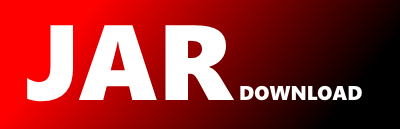
com.prowidesoftware.swift.model.mx.dic.IntraBalanceQueryCriteria7 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Defines the criteria based on which information is included.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "IntraBalanceQueryCriteria7", propOrder = {
"cxlReqId",
"prcgSts",
"cshAcct",
"cshAcctOwnr",
"cshAcctSvcr",
"msgOrgtr",
"creDtTm"
})
public class IntraBalanceQueryCriteria7 {
@XmlElement(name = "CxlReqId")
protected List cxlReqId;
@XmlElement(name = "PrcgSts")
protected List prcgSts;
@XmlElement(name = "CshAcct")
protected List cshAcct;
@XmlElement(name = "CshAcctOwnr")
protected List cshAcctOwnr;
@XmlElement(name = "CshAcctSvcr")
protected BranchAndFinancialInstitutionIdentification6 cshAcctSvcr;
@XmlElement(name = "MsgOrgtr")
protected List msgOrgtr;
@XmlElement(name = "CreDtTm")
protected DateAndDateTimeSearch5Choice creDtTm;
/**
* Gets the value of the cxlReqId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cxlReqId property.
*
*
* For example, to add a new item, do as follows:
*
* getCxlReqId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the cxlReqId property.
*/
public List getCxlReqId() {
if (cxlReqId == null) {
cxlReqId = new ArrayList<>();
}
return this.cxlReqId;
}
/**
* Gets the value of the prcgSts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the prcgSts property.
*
*
* For example, to add a new item, do as follows:
*
* getPrcgSts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CancellationProcessingStatus9Choice }
*
*
* @return
* The value of the prcgSts property.
*/
public List getPrcgSts() {
if (prcgSts == null) {
prcgSts = new ArrayList<>();
}
return this.prcgSts;
}
/**
* Gets the value of the cshAcct property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cshAcct property.
*
*
* For example, to add a new item, do as follows:
*
* getCshAcct().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AccountIdentificationSearchCriteria2Choice }
*
*
* @return
* The value of the cshAcct property.
*/
public List getCshAcct() {
if (cshAcct == null) {
cshAcct = new ArrayList<>();
}
return this.cshAcct;
}
/**
* Gets the value of the cshAcctOwnr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the cshAcctOwnr property.
*
*
* For example, to add a new item, do as follows:
*
* getCshAcctOwnr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SystemPartyIdentification8 }
*
*
* @return
* The value of the cshAcctOwnr property.
*/
public List getCshAcctOwnr() {
if (cshAcctOwnr == null) {
cshAcctOwnr = new ArrayList<>();
}
return this.cshAcctOwnr;
}
/**
* Gets the value of the cshAcctSvcr property.
*
* @return
* possible object is
* {@link BranchAndFinancialInstitutionIdentification6 }
*
*/
public BranchAndFinancialInstitutionIdentification6 getCshAcctSvcr() {
return cshAcctSvcr;
}
/**
* Sets the value of the cshAcctSvcr property.
*
* @param value
* allowed object is
* {@link BranchAndFinancialInstitutionIdentification6 }
*
*/
public IntraBalanceQueryCriteria7 setCshAcctSvcr(BranchAndFinancialInstitutionIdentification6 value) {
this.cshAcctSvcr = value;
return this;
}
/**
* Gets the value of the msgOrgtr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the msgOrgtr property.
*
*
* For example, to add a new item, do as follows:
*
* getMsgOrgtr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SystemPartyIdentification8 }
*
*
* @return
* The value of the msgOrgtr property.
*/
public List getMsgOrgtr() {
if (msgOrgtr == null) {
msgOrgtr = new ArrayList<>();
}
return this.msgOrgtr;
}
/**
* Gets the value of the creDtTm property.
*
* @return
* possible object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public DateAndDateTimeSearch5Choice getCreDtTm() {
return creDtTm;
}
/**
* Sets the value of the creDtTm property.
*
* @param value
* allowed object is
* {@link DateAndDateTimeSearch5Choice }
*
*/
public IntraBalanceQueryCriteria7 setCreDtTm(DateAndDateTimeSearch5Choice value) {
this.creDtTm = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the cxlReqId list.
* @see #getCxlReqId()
*
*/
public IntraBalanceQueryCriteria7 addCxlReqId(String cxlReqId) {
getCxlReqId().add(cxlReqId);
return this;
}
/**
* Adds a new item to the prcgSts list.
* @see #getPrcgSts()
*
*/
public IntraBalanceQueryCriteria7 addPrcgSts(CancellationProcessingStatus9Choice prcgSts) {
getPrcgSts().add(prcgSts);
return this;
}
/**
* Adds a new item to the cshAcct list.
* @see #getCshAcct()
*
*/
public IntraBalanceQueryCriteria7 addCshAcct(AccountIdentificationSearchCriteria2Choice cshAcct) {
getCshAcct().add(cshAcct);
return this;
}
/**
* Adds a new item to the cshAcctOwnr list.
* @see #getCshAcctOwnr()
*
*/
public IntraBalanceQueryCriteria7 addCshAcctOwnr(SystemPartyIdentification8 cshAcctOwnr) {
getCshAcctOwnr().add(cshAcctOwnr);
return this;
}
/**
* Adds a new item to the msgOrgtr list.
* @see #getMsgOrgtr()
*
*/
public IntraBalanceQueryCriteria7 addMsgOrgtr(SystemPartyIdentification8 msgOrgtr) {
getMsgOrgtr().add(msgOrgtr);
return this;
}
}