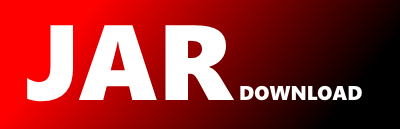
com.prowidesoftware.swift.model.mx.dic.Fund1 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Information about an investment fund.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Fund1", propOrder = {
"nm",
"lglNttyIdr",
"id",
"ccy",
"tradDtTm",
"prvsTradDtTm",
"estmtdTtlNAV",
"prvsTtlNAV",
"estmtdTtlUnitsNb",
"prvsTtlUnitsNb",
"estmtdPctgOfFndTtlNAV",
"estmtdCshInFcstDtls",
"estmtdCshOutFcstDtls",
"estmtdNetCshFcstDtls"
})
public class Fund1 {
@XmlElement(name = "Nm")
protected String nm;
@XmlElement(name = "LglNttyIdr")
protected String lglNttyIdr;
@XmlElement(name = "Id")
protected OtherIdentification4 id;
@XmlElement(name = "Ccy")
protected String ccy;
@XmlElement(name = "TradDtTm")
protected DateAndDateTimeChoice tradDtTm;
@XmlElement(name = "PrvsTradDtTm")
protected DateAndDateTimeChoice prvsTradDtTm;
@XmlElement(name = "EstmtdTtlNAV")
protected ActiveOrHistoricCurrencyAndAmount estmtdTtlNAV;
@XmlElement(name = "PrvsTtlNAV")
protected ActiveOrHistoricCurrencyAndAmount prvsTtlNAV;
@XmlElement(name = "EstmtdTtlUnitsNb")
protected FinancialInstrumentQuantity1 estmtdTtlUnitsNb;
@XmlElement(name = "PrvsTtlUnitsNb")
protected FinancialInstrumentQuantity1 prvsTtlUnitsNb;
@XmlElement(name = "EstmtdPctgOfFndTtlNAV")
protected BigDecimal estmtdPctgOfFndTtlNAV;
@XmlElement(name = "EstmtdCshInFcstDtls")
protected List estmtdCshInFcstDtls;
@XmlElement(name = "EstmtdCshOutFcstDtls")
protected List estmtdCshOutFcstDtls;
@XmlElement(name = "EstmtdNetCshFcstDtls")
protected List estmtdNetCshFcstDtls;
/**
* Gets the value of the nm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNm() {
return nm;
}
/**
* Sets the value of the nm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Fund1 setNm(String value) {
this.nm = value;
return this;
}
/**
* Gets the value of the lglNttyIdr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLglNttyIdr() {
return lglNttyIdr;
}
/**
* Sets the value of the lglNttyIdr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Fund1 setLglNttyIdr(String value) {
this.lglNttyIdr = value;
return this;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link OtherIdentification4 }
*
*/
public OtherIdentification4 getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link OtherIdentification4 }
*
*/
public Fund1 setId(OtherIdentification4 value) {
this.id = value;
return this;
}
/**
* Gets the value of the ccy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCcy() {
return ccy;
}
/**
* Sets the value of the ccy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public Fund1 setCcy(String value) {
this.ccy = value;
return this;
}
/**
* Gets the value of the tradDtTm property.
*
* @return
* possible object is
* {@link DateAndDateTimeChoice }
*
*/
public DateAndDateTimeChoice getTradDtTm() {
return tradDtTm;
}
/**
* Sets the value of the tradDtTm property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeChoice }
*
*/
public Fund1 setTradDtTm(DateAndDateTimeChoice value) {
this.tradDtTm = value;
return this;
}
/**
* Gets the value of the prvsTradDtTm property.
*
* @return
* possible object is
* {@link DateAndDateTimeChoice }
*
*/
public DateAndDateTimeChoice getPrvsTradDtTm() {
return prvsTradDtTm;
}
/**
* Sets the value of the prvsTradDtTm property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeChoice }
*
*/
public Fund1 setPrvsTradDtTm(DateAndDateTimeChoice value) {
this.prvsTradDtTm = value;
return this;
}
/**
* Gets the value of the estmtdTtlNAV property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getEstmtdTtlNAV() {
return estmtdTtlNAV;
}
/**
* Sets the value of the estmtdTtlNAV property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public Fund1 setEstmtdTtlNAV(ActiveOrHistoricCurrencyAndAmount value) {
this.estmtdTtlNAV = value;
return this;
}
/**
* Gets the value of the prvsTtlNAV property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getPrvsTtlNAV() {
return prvsTtlNAV;
}
/**
* Sets the value of the prvsTtlNAV property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public Fund1 setPrvsTtlNAV(ActiveOrHistoricCurrencyAndAmount value) {
this.prvsTtlNAV = value;
return this;
}
/**
* Gets the value of the estmtdTtlUnitsNb property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public FinancialInstrumentQuantity1 getEstmtdTtlUnitsNb() {
return estmtdTtlUnitsNb;
}
/**
* Sets the value of the estmtdTtlUnitsNb property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public Fund1 setEstmtdTtlUnitsNb(FinancialInstrumentQuantity1 value) {
this.estmtdTtlUnitsNb = value;
return this;
}
/**
* Gets the value of the prvsTtlUnitsNb property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public FinancialInstrumentQuantity1 getPrvsTtlUnitsNb() {
return prvsTtlUnitsNb;
}
/**
* Sets the value of the prvsTtlUnitsNb property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public Fund1 setPrvsTtlUnitsNb(FinancialInstrumentQuantity1 value) {
this.prvsTtlUnitsNb = value;
return this;
}
/**
* Gets the value of the estmtdPctgOfFndTtlNAV property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getEstmtdPctgOfFndTtlNAV() {
return estmtdPctgOfFndTtlNAV;
}
/**
* Sets the value of the estmtdPctgOfFndTtlNAV property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public Fund1 setEstmtdPctgOfFndTtlNAV(BigDecimal value) {
this.estmtdPctgOfFndTtlNAV = value;
return this;
}
/**
* Gets the value of the estmtdCshInFcstDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the estmtdCshInFcstDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getEstmtdCshInFcstDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CashInOutForecast7 }
*
*
* @return
* The value of the estmtdCshInFcstDtls property.
*/
public List getEstmtdCshInFcstDtls() {
if (estmtdCshInFcstDtls == null) {
estmtdCshInFcstDtls = new ArrayList<>();
}
return this.estmtdCshInFcstDtls;
}
/**
* Gets the value of the estmtdCshOutFcstDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the estmtdCshOutFcstDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getEstmtdCshOutFcstDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CashInOutForecast7 }
*
*
* @return
* The value of the estmtdCshOutFcstDtls property.
*/
public List getEstmtdCshOutFcstDtls() {
if (estmtdCshOutFcstDtls == null) {
estmtdCshOutFcstDtls = new ArrayList<>();
}
return this.estmtdCshOutFcstDtls;
}
/**
* Gets the value of the estmtdNetCshFcstDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the estmtdNetCshFcstDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getEstmtdNetCshFcstDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NetCashForecast5 }
*
*
* @return
* The value of the estmtdNetCshFcstDtls property.
*/
public List getEstmtdNetCshFcstDtls() {
if (estmtdNetCshFcstDtls == null) {
estmtdNetCshFcstDtls = new ArrayList<>();
}
return this.estmtdNetCshFcstDtls;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the estmtdCshInFcstDtls list.
* @see #getEstmtdCshInFcstDtls()
*
*/
public Fund1 addEstmtdCshInFcstDtls(CashInOutForecast7 estmtdCshInFcstDtls) {
getEstmtdCshInFcstDtls().add(estmtdCshInFcstDtls);
return this;
}
/**
* Adds a new item to the estmtdCshOutFcstDtls list.
* @see #getEstmtdCshOutFcstDtls()
*
*/
public Fund1 addEstmtdCshOutFcstDtls(CashInOutForecast7 estmtdCshOutFcstDtls) {
getEstmtdCshOutFcstDtls().add(estmtdCshOutFcstDtls);
return this;
}
/**
* Adds a new item to the estmtdNetCshFcstDtls list.
* @see #getEstmtdNetCshFcstDtls()
*
*/
public Fund1 addEstmtdNetCshFcstDtls(NetCashForecast5 estmtdNetCshFcstDtls) {
getEstmtdNetCshFcstDtls().add(estmtdNetCshFcstDtls);
return this;
}
}