"nxtIntrstRate",
"indxRateBsis",
"cpnAttchdNb",
"poolNb",
"varblRateInd",
"cllblInd",
"putblInd",
"mktOrIndctvPric",
"exrcPric",
"sbcptPric",
"convsPric",
"strkPric",
"minNmnlQty",
"ctrctSz",
"undrlygFinInstrmId",
"finInstrmAttrAddtlDtls"
})
public class FinancialInstrumentAttributes78 {
@XmlElement(name = "PlcOfListg")
protected MarketIdentification4Choice plcOfListg;
@XmlElement(name = "DayCntBsis")
protected InterestComputationMethodFormat5Choice dayCntBsis;
@XmlElement(name = "RegnForm")
protected FormOfSecurity7Choice regnForm;
@XmlElement(name = "PmtFrqcy")
protected Frequency27Choice pmtFrqcy;
@XmlElement(name = "PmtSts")
protected SecuritiesPaymentStatus6Choice pmtSts;
@XmlElement(name = "VarblRateChngFrqcy")
protected Frequency27Choice varblRateChngFrqcy;
@XmlElement(name = "ClssfctnTp")
protected ClassificationType33Choice clssfctnTp;
@XmlElement(name = "OptnStyle")
protected OptionStyle9Choice optnStyle;
@XmlElement(name = "OptnTp")
protected OptionType7Choice optnTp;
@XmlElement(name = "DnmtnCcy")
protected String dnmtnCcy;
@XmlElement(name = "CpnDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate cpnDt;
@XmlElement(name = "XpryDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate xpryDt;
@XmlElement(name = "FltgRateFxgDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate fltgRateFxgDt;
@XmlElement(name = "MtrtyDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate mtrtyDt;
@XmlElement(name = "IsseDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate isseDt;
@XmlElement(name = "NxtCllblDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate nxtCllblDt;
@XmlElement(name = "PutblDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate putblDt;
@XmlElement(name = "DtdDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate dtdDt;
@XmlElement(name = "FrstPmtDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate frstPmtDt;
@XmlElement(name = "PrvsFctr")
protected BigDecimal prvsFctr;
@XmlElement(name = "CurFctr")
protected BigDecimal curFctr;
@XmlElement(name = "NxtFctr")
protected BigDecimal nxtFctr;
@XmlElement(name = "IntrstRate")
protected BigDecimal intrstRate;
@XmlElement(name = "YldToMtrtyRate")
protected BigDecimal yldToMtrtyRate;
@XmlElement(name = "NxtIntrstRate")
protected BigDecimal nxtIntrstRate;
@XmlElement(name = "IndxRateBsis")
protected BigDecimal indxRateBsis;
@XmlElement(name = "CpnAttchdNb")
protected Number23Choice cpnAttchdNb;
@XmlElement(name = "PoolNb")
protected GenericIdentification39 poolNb;
@XmlElement(name = "VarblRateInd")
protected Boolean varblRateInd;
@XmlElement(name = "CllblInd")
protected Boolean cllblInd;
@XmlElement(name = "PutblInd")
protected Boolean putblInd;
@XmlElement(name = "MktOrIndctvPric")
protected PriceType2Choice mktOrIndctvPric;
@XmlElement(name = "ExrcPric")
protected Price3 exrcPric;
@XmlElement(name = "SbcptPric")
protected Price3 sbcptPric;
@XmlElement(name = "ConvsPric")
protected Price3 convsPric;
@XmlElement(name = "StrkPric")
protected Price3 strkPric;
@XmlElement(name = "MinNmnlQty")
protected FinancialInstrumentQuantity15Choice minNmnlQty;
@XmlElement(name = "CtrctSz")
protected FinancialInstrumentQuantity15Choice ctrctSz;
@XmlElement(name = "UndrlygFinInstrmId")
protected List undrlygFinInstrmId;
@XmlElement(name = "FinInstrmAttrAddtlDtls")
protected String finInstrmAttrAddtlDtls;
/**
* Gets the value of the plcOfListg property.
*
* @return
* possible object is
* {@link MarketIdentification4Choice }
*
*/
public MarketIdentification4Choice getPlcOfListg() {
return plcOfListg;
}
/**
* Sets the value of the plcOfListg property.
*
* @param value
* allowed object is
* {@link MarketIdentification4Choice }
*
*/
public FinancialInstrumentAttributes78 setPlcOfListg(MarketIdentification4Choice value) {
this.plcOfListg = value;
return this;
}
/**
* Gets the value of the dayCntBsis property.
*
* @return
* possible object is
* {@link InterestComputationMethodFormat5Choice }
*
*/
public InterestComputationMethodFormat5Choice getDayCntBsis() {
return dayCntBsis;
}
/**
* Sets the value of the dayCntBsis property.
*
* @param value
* allowed object is
* {@link InterestComputationMethodFormat5Choice }
*
*/
public FinancialInstrumentAttributes78 setDayCntBsis(InterestComputationMethodFormat5Choice value) {
this.dayCntBsis = value;
return this;
}
/**
* Gets the value of the regnForm property.
*
* @return
* possible object is
* {@link FormOfSecurity7Choice }
*
*/
public FormOfSecurity7Choice getRegnForm() {
return regnForm;
}
/**
* Sets the value of the regnForm property.
*
* @param value
* allowed object is
* {@link FormOfSecurity7Choice }
*
*/
public FinancialInstrumentAttributes78 setRegnForm(FormOfSecurity7Choice value) {
this.regnForm = value;
return this;
}
/**
* Gets the value of the pmtFrqcy property.
*
* @return
* possible object is
* {@link Frequency27Choice }
*
*/
public Frequency27Choice getPmtFrqcy() {
return pmtFrqcy;
}
/**
* Sets the value of the pmtFrqcy property.
*
* @param value
* allowed object is
* {@link Frequency27Choice }
*
*/
public FinancialInstrumentAttributes78 setPmtFrqcy(Frequency27Choice value) {
this.pmtFrqcy = value;
return this;
}
/**
* Gets the value of the pmtSts property.
*
* @return
* possible object is
* {@link SecuritiesPaymentStatus6Choice }
*
*/
public SecuritiesPaymentStatus6Choice getPmtSts() {
return pmtSts;
}
/**
* Sets the value of the pmtSts property.
*
* @param value
* allowed object is
* {@link SecuritiesPaymentStatus6Choice }
*
*/
public FinancialInstrumentAttributes78 setPmtSts(SecuritiesPaymentStatus6Choice value) {
this.pmtSts = value;
return this;
}
/**
* Gets the value of the varblRateChngFrqcy property.
*
* @return
* possible object is
* {@link Frequency27Choice }
*
*/
public Frequency27Choice getVarblRateChngFrqcy() {
return varblRateChngFrqcy;
}
/**
* Sets the value of the varblRateChngFrqcy property.
*
* @param value
* allowed object is
* {@link Frequency27Choice }
*
*/
public FinancialInstrumentAttributes78 setVarblRateChngFrqcy(Frequency27Choice value) {
this.varblRateChngFrqcy = value;
return this;
}
/**
* Gets the value of the clssfctnTp property.
*
* @return
* possible object is
* {@link ClassificationType33Choice }
*
*/
public ClassificationType33Choice getClssfctnTp() {
return clssfctnTp;
}
/**
* Sets the value of the clssfctnTp property.
*
* @param value
* allowed object is
* {@link ClassificationType33Choice }
*
*/
public FinancialInstrumentAttributes78 setClssfctnTp(ClassificationType33Choice value) {
this.clssfctnTp = value;
return this;
}
/**
* Gets the value of the optnStyle property.
*
* @return
* possible object is
* {@link OptionStyle9Choice }
*
*/
public OptionStyle9Choice getOptnStyle() {
return optnStyle;
}
/**
* Sets the value of the optnStyle property.
*
* @param value
* allowed object is
* {@link OptionStyle9Choice }
*
*/
public FinancialInstrumentAttributes78 setOptnStyle(OptionStyle9Choice value) {
this.optnStyle = value;
return this;
}
/**
* Gets the value of the optnTp property.
*
* @return
* possible object is
* {@link OptionType7Choice }
*
*/
public OptionType7Choice getOptnTp() {
return optnTp;
}
/**
* Sets the value of the optnTp property.
*
* @param value
* allowed object is
* {@link OptionType7Choice }
*
*/
public FinancialInstrumentAttributes78 setOptnTp(OptionType7Choice value) {
this.optnTp = value;
return this;
}
/**
* Gets the value of the dnmtnCcy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDnmtnCcy() {
return dnmtnCcy;
}
/**
* Sets the value of the dnmtnCcy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setDnmtnCcy(String value) {
this.dnmtnCcy = value;
return this;
}
/**
* Gets the value of the cpnDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getCpnDt() {
return cpnDt;
}
/**
* Sets the value of the cpnDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setCpnDt(LocalDate value) {
this.cpnDt = value;
return this;
}
/**
* Gets the value of the xpryDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getXpryDt() {
return xpryDt;
}
/**
* Sets the value of the xpryDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setXpryDt(LocalDate value) {
this.xpryDt = value;
return this;
}
/**
* Gets the value of the fltgRateFxgDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getFltgRateFxgDt() {
return fltgRateFxgDt;
}
/**
* Sets the value of the fltgRateFxgDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setFltgRateFxgDt(LocalDate value) {
this.fltgRateFxgDt = value;
return this;
}
/**
* Gets the value of the mtrtyDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getMtrtyDt() {
return mtrtyDt;
}
/**
* Sets the value of the mtrtyDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setMtrtyDt(LocalDate value) {
this.mtrtyDt = value;
return this;
}
/**
* Gets the value of the isseDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getIsseDt() {
return isseDt;
}
/**
* Sets the value of the isseDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setIsseDt(LocalDate value) {
this.isseDt = value;
return this;
}
/**
* Gets the value of the nxtCllblDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getNxtCllblDt() {
return nxtCllblDt;
}
/**
* Sets the value of the nxtCllblDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setNxtCllblDt(LocalDate value) {
this.nxtCllblDt = value;
return this;
}
/**
* Gets the value of the putblDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getPutblDt() {
return putblDt;
}
/**
* Sets the value of the putblDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setPutblDt(LocalDate value) {
this.putblDt = value;
return this;
}
/**
* Gets the value of the dtdDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getDtdDt() {
return dtdDt;
}
/**
* Sets the value of the dtdDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setDtdDt(LocalDate value) {
this.dtdDt = value;
return this;
}
/**
* Gets the value of the frstPmtDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getFrstPmtDt() {
return frstPmtDt;
}
/**
* Sets the value of the frstPmtDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setFrstPmtDt(LocalDate value) {
this.frstPmtDt = value;
return this;
}
/**
* Gets the value of the prvsFctr property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getPrvsFctr() {
return prvsFctr;
}
/**
* Sets the value of the prvsFctr property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrumentAttributes78 setPrvsFctr(BigDecimal value) {
this.prvsFctr = value;
return this;
}
/**
* Gets the value of the curFctr property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getCurFctr() {
return curFctr;
}
/**
* Sets the value of the curFctr property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrumentAttributes78 setCurFctr(BigDecimal value) {
this.curFctr = value;
return this;
}
/**
* Gets the value of the nxtFctr property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getNxtFctr() {
return nxtFctr;
}
/**
* Sets the value of the nxtFctr property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrumentAttributes78 setNxtFctr(BigDecimal value) {
this.nxtFctr = value;
return this;
}
/**
* Gets the value of the intrstRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getIntrstRate() {
return intrstRate;
}
/**
* Sets the value of the intrstRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrumentAttributes78 setIntrstRate(BigDecimal value) {
this.intrstRate = value;
return this;
}
/**
* Gets the value of the yldToMtrtyRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getYldToMtrtyRate() {
return yldToMtrtyRate;
}
/**
* Sets the value of the yldToMtrtyRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrumentAttributes78 setYldToMtrtyRate(BigDecimal value) {
this.yldToMtrtyRate = value;
return this;
}
/**
* Gets the value of the nxtIntrstRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getNxtIntrstRate() {
return nxtIntrstRate;
}
/**
* Sets the value of the nxtIntrstRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrumentAttributes78 setNxtIntrstRate(BigDecimal value) {
this.nxtIntrstRate = value;
return this;
}
/**
* Gets the value of the indxRateBsis property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getIndxRateBsis() {
return indxRateBsis;
}
/**
* Sets the value of the indxRateBsis property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FinancialInstrumentAttributes78 setIndxRateBsis(BigDecimal value) {
this.indxRateBsis = value;
return this;
}
/**
* Gets the value of the cpnAttchdNb property.
*
* @return
* possible object is
* {@link Number23Choice }
*
*/
public Number23Choice getCpnAttchdNb() {
return cpnAttchdNb;
}
/**
* Sets the value of the cpnAttchdNb property.
*
* @param value
* allowed object is
* {@link Number23Choice }
*
*/
public FinancialInstrumentAttributes78 setCpnAttchdNb(Number23Choice value) {
this.cpnAttchdNb = value;
return this;
}
/**
* Gets the value of the poolNb property.
*
* @return
* possible object is
* {@link GenericIdentification39 }
*
*/
public GenericIdentification39 getPoolNb() {
return poolNb;
}
/**
* Sets the value of the poolNb property.
*
* @param value
* allowed object is
* {@link GenericIdentification39 }
*
*/
public FinancialInstrumentAttributes78 setPoolNb(GenericIdentification39 value) {
this.poolNb = value;
return this;
}
/**
* Gets the value of the varblRateInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isVarblRateInd() {
return varblRateInd;
}
/**
* Sets the value of the varblRateInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public FinancialInstrumentAttributes78 setVarblRateInd(Boolean value) {
this.varblRateInd = value;
return this;
}
/**
* Gets the value of the cllblInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCllblInd() {
return cllblInd;
}
/**
* Sets the value of the cllblInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public FinancialInstrumentAttributes78 setCllblInd(Boolean value) {
this.cllblInd = value;
return this;
}
/**
* Gets the value of the putblInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isPutblInd() {
return putblInd;
}
/**
* Sets the value of the putblInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public FinancialInstrumentAttributes78 setPutblInd(Boolean value) {
this.putblInd = value;
return this;
}
/**
* Gets the value of the mktOrIndctvPric property.
*
* @return
* possible object is
* {@link PriceType2Choice }
*
*/
public PriceType2Choice getMktOrIndctvPric() {
return mktOrIndctvPric;
}
/**
* Sets the value of the mktOrIndctvPric property.
*
* @param value
* allowed object is
* {@link PriceType2Choice }
*
*/
public FinancialInstrumentAttributes78 setMktOrIndctvPric(PriceType2Choice value) {
this.mktOrIndctvPric = value;
return this;
}
/**
* Gets the value of the exrcPric property.
*
* @return
* possible object is
* {@link Price3 }
*
*/
public Price3 getExrcPric() {
return exrcPric;
}
/**
* Sets the value of the exrcPric property.
*
* @param value
* allowed object is
* {@link Price3 }
*
*/
public FinancialInstrumentAttributes78 setExrcPric(Price3 value) {
this.exrcPric = value;
return this;
}
/**
* Gets the value of the sbcptPric property.
*
* @return
* possible object is
* {@link Price3 }
*
*/
public Price3 getSbcptPric() {
return sbcptPric;
}
/**
* Sets the value of the sbcptPric property.
*
* @param value
* allowed object is
* {@link Price3 }
*
*/
public FinancialInstrumentAttributes78 setSbcptPric(Price3 value) {
this.sbcptPric = value;
return this;
}
/**
* Gets the value of the convsPric property.
*
* @return
* possible object is
* {@link Price3 }
*
*/
public Price3 getConvsPric() {
return convsPric;
}
/**
* Sets the value of the convsPric property.
*
* @param value
* allowed object is
* {@link Price3 }
*
*/
public FinancialInstrumentAttributes78 setConvsPric(Price3 value) {
this.convsPric = value;
return this;
}
/**
* Gets the value of the strkPric property.
*
* @return
* possible object is
* {@link Price3 }
*
*/
public Price3 getStrkPric() {
return strkPric;
}
/**
* Sets the value of the strkPric property.
*
* @param value
* allowed object is
* {@link Price3 }
*
*/
public FinancialInstrumentAttributes78 setStrkPric(Price3 value) {
this.strkPric = value;
return this;
}
/**
* Gets the value of the minNmnlQty property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity15Choice }
*
*/
public FinancialInstrumentQuantity15Choice getMinNmnlQty() {
return minNmnlQty;
}
/**
* Sets the value of the minNmnlQty property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity15Choice }
*
*/
public FinancialInstrumentAttributes78 setMinNmnlQty(FinancialInstrumentQuantity15Choice value) {
this.minNmnlQty = value;
return this;
}
/**
* Gets the value of the ctrctSz property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity15Choice }
*
*/
public FinancialInstrumentQuantity15Choice getCtrctSz() {
return ctrctSz;
}
/**
* Sets the value of the ctrctSz property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity15Choice }
*
*/
public FinancialInstrumentAttributes78 setCtrctSz(FinancialInstrumentQuantity15Choice value) {
this.ctrctSz = value;
return this;
}
/**
* Gets the value of the undrlygFinInstrmId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the undrlygFinInstrmId property.
*
*
* For example, to add a new item, do as follows:
*
* getUndrlygFinInstrmId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SecurityIdentification20 }
*
*
* @return
* The value of the undrlygFinInstrmId property.
*/
public List getUndrlygFinInstrmId() {
if (undrlygFinInstrmId == null) {
undrlygFinInstrmId = new ArrayList<>();
}
return this.undrlygFinInstrmId;
}
/**
* Gets the value of the finInstrmAttrAddtlDtls property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFinInstrmAttrAddtlDtls() {
return finInstrmAttrAddtlDtls;
}
/**
* Sets the value of the finInstrmAttrAddtlDtls property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public FinancialInstrumentAttributes78 setFinInstrmAttrAddtlDtls(String value) {
this.finInstrmAttrAddtlDtls = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the undrlygFinInstrmId list.
* @see #getUndrlygFinInstrmId()
*
*/
public FinancialInstrumentAttributes78 addUndrlygFinInstrmId(SecurityIdentification20 undrlygFinInstrmId) {
getUndrlygFinInstrmId().add(undrlygFinInstrmId);
return this;
}
}