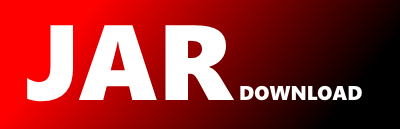
com.prowidesoftware.swift.model.mx.dic.InvestmentAccountOwnershipInformation14 Maven / Gradle / Ivy
Show all versions of pw-iso20022 Show documentation
package com.prowidesoftware.swift.model.mx.dic;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import com.prowidesoftware.swift.model.mx.adapters.IsoDateAdapter;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* Characteristics of the ownership of a securities account.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "InvestmentAccountOwnershipInformation14", propOrder = {
"pty",
"mnyLndrgChck",
"invstrPrflVldtn",
"ownrshBnfcryRate",
"clntId",
"fsclXmptn",
"sgntryRghtInd",
"miFIDClssfctn",
"ntfctn",
"fatcaFormTp",
"fatcaSts",
"fatcaRptgDt",
"crsFormTp",
"crsSts",
"crsRptgDt",
"othrId",
"taxXmptn",
"taxRptg",
"lang",
"mailTp",
"ctryAndResdtlSts",
"mntryWlth",
"eqtyVal",
"workgCptl",
"cpnyLk",
"elctrncMlngSvcRef",
"pmryComAdr",
"scndryComAdr",
"addtlRgltryInf",
"acctgSts",
"addtlInf",
"ctrlgPty"
})
public class InvestmentAccountOwnershipInformation14 {
@XmlElement(name = "Pty", required = true)
protected Party32Choice pty;
@XmlElement(name = "MnyLndrgChck")
protected MoneyLaunderingCheck1Choice mnyLndrgChck;
@XmlElement(name = "InvstrPrflVldtn")
protected List invstrPrflVldtn;
@XmlElement(name = "OwnrshBnfcryRate")
protected OwnershipBeneficiaryRate1 ownrshBnfcryRate;
@XmlElement(name = "ClntId")
protected String clntId;
@XmlElement(name = "FsclXmptn")
protected Boolean fsclXmptn;
@XmlElement(name = "SgntryRghtInd")
protected Boolean sgntryRghtInd;
@XmlElement(name = "MiFIDClssfctn")
protected MiFIDClassification1 miFIDClssfctn;
@XmlElement(name = "Ntfctn")
protected List ntfctn;
@XmlElement(name = "FATCAFormTp")
protected List fatcaFormTp;
@XmlElement(name = "FATCASts")
protected List fatcaSts;
@XmlElement(name = "FATCARptgDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate fatcaRptgDt;
@XmlElement(name = "CRSFormTp")
protected List crsFormTp;
@XmlElement(name = "CRSSts")
protected List crsSts;
@XmlElement(name = "CRSRptgDt", type = String.class)
@XmlJavaTypeAdapter(IsoDateAdapter.class)
@XmlSchemaType(name = "date")
protected LocalDate crsRptgDt;
@XmlElement(name = "OthrId")
protected List othrId;
@XmlElement(name = "TaxXmptn")
protected TaxExemptionReason2Choice taxXmptn;
@XmlElement(name = "TaxRptg")
protected List taxRptg;
@XmlElement(name = "Lang")
protected String lang;
@XmlElement(name = "MailTp")
protected MailType1Choice mailTp;
@XmlElement(name = "CtryAndResdtlSts")
protected CountryAndResidentialStatusType2 ctryAndResdtlSts;
@XmlElement(name = "MntryWlth")
protected DateAndAmount1 mntryWlth;
@XmlElement(name = "EqtyVal")
protected DateAndAmount1 eqtyVal;
@XmlElement(name = "WorkgCptl")
protected DateAndAmount1 workgCptl;
@XmlElement(name = "CpnyLk")
protected CompanyLink1Choice cpnyLk;
@XmlElement(name = "ElctrncMlngSvcRef")
protected String elctrncMlngSvcRef;
@XmlElement(name = "PmryComAdr")
protected List pmryComAdr;
@XmlElement(name = "ScndryComAdr")
protected List scndryComAdr;
@XmlElement(name = "AddtlRgltryInf")
protected RegulatoryInformation1 addtlRgltryInf;
@XmlElement(name = "AcctgSts")
protected AccountingStatus1Choice acctgSts;
@XmlElement(name = "AddtlInf")
protected List addtlInf;
@XmlElement(name = "CtrlgPty")
protected Boolean ctrlgPty;
/**
* Gets the value of the pty property.
*
* @return
* possible object is
* {@link Party32Choice }
*
*/
public Party32Choice getPty() {
return pty;
}
/**
* Sets the value of the pty property.
*
* @param value
* allowed object is
* {@link Party32Choice }
*
*/
public InvestmentAccountOwnershipInformation14 setPty(Party32Choice value) {
this.pty = value;
return this;
}
/**
* Gets the value of the mnyLndrgChck property.
*
* @return
* possible object is
* {@link MoneyLaunderingCheck1Choice }
*
*/
public MoneyLaunderingCheck1Choice getMnyLndrgChck() {
return mnyLndrgChck;
}
/**
* Sets the value of the mnyLndrgChck property.
*
* @param value
* allowed object is
* {@link MoneyLaunderingCheck1Choice }
*
*/
public InvestmentAccountOwnershipInformation14 setMnyLndrgChck(MoneyLaunderingCheck1Choice value) {
this.mnyLndrgChck = value;
return this;
}
/**
* Gets the value of the invstrPrflVldtn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the invstrPrflVldtn property.
*
*
* For example, to add a new item, do as follows:
*
* getInvstrPrflVldtn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyProfileInformation5 }
*
*
* @return
* The value of the invstrPrflVldtn property.
*/
public List getInvstrPrflVldtn() {
if (invstrPrflVldtn == null) {
invstrPrflVldtn = new ArrayList<>();
}
return this.invstrPrflVldtn;
}
/**
* Gets the value of the ownrshBnfcryRate property.
*
* @return
* possible object is
* {@link OwnershipBeneficiaryRate1 }
*
*/
public OwnershipBeneficiaryRate1 getOwnrshBnfcryRate() {
return ownrshBnfcryRate;
}
/**
* Sets the value of the ownrshBnfcryRate property.
*
* @param value
* allowed object is
* {@link OwnershipBeneficiaryRate1 }
*
*/
public InvestmentAccountOwnershipInformation14 setOwnrshBnfcryRate(OwnershipBeneficiaryRate1 value) {
this.ownrshBnfcryRate = value;
return this;
}
/**
* Gets the value of the clntId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClntId() {
return clntId;
}
/**
* Sets the value of the clntId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public InvestmentAccountOwnershipInformation14 setClntId(String value) {
this.clntId = value;
return this;
}
/**
* Gets the value of the fsclXmptn property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFsclXmptn() {
return fsclXmptn;
}
/**
* Sets the value of the fsclXmptn property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public InvestmentAccountOwnershipInformation14 setFsclXmptn(Boolean value) {
this.fsclXmptn = value;
return this;
}
/**
* Gets the value of the sgntryRghtInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isSgntryRghtInd() {
return sgntryRghtInd;
}
/**
* Sets the value of the sgntryRghtInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public InvestmentAccountOwnershipInformation14 setSgntryRghtInd(Boolean value) {
this.sgntryRghtInd = value;
return this;
}
/**
* Gets the value of the miFIDClssfctn property.
*
* @return
* possible object is
* {@link MiFIDClassification1 }
*
*/
public MiFIDClassification1 getMiFIDClssfctn() {
return miFIDClssfctn;
}
/**
* Sets the value of the miFIDClssfctn property.
*
* @param value
* allowed object is
* {@link MiFIDClassification1 }
*
*/
public InvestmentAccountOwnershipInformation14 setMiFIDClssfctn(MiFIDClassification1 value) {
this.miFIDClssfctn = value;
return this;
}
/**
* Gets the value of the ntfctn property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the ntfctn property.
*
*
* For example, to add a new item, do as follows:
*
* getNtfctn().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Notification2 }
*
*
* @return
* The value of the ntfctn property.
*/
public List getNtfctn() {
if (ntfctn == null) {
ntfctn = new ArrayList<>();
}
return this.ntfctn;
}
/**
* Gets the value of the fatcaFormTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the fatcaFormTp property.
*
*
* For example, to add a new item, do as follows:
*
* getFATCAFormTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FATCAForm1Choice }
*
*
* @return
* The value of the fatcaFormTp property.
*/
public List getFATCAFormTp() {
if (fatcaFormTp == null) {
fatcaFormTp = new ArrayList<>();
}
return this.fatcaFormTp;
}
/**
* Gets the value of the fatcaSts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the fatcaSts property.
*
*
* For example, to add a new item, do as follows:
*
* getFATCASts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FATCAStatus2 }
*
*
* @return
* The value of the fatcaSts property.
*/
public List getFATCASts() {
if (fatcaSts == null) {
fatcaSts = new ArrayList<>();
}
return this.fatcaSts;
}
/**
* Gets the value of the fatcaRptgDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getFATCARptgDt() {
return fatcaRptgDt;
}
/**
* Sets the value of the fatcaRptgDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public InvestmentAccountOwnershipInformation14 setFATCARptgDt(LocalDate value) {
this.fatcaRptgDt = value;
return this;
}
/**
* Gets the value of the crsFormTp property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the crsFormTp property.
*
*
* For example, to add a new item, do as follows:
*
* getCRSFormTp().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CRSForm1Choice }
*
*
* @return
* The value of the crsFormTp property.
*/
public List getCRSFormTp() {
if (crsFormTp == null) {
crsFormTp = new ArrayList<>();
}
return this.crsFormTp;
}
/**
* Gets the value of the crsSts property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the crsSts property.
*
*
* For example, to add a new item, do as follows:
*
* getCRSSts().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CRSStatus4 }
*
*
* @return
* The value of the crsSts property.
*/
public List getCRSSts() {
if (crsSts == null) {
crsSts = new ArrayList<>();
}
return this.crsSts;
}
/**
* Gets the value of the crsRptgDt property.
*
* @return
* possible object is
* {@link String }
*
*/
public LocalDate getCRSRptgDt() {
return crsRptgDt;
}
/**
* Sets the value of the crsRptgDt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public InvestmentAccountOwnershipInformation14 setCRSRptgDt(LocalDate value) {
this.crsRptgDt = value;
return this;
}
/**
* Gets the value of the othrId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the othrId property.
*
*
* For example, to add a new item, do as follows:
*
* getOthrId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link GenericIdentification82 }
*
*
* @return
* The value of the othrId property.
*/
public List getOthrId() {
if (othrId == null) {
othrId = new ArrayList<>();
}
return this.othrId;
}
/**
* Gets the value of the taxXmptn property.
*
* @return
* possible object is
* {@link TaxExemptionReason2Choice }
*
*/
public TaxExemptionReason2Choice getTaxXmptn() {
return taxXmptn;
}
/**
* Sets the value of the taxXmptn property.
*
* @param value
* allowed object is
* {@link TaxExemptionReason2Choice }
*
*/
public InvestmentAccountOwnershipInformation14 setTaxXmptn(TaxExemptionReason2Choice value) {
this.taxXmptn = value;
return this;
}
/**
* Gets the value of the taxRptg property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the taxRptg property.
*
*
* For example, to add a new item, do as follows:
*
* getTaxRptg().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TaxReporting2 }
*
*
* @return
* The value of the taxRptg property.
*/
public List getTaxRptg() {
if (taxRptg == null) {
taxRptg = new ArrayList<>();
}
return this.taxRptg;
}
/**
* Gets the value of the lang property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLang() {
return lang;
}
/**
* Sets the value of the lang property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public InvestmentAccountOwnershipInformation14 setLang(String value) {
this.lang = value;
return this;
}
/**
* Gets the value of the mailTp property.
*
* @return
* possible object is
* {@link MailType1Choice }
*
*/
public MailType1Choice getMailTp() {
return mailTp;
}
/**
* Sets the value of the mailTp property.
*
* @param value
* allowed object is
* {@link MailType1Choice }
*
*/
public InvestmentAccountOwnershipInformation14 setMailTp(MailType1Choice value) {
this.mailTp = value;
return this;
}
/**
* Gets the value of the ctryAndResdtlSts property.
*
* @return
* possible object is
* {@link CountryAndResidentialStatusType2 }
*
*/
public CountryAndResidentialStatusType2 getCtryAndResdtlSts() {
return ctryAndResdtlSts;
}
/**
* Sets the value of the ctryAndResdtlSts property.
*
* @param value
* allowed object is
* {@link CountryAndResidentialStatusType2 }
*
*/
public InvestmentAccountOwnershipInformation14 setCtryAndResdtlSts(CountryAndResidentialStatusType2 value) {
this.ctryAndResdtlSts = value;
return this;
}
/**
* Gets the value of the mntryWlth property.
*
* @return
* possible object is
* {@link DateAndAmount1 }
*
*/
public DateAndAmount1 getMntryWlth() {
return mntryWlth;
}
/**
* Sets the value of the mntryWlth property.
*
* @param value
* allowed object is
* {@link DateAndAmount1 }
*
*/
public InvestmentAccountOwnershipInformation14 setMntryWlth(DateAndAmount1 value) {
this.mntryWlth = value;
return this;
}
/**
* Gets the value of the eqtyVal property.
*
* @return
* possible object is
* {@link DateAndAmount1 }
*
*/
public DateAndAmount1 getEqtyVal() {
return eqtyVal;
}
/**
* Sets the value of the eqtyVal property.
*
* @param value
* allowed object is
* {@link DateAndAmount1 }
*
*/
public InvestmentAccountOwnershipInformation14 setEqtyVal(DateAndAmount1 value) {
this.eqtyVal = value;
return this;
}
/**
* Gets the value of the workgCptl property.
*
* @return
* possible object is
* {@link DateAndAmount1 }
*
*/
public DateAndAmount1 getWorkgCptl() {
return workgCptl;
}
/**
* Sets the value of the workgCptl property.
*
* @param value
* allowed object is
* {@link DateAndAmount1 }
*
*/
public InvestmentAccountOwnershipInformation14 setWorkgCptl(DateAndAmount1 value) {
this.workgCptl = value;
return this;
}
/**
* Gets the value of the cpnyLk property.
*
* @return
* possible object is
* {@link CompanyLink1Choice }
*
*/
public CompanyLink1Choice getCpnyLk() {
return cpnyLk;
}
/**
* Sets the value of the cpnyLk property.
*
* @param value
* allowed object is
* {@link CompanyLink1Choice }
*
*/
public InvestmentAccountOwnershipInformation14 setCpnyLk(CompanyLink1Choice value) {
this.cpnyLk = value;
return this;
}
/**
* Gets the value of the elctrncMlngSvcRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getElctrncMlngSvcRef() {
return elctrncMlngSvcRef;
}
/**
* Sets the value of the elctrncMlngSvcRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public InvestmentAccountOwnershipInformation14 setElctrncMlngSvcRef(String value) {
this.elctrncMlngSvcRef = value;
return this;
}
/**
* Gets the value of the pmryComAdr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the pmryComAdr property.
*
*
* For example, to add a new item, do as follows:
*
* getPmryComAdr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CommunicationAddress6 }
*
*
* @return
* The value of the pmryComAdr property.
*/
public List getPmryComAdr() {
if (pmryComAdr == null) {
pmryComAdr = new ArrayList<>();
}
return this.pmryComAdr;
}
/**
* Gets the value of the scndryComAdr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the scndryComAdr property.
*
*
* For example, to add a new item, do as follows:
*
* getScndryComAdr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CommunicationAddress6 }
*
*
* @return
* The value of the scndryComAdr property.
*/
public List getScndryComAdr() {
if (scndryComAdr == null) {
scndryComAdr = new ArrayList<>();
}
return this.scndryComAdr;
}
/**
* Gets the value of the addtlRgltryInf property.
*
* @return
* possible object is
* {@link RegulatoryInformation1 }
*
*/
public RegulatoryInformation1 getAddtlRgltryInf() {
return addtlRgltryInf;
}
/**
* Sets the value of the addtlRgltryInf property.
*
* @param value
* allowed object is
* {@link RegulatoryInformation1 }
*
*/
public InvestmentAccountOwnershipInformation14 setAddtlRgltryInf(RegulatoryInformation1 value) {
this.addtlRgltryInf = value;
return this;
}
/**
* Gets the value of the acctgSts property.
*
* @return
* possible object is
* {@link AccountingStatus1Choice }
*
*/
public AccountingStatus1Choice getAcctgSts() {
return acctgSts;
}
/**
* Sets the value of the acctgSts property.
*
* @param value
* allowed object is
* {@link AccountingStatus1Choice }
*
*/
public InvestmentAccountOwnershipInformation14 setAcctgSts(AccountingStatus1Choice value) {
this.acctgSts = value;
return this;
}
/**
* Gets the value of the addtlInf property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the addtlInf property.
*
*
* For example, to add a new item, do as follows:
*
* getAddtlInf().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AdditiononalInformation12 }
*
*
* @return
* The value of the addtlInf property.
*/
public List getAddtlInf() {
if (addtlInf == null) {
addtlInf = new ArrayList<>();
}
return this.addtlInf;
}
/**
* Gets the value of the ctrlgPty property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCtrlgPty() {
return ctrlgPty;
}
/**
* Sets the value of the ctrlgPty property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public InvestmentAccountOwnershipInformation14 setCtrlgPty(Boolean value) {
this.ctrlgPty = value;
return this;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
@Override
public boolean equals(Object that) {
return EqualsBuilder.reflectionEquals(this, that);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
/**
* Adds a new item to the invstrPrflVldtn list.
* @see #getInvstrPrflVldtn()
*
*/
public InvestmentAccountOwnershipInformation14 addInvstrPrflVldtn(PartyProfileInformation5 invstrPrflVldtn) {
getInvstrPrflVldtn().add(invstrPrflVldtn);
return this;
}
/**
* Adds a new item to the ntfctn list.
* @see #getNtfctn()
*
*/
public InvestmentAccountOwnershipInformation14 addNtfctn(Notification2 ntfctn) {
getNtfctn().add(ntfctn);
return this;
}
/**
* Adds a new item to the fATCAFormTp list.
* @see #getFATCAFormTp()
*
*/
public InvestmentAccountOwnershipInformation14 addFATCAFormTp(FATCAForm1Choice fATCAFormTp) {
getFATCAFormTp().add(fATCAFormTp);
return this;
}
/**
* Adds a new item to the fATCASts list.
* @see #getFATCASts()
*
*/
public InvestmentAccountOwnershipInformation14 addFATCASts(FATCAStatus2 fATCASts) {
getFATCASts().add(fATCASts);
return this;
}
/**
* Adds a new item to the cRSFormTp list.
* @see #getCRSFormTp()
*
*/
public InvestmentAccountOwnershipInformation14 addCRSFormTp(CRSForm1Choice cRSFormTp) {
getCRSFormTp().add(cRSFormTp);
return this;
}
/**
* Adds a new item to the cRSSts list.
* @see #getCRSSts()
*
*/
public InvestmentAccountOwnershipInformation14 addCRSSts(CRSStatus4 cRSSts) {
getCRSSts().add(cRSSts);
return this;
}
/**
* Adds a new item to the othrId list.
* @see #getOthrId()
*
*/
public InvestmentAccountOwnershipInformation14 addOthrId(GenericIdentification82 othrId) {
getOthrId().add(othrId);
return this;
}
/**
* Adds a new item to the taxRptg list.
* @see #getTaxRptg()
*
*/
public InvestmentAccountOwnershipInformation14 addTaxRptg(TaxReporting2 taxRptg) {
getTaxRptg().add(taxRptg);
return this;
}
/**
* Adds a new item to the pmryComAdr list.
* @see #getPmryComAdr()
*
*/
public InvestmentAccountOwnershipInformation14 addPmryComAdr(CommunicationAddress6 pmryComAdr) {
getPmryComAdr().add(pmryComAdr);
return this;
}
/**
* Adds a new item to the scndryComAdr list.
* @see #getScndryComAdr()
*
*/
public InvestmentAccountOwnershipInformation14 addScndryComAdr(CommunicationAddress6 scndryComAdr) {
getScndryComAdr().add(scndryComAdr);
return this;
}
/**
* Adds a new item to the addtlInf list.
* @see #getAddtlInf()
*
*/
public InvestmentAccountOwnershipInformation14 addAddtlInf(AdditiononalInformation12 addtlInf) {
getAddtlInf().add(addtlInf);
return this;
}
}