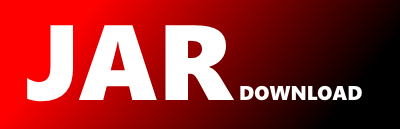
com.prowidesoftware.swift.model.mx.dic.FundCashForecast2 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Cash movements from or to a fund as a result of investment funds transactions, eg, subscriptions or redemptions.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FundCashForecast2", propOrder = {
"tradDtTm",
"prvsTradDtTm",
"finInstrmDtls",
"ttlNAV",
"prvsTtlNAV",
"ttlUnitsNb",
"prvsTtlUnitsNb",
"ttlNAVChngRate",
"invstmtCcy",
"xcptnlNetCshFlowInd",
"srtgCritDtls",
"netCshFcstDtls"
})
public class FundCashForecast2 {
@XmlElement(name = "TradDtTm", required = true)
protected DateAndDateTimeChoice tradDtTm;
@XmlElement(name = "PrvsTradDtTm")
protected DateAndDateTimeChoice prvsTradDtTm;
@XmlElement(name = "FinInstrmDtls", required = true)
protected FinancialInstrument5 finInstrmDtls;
@XmlElement(name = "TtlNAV")
protected ActiveOrHistoricCurrencyAndAmount ttlNAV;
@XmlElement(name = "PrvsTtlNAV")
protected ActiveOrHistoricCurrencyAndAmount prvsTtlNAV;
@XmlElement(name = "TtlUnitsNb")
protected FinancialInstrumentQuantity1 ttlUnitsNb;
@XmlElement(name = "PrvsTtlUnitsNb")
protected FinancialInstrumentQuantity1 prvsTtlUnitsNb;
@XmlElement(name = "TtlNAVChngRate")
protected BigDecimal ttlNAVChngRate;
@XmlElement(name = "InvstmtCcy")
protected List invstmtCcy;
@XmlElement(name = "XcptnlNetCshFlowInd")
protected boolean xcptnlNetCshFlowInd;
@XmlElement(name = "SrtgCritDtls", required = true)
protected List srtgCritDtls;
@XmlElement(name = "NetCshFcstDtls")
protected List netCshFcstDtls;
/**
* Gets the value of the tradDtTm property.
*
* @return
* possible object is
* {@link DateAndDateTimeChoice }
*
*/
public DateAndDateTimeChoice getTradDtTm() {
return tradDtTm;
}
/**
* Sets the value of the tradDtTm property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeChoice }
*
*/
public FundCashForecast2 setTradDtTm(DateAndDateTimeChoice value) {
this.tradDtTm = value;
return this;
}
/**
* Gets the value of the prvsTradDtTm property.
*
* @return
* possible object is
* {@link DateAndDateTimeChoice }
*
*/
public DateAndDateTimeChoice getPrvsTradDtTm() {
return prvsTradDtTm;
}
/**
* Sets the value of the prvsTradDtTm property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeChoice }
*
*/
public FundCashForecast2 setPrvsTradDtTm(DateAndDateTimeChoice value) {
this.prvsTradDtTm = value;
return this;
}
/**
* Gets the value of the finInstrmDtls property.
*
* @return
* possible object is
* {@link FinancialInstrument5 }
*
*/
public FinancialInstrument5 getFinInstrmDtls() {
return finInstrmDtls;
}
/**
* Sets the value of the finInstrmDtls property.
*
* @param value
* allowed object is
* {@link FinancialInstrument5 }
*
*/
public FundCashForecast2 setFinInstrmDtls(FinancialInstrument5 value) {
this.finInstrmDtls = value;
return this;
}
/**
* Gets the value of the ttlNAV property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getTtlNAV() {
return ttlNAV;
}
/**
* Sets the value of the ttlNAV property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public FundCashForecast2 setTtlNAV(ActiveOrHistoricCurrencyAndAmount value) {
this.ttlNAV = value;
return this;
}
/**
* Gets the value of the prvsTtlNAV property.
*
* @return
* possible object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public ActiveOrHistoricCurrencyAndAmount getPrvsTtlNAV() {
return prvsTtlNAV;
}
/**
* Sets the value of the prvsTtlNAV property.
*
* @param value
* allowed object is
* {@link ActiveOrHistoricCurrencyAndAmount }
*
*/
public FundCashForecast2 setPrvsTtlNAV(ActiveOrHistoricCurrencyAndAmount value) {
this.prvsTtlNAV = value;
return this;
}
/**
* Gets the value of the ttlUnitsNb property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public FinancialInstrumentQuantity1 getTtlUnitsNb() {
return ttlUnitsNb;
}
/**
* Sets the value of the ttlUnitsNb property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public FundCashForecast2 setTtlUnitsNb(FinancialInstrumentQuantity1 value) {
this.ttlUnitsNb = value;
return this;
}
/**
* Gets the value of the prvsTtlUnitsNb property.
*
* @return
* possible object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public FinancialInstrumentQuantity1 getPrvsTtlUnitsNb() {
return prvsTtlUnitsNb;
}
/**
* Sets the value of the prvsTtlUnitsNb property.
*
* @param value
* allowed object is
* {@link FinancialInstrumentQuantity1 }
*
*/
public FundCashForecast2 setPrvsTtlUnitsNb(FinancialInstrumentQuantity1 value) {
this.prvsTtlUnitsNb = value;
return this;
}
/**
* Gets the value of the ttlNAVChngRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTtlNAVChngRate() {
return ttlNAVChngRate;
}
/**
* Sets the value of the ttlNAVChngRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public FundCashForecast2 setTtlNAVChngRate(BigDecimal value) {
this.ttlNAVChngRate = value;
return this;
}
/**
* Gets the value of the invstmtCcy property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the invstmtCcy property.
*
*
* For example, to add a new item, do as follows:
*
* getInvstmtCcy().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the invstmtCcy property.
*/
public List getInvstmtCcy() {
if (invstmtCcy == null) {
invstmtCcy = new ArrayList<>();
}
return this.invstmtCcy;
}
/**
* Gets the value of the xcptnlNetCshFlowInd property.
*
*/
public boolean isXcptnlNetCshFlowInd() {
return xcptnlNetCshFlowInd;
}
/**
* Sets the value of the xcptnlNetCshFlowInd property.
*
*/
public FundCashForecast2 setXcptnlNetCshFlowInd(boolean value) {
this.xcptnlNetCshFlowInd = value;
return this;
}
/**
* Gets the value of the srtgCritDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the srtgCritDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getSrtgCritDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CashSortingCriterion2 }
*
*
* @return
* The value of the srtgCritDtls property.
*/
public List getSrtgCritDtls() {
if (srtgCritDtls == null) {
srtgCritDtls = new ArrayList<>();
}
return this.srtgCritDtls;
}
/**
* Gets the value of the netCshFcstDtls property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the netCshFcstDtls property.
*
*
* For example, to add a new item, do as follows:
*
* getNetCshFcstDtls().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NetCashForecast1 }
*
*
* @return
* The value of the netCshFcstDtls property.
*/
public List getNetCshFcstDtls() {
if (netCshFcstDtls == null) {
netCshFcstDtls = new ArrayList<>();
}
return this.netCshFcstDtls;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the invstmtCcy list.
* @see #getInvstmtCcy()
*
*/
public FundCashForecast2 addInvstmtCcy(String invstmtCcy) {
getInvstmtCcy().add(invstmtCcy);
return this;
}
/**
* Adds a new item to the srtgCritDtls list.
* @see #getSrtgCritDtls()
*
*/
public FundCashForecast2 addSrtgCritDtls(CashSortingCriterion2 srtgCritDtls) {
getSrtgCritDtls().add(srtgCritDtls);
return this;
}
/**
* Adds a new item to the netCshFcstDtls list.
* @see #getNetCshFcstDtls()
*
*/
public FundCashForecast2 addNetCshFcstDtls(NetCashForecast1 netCshFcstDtls) {
getNetCshFcstDtls().add(netCshFcstDtls);
return this;
}
}