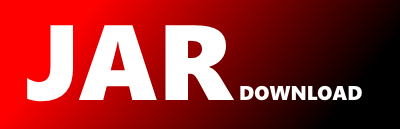
com.prowidesoftware.swift.model.mx.dic.IntraBalanceQueryCriteria11 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Defines the criteria based on which information is included.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "IntraBalanceQueryCriteria11", propOrder = {
"refs",
"sts",
"cshAcct",
"cshAcctOwnr",
"cshAcctSvcr",
"balTp",
"cshSubBalId",
"sttlmAmt",
"sttldAmt",
"sttlmCcy",
"intnddSttlmDt",
"fctvSttlmDt",
"prty",
"msgOrgtr",
"creDtTm"
})
public class IntraBalanceQueryCriteria11 {
@XmlElement(name = "Refs")
protected List refs;
@XmlElement(name = "Sts")
protected IntraBalanceQueryStatus3 sts;
@XmlElement(name = "CshAcct")
protected List cshAcct;
@XmlElement(name = "CshAcctOwnr")
protected List cshAcctOwnr;
@XmlElement(name = "CshAcctSvcr")
protected BranchAndFinancialInstitutionIdentification8 cshAcctSvcr;
@XmlElement(name = "BalTp")
protected List balTp;
@XmlElement(name = "CshSubBalId")
protected List cshSubBalId;
@XmlElement(name = "SttlmAmt")
protected ImpliedCurrencyAmountRange1Choice sttlmAmt;
@XmlElement(name = "SttldAmt")
protected ImpliedCurrencyAmountRange1Choice sttldAmt;
@XmlElement(name = "SttlmCcy")
protected List sttlmCcy;
@XmlElement(name = "IntnddSttlmDt")
protected DateAndDateTimeSearch5Choice intnddSttlmDt;
@XmlElement(name = "FctvSttlmDt")
protected DateAndDateTimeSearch5Choice fctvSttlmDt;
@XmlElement(name = "Prty")
protected List prty;
@XmlElement(name = "MsgOrgtr")
protected List msgOrgtr;
@XmlElement(name = "CreDtTm")
protected DateAndDateTimeSearch5Choice creDtTm;
/**
* Gets the value of the refs property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the refs property.
*
*
* For example, to add a new item, do as follows:
*
* getRefs().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link References36Choice }
*
*
* @return
* The value of the refs property.
*/
public List getRefs() {
if (refs == null) {
refs = new ArrayList<>();
}
return this.refs;
}
/**
* Gets the value of the sts property.
*
* @return
* possible object is
* {@link IntraBalanceQueryStatus3 }
*
*/
public IntraBalanceQueryStatus3 getSts() {
return sts;
}
/**
* Sets the value of the sts property.
*
* @param value
* allowed object is
* {@link IntraBalanceQueryStatus3 }
*
*/
public IntraBalanceQueryCriteria11 setSts(IntraBalanceQueryStatus3 value) {
this.sts = value;
return this;
}
/**
* Gets the value of the cshAcct property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cshAcct property.
*
*
* For example, to add a new item, do as follows:
*
* getCshAcct().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AccountIdentificationSearchCriteria2Choice }
*
*
* @return
* The value of the cshAcct property.
*/
public List getCshAcct() {
if (cshAcct == null) {
cshAcct = new ArrayList<>();
}
return this.cshAcct;
}
/**
* Gets the value of the cshAcctOwnr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cshAcctOwnr property.
*
*
* For example, to add a new item, do as follows:
*
* getCshAcctOwnr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SystemPartyIdentification8 }
*
*
* @return
* The value of the cshAcctOwnr property.
*/
public List getCshAcctOwnr() {
if (cshAcctOwnr == null) {
cshAcctOwnr = new ArrayList<>();
}
return this.cshAcctOwnr;
}
/**
* Gets the value of the cshAcctSvcr property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public BranchAndFinancialInstitutionIdentification8 getCshAcctSvcr() {
return cshAcctSvcr;
}
/**
* Sets the value of the cshAcctSvcr property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification8 }
*
*/
public IntraBalanceQueryCriteria11 setCshAcctSvcr(BranchAndFinancialInstitutionIdentification8 value) {
this.cshAcctSvcr = value;
return this;
}
/**
* Gets the value of the balTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the balTp property.
*
*
* For example, to add a new item, do as follows:
*
* getBalTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IntraBalanceType3 }
*
*
* @return
* The value of the balTp property.
*/
public List getBalTp() {
if (balTp == null) {
balTp = new ArrayList<>();
}
return this.balTp;
}
/**
* Gets the value of the cshSubBalId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cshSubBalId property.
*
*
* For example, to add a new item, do as follows:
*
* getCshSubBalId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link GenericIdentification37 }
*
*
* @return
* The value of the cshSubBalId property.
*/
public List getCshSubBalId() {
if (cshSubBalId == null) {
cshSubBalId = new ArrayList<>();
}
return this.cshSubBalId;
}
/**
* Gets the value of the sttlmAmt property.
*
* @return
* possible object is
* {@link ImpliedCurrencyAmountRange1Choice }
*
*/
public ImpliedCurrencyAmountRange1Choice getSttlmAmt() {
return sttlmAmt;
}
/**
* Sets the value of the sttlmAmt property.
*
* @param value
* allowed object is
* {@link ImpliedCurrencyAmountRange1Choice }
*
*/
public IntraBalanceQueryCriteria11 setSttlmAmt(ImpliedCurrencyAmountRange1Choice value) {
this.sttlmAmt = value;
return this;
}
/**
* Gets the value of the sttldAmt property.
*
* @return
* possible object is
* {@link ImpliedCurrencyAmountRange1Choice }
*
*/
public ImpliedCurrencyAmountRange1Choice getSttldAmt() {
return sttldAmt;
}
/**
* Sets the value of the sttldAmt property.
*
* @param value
* allowed object is
* {@link ImpliedCurrencyAmountRange1Choice }
*
*/
public IntraBalanceQueryCriteria11 setSttldAmt(ImpliedCurrencyAmountRange1Choice value) {
this.sttldAmt = value;
return this;
}
/**
* Gets the value of the sttlmCcy property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the sttlmCcy property.
*
*
* For example, to add a new item, do as follows:
*
* getSttlmCcy().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the sttlmCcy property.
*/
public List getSttlmCcy() {
if (sttlmCcy == null) {
sttlmCcy = new ArrayList<>();
}
return this.sttlmCcy;
}
/**
* Gets the value of the intnddSttlmDt property.
*
* @return
* possible object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public DateAndDateTimeSearch5Choice getIntnddSttlmDt() {
return intnddSttlmDt;
}
/**
* Sets the value of the intnddSttlmDt property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public IntraBalanceQueryCriteria11 setIntnddSttlmDt(DateAndDateTimeSearch5Choice value) {
this.intnddSttlmDt = value;
return this;
}
/**
* Gets the value of the fctvSttlmDt property.
*
* @return
* possible object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public DateAndDateTimeSearch5Choice getFctvSttlmDt() {
return fctvSttlmDt;
}
/**
* Sets the value of the fctvSttlmDt property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public IntraBalanceQueryCriteria11 setFctvSttlmDt(DateAndDateTimeSearch5Choice value) {
this.fctvSttlmDt = value;
return this;
}
/**
* Gets the value of the prty property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the prty property.
*
*
* For example, to add a new item, do as follows:
*
* getPrty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PriorityNumeric4Choice }
*
*
* @return
* The value of the prty property.
*/
public List getPrty() {
if (prty == null) {
prty = new ArrayList<>();
}
return this.prty;
}
/**
* Gets the value of the msgOrgtr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the msgOrgtr property.
*
*
* For example, to add a new item, do as follows:
*
* getMsgOrgtr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SystemPartyIdentification8 }
*
*
* @return
* The value of the msgOrgtr property.
*/
public List getMsgOrgtr() {
if (msgOrgtr == null) {
msgOrgtr = new ArrayList<>();
}
return this.msgOrgtr;
}
/**
* Gets the value of the creDtTm property.
*
* @return
* possible object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public DateAndDateTimeSearch5Choice getCreDtTm() {
return creDtTm;
}
/**
* Sets the value of the creDtTm property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public IntraBalanceQueryCriteria11 setCreDtTm(DateAndDateTimeSearch5Choice value) {
this.creDtTm = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the refs list.
* @see #getRefs()
*
*/
public IntraBalanceQueryCriteria11 addRefs(References36Choice refs) {
getRefs().add(refs);
return this;
}
/**
* Adds a new item to the cshAcct list.
* @see #getCshAcct()
*
*/
public IntraBalanceQueryCriteria11 addCshAcct(AccountIdentificationSearchCriteria2Choice cshAcct) {
getCshAcct().add(cshAcct);
return this;
}
/**
* Adds a new item to the cshAcctOwnr list.
* @see #getCshAcctOwnr()
*
*/
public IntraBalanceQueryCriteria11 addCshAcctOwnr(SystemPartyIdentification8 cshAcctOwnr) {
getCshAcctOwnr().add(cshAcctOwnr);
return this;
}
/**
* Adds a new item to the balTp list.
* @see #getBalTp()
*
*/
public IntraBalanceQueryCriteria11 addBalTp(IntraBalanceType3 balTp) {
getBalTp().add(balTp);
return this;
}
/**
* Adds a new item to the cshSubBalId list.
* @see #getCshSubBalId()
*
*/
public IntraBalanceQueryCriteria11 addCshSubBalId(GenericIdentification37 cshSubBalId) {
getCshSubBalId().add(cshSubBalId);
return this;
}
/**
* Adds a new item to the sttlmCcy list.
* @see #getSttlmCcy()
*
*/
public IntraBalanceQueryCriteria11 addSttlmCcy(String sttlmCcy) {
getSttlmCcy().add(sttlmCcy);
return this;
}
/**
* Adds a new item to the prty list.
* @see #getPrty()
*
*/
public IntraBalanceQueryCriteria11 addPrty(PriorityNumeric4Choice prty) {
getPrty().add(prty);
return this;
}
/**
* Adds a new item to the msgOrgtr list.
* @see #getMsgOrgtr()
*
*/
public IntraBalanceQueryCriteria11 addMsgOrgtr(SystemPartyIdentification8 msgOrgtr) {
getMsgOrgtr().add(msgOrgtr);
return this;
}
}